Connectivity analysis¶
Within this section the connectivity analyses
, that is ROI to ROI connectivity
and voxel to voxel connectivity
, are shown, explained and set up in a way that they can be rerun and reproduced. In short, we employed task-based functional connectivity
through NiBetaSeries analysis, to investigate if condition specific information flow within the auditory cortex
provides insights concerning the proposed functional principles
. The analysis is divided into the following sections:
Prerequisites
Auditory cortex atlas creation
Auditory cortex connectivity working model
Event file adaption
Evaluating confounds
NiBetaSeries
model fitting
spatial beta series transformation
Correlation analysis - ROI to ROI
Condition-specific functional connectivity
Comparison of condition-specific functional connectivity
Correlation analysis - voxel to voxel
Diffusion map embedding - Gradients
Diffusion map embedding - component plots
We provide more detailed information and explanations in the respective sections. As mentioned before, we’re working with derivatives
here and thus we can share them, meaning that you can rerun the analyses either by downloading this page as a Jupyter Notebook (via the download button at the top) or interactively via a cloud instance through the amazing mybinder project (the little rocket at the top). We recommend the later to spare installation and conda environment
related problems. One more thing… Please note, that here derivatives
refers to the computed Beta Series
(i.e. condition specific trial-wise beta estimates
) which we provide via the project’s OSF page because their computation is computationally very expensive and would never run via the resources available through binder
. However, we included the code and explainations depicting how this steps were run. The code that will download the required data is included in the respective sections.
As usual, we will start with importing all necessary modules
and functions
.
from os.path import join as opj
from os.path import basename as opb
from os import makedirs, listdir
import numpy as np
from glob import glob
import pandas as pd
import nibabel as nb
from nilearn import image
from nilearn.plotting import plot_roi, plot_matrix
from mne.viz import circular_layout, plot_connectivity_circle
import matplotlib.pyplot as plt
import matplotlib
from matplotlib.colors import ListedColormap
from matplotlib.lines import Line2D
import seaborn as sns
import ptitprince as pt
import plotly.express as px
import plotly.graph_objects as go
from subprocess import Popen, PIPE, STDOUT
%matplotlib inline
Let’s also gather and set some important paths. This includes the path to the general derivatives
directory, as well as analyses
specific ones. In more detail, we of course aim to keep it as BIDS-y as possible and therefore set a path for the Connectivity analyses
here. To keep results and necessary prerequisites
separate (keeping it tidy), we also create a dedicated directory which will include the auditory cortex atlas
, as well as the input data
.
derivatives_path = '/data/mvs/derivatives/'
roi_mask_path ='/data/mvs/derivatives/ROIs_masks/'
prereq_path = '/data/mvs/derivatives/connectivity/prerequisites/'
results_path = '/data/mvs/derivatives/connectivity/'
Prerequisites¶
Atlas creation¶
At first, we need to create an atlas
and corresponding .csv
file to extract ROI
specific beta series
. While the usual approach would be to utilize an existing whole brain atlas, we’re going to rely on our auditory cortex parcellation
introduced in the Auditory cortex working model section given that we are interested in its proposed functional principles. only a subset of regions, comprising the auditory cortex. In more detail, this entails the STP, including HG, PP and PT, and the upper banks of the STG, including aSTG and pSTG. At first, we gather them within a list (please note that we will exclude the auditory cortex masks
):
rois = [roi for roi in glob(opj(roi_mask_path, '*.nii.gz'))
if not 'AC' in opb(roi)]
rois.sort()
rois
['/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-HGleft_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-HGright_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-PPleft_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-PPright_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-PTleft_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-PTright_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-aSTGleft_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-aSTGright_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-pSTGleft_mask.nii.gz',
'/data/mvs/derivatives/ROIs_masks/tpl-MNI152NLin6Sym_res_02_desc-pSTGright_mask.nii.gz']
Great. Now, we will use nilearn’s concat_imgs & nilearn’s math_img to concatenate our ROI images
and create the corresponding atlas:
atlas = image.concat_imgs(rois)
atlas = image.math_img('np.sum(np.arange(1, atlas.shape[-1] + 1)[None, None, None] * atlas, axis=-1)',
atlas=atlas)
atlas.to_filename(opj(prereq_path, 'atlas/tpl-MNI152NLin2009cAsym_res-02_desc-ACatlas.nii.gz'))
So far so good, let’s have a look by overlaying the atlas on the MNI152 template brain
:
sns.set_style('white')
plt.rcParams["font.family"] = "Arial"
ac_atlas_cmap = sns.color_palette('cividis')
fig=plt.figure(num=None, figsize=(12, 6))
plot_roi(opj(prereq_path, 'atlas/tpl-MNI152NLin2009cAsym_res-02_desc-ACatlas.nii.gz'),
draw_cross=False, cmap=ListedColormap(ac_atlas_cmap), cut_coords=[-55, -34, 7], figure=fig)
roi_legend = [Line2D([0], [0], color=ac_atlas_cmap[3], lw=4, label='aSTg'),
Line2D([0], [0], color=ac_atlas_cmap[1], lw=4, label='PP'),
Line2D([0], [0], color=ac_atlas_cmap[0], lw=4, label='HG'),
Line2D([0], [0], color=ac_atlas_cmap[2], lw=4, label='PT'),
Line2D([0], [0], color=ac_atlas_cmap[4], lw=4, label='pSTG')]
plt.legend(handles=roi_legend, bbox_to_anchor=(1.5,0.5), loc="right", title='ROIs')
plt.text(-215, 90, 'Auditory cortex atlas',
fontsize=22)
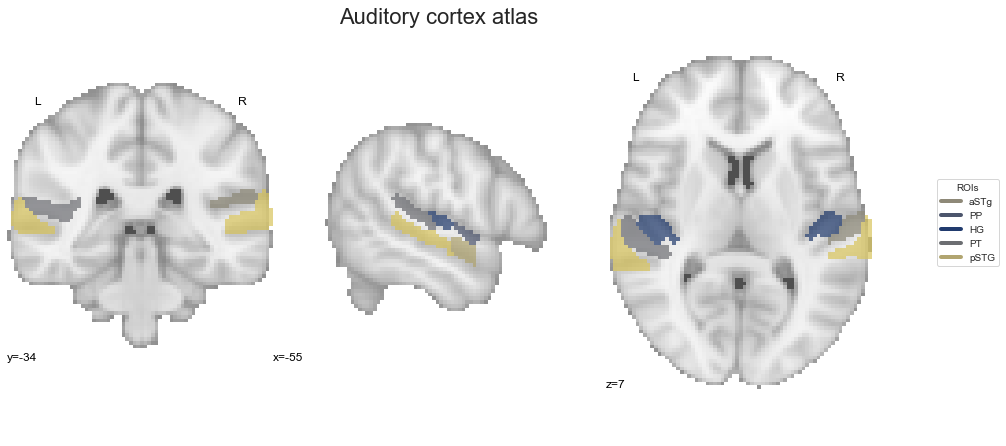
Looks alright (please note that the “holes” are based on the slice and because we didn’t dilate the ROIs). Next, we need to provide information about the atlas, more precisely label names
and their index
. To do so, we will follow the order of ROIs as indicated above:
regions = ['HG_lh', 'HG_rh', 'PP_lh', 'PP_rh', 'PT_lh',
'PT_rh', 'aSTG_lh', 'aSTG_rh', 'pSTG_lh', 'pSTG_rh']
atlas_rois_df = pd.DataFrame({'index': np.arange(1,11),
'regions': regions})
atlas_rois_df.to_csv(opj(prereq_path, 'atlas/AC_atlas_regions.txt'), sep="\t", index=False)
atlas_rois_df
index | regions | |
---|---|---|
0 | 1 | HG_lh |
1 | 2 | HG_rh |
2 | 3 | PP_lh |
3 | 4 | PP_rh |
4 | 5 | PT_lh |
5 | 6 | PT_rh |
6 | 7 | aSTG_lh |
7 | 8 | aSTG_rh |
8 | 9 | pSTG_lh |
9 | 10 | pSTG_rh |
In order to provide folks with an idea of the atlas
and corresponding connections
we created, we are going to visualize the resulting network structure. More precisely, we will visualize the network structure “as is”, meaning fully connected and not weighted. We will use this as a graphical explanation and a starting point from which we will specify network model variants later on.
In a first step we will define a connectivity matrix that is a) fully connected, b) no intrinsic connections and c) have different ‘weights’ for intra- and inter-hemispheric connections respectively. Please note that the c) is only included to visualize these connections differently in the graphic we are going to create.
network_structure_full = np.array([[0., 2., 1., 2., 1., 2., 1., 2., 1., 2.],
[2., 0., 2., 1., 2., 1., 2., 1., 2., 1.],
[1., 2., 0., 2., 1., 2., 1., 2., 1., 2.],
[2., 1., 2., 0., 2., 1., 2., 1., 2., 1.],
[1., 2., 1., 2., 0., 2., 1., 2., 1., 2.],
[2., 1., 2., 1., 2., 0., 2., 1., 2., 1.],
[1., 2., 1., 2., 1., 2., 0., 2., 1., 2.],
[2., 1., 2., 1., 2., 1., 2., 0., 2., 1.],
[1., 2., 1., 2., 1., 2., 1., 2., 0., 2.],
[2., 1., 2., 1., 2., 1., 2., 1., 2., 0.]])
Coolio, now to the graphic itself. What follows might seem a bit rough and convoluted and there are definitely other, more suitable ways of achieving the wanted outcome. However, this approach worked for nicely within the project and we are going to put it into a function that we can use throughout the analyses.
def plot_auditory_cortex_connectome(network_connections, name=None, model=True, n_lines=None):
regions = atlas_rois_df['regions'].str.replace('_', ' ', regex=True)
# Reorder the labels based on their location
lh_labels = ['pSTG lh', 'PT lh', 'HG lh', 'PP lh', 'aSTG lh']
# For the right hemi
rh_labels = [label[:-2] + 'rh' for label in lh_labels]
# Save the plot order
node_order = lh_labels[::-1] + rh_labels
node_angles = circular_layout(regions, node_order, start_pos=90,
group_boundaries=[0, len(atlas_rois_df['regions']) // 2])
cmap = ListedColormap(sns.color_palette('cividis'))#ac_atlas_cmap)
node_color = []
#for value in [0, 0.15, 0.30, 0.45, 0.60]:
for value in [0, 1, 2, 3, 4]:
node_color.append(cmap(value)[:-1])
node_color.append(cmap(value)[:-1])
node_color
fig = plt.figure(num=None, figsize=(8, 8), facecolor='white')
fig.text(0.85, 0.85, 'right hemisphere', size=13)
fig.text(0, 0.85, 'left hemisphere', size=13)
l1 = matplotlib.lines.Line2D([0.515, 0.515], [0.15, 0.85],
color='black', linestyle='--', transform=fig.transFigure, figure=fig)
if model:
anterior_path = plt.arrow(1, 0.52, 0, 0.2, ec='none', color='black', linestyle='--', head_width=0.01, transform=fig.transFigure, figure=fig, clip_on=False)
posterior_path = plt.arrow(1, 0.48, 0, -0.2, ec="none", color='black', head_width=0.01, transform=fig.transFigure, figure=fig, clip_on=False)
fig.text(1.02, 0.485, ' primary\n auditory cortex', size=13)
fig.text(1.02, 0.7, 'anterior regions', size=13)
fig.text(1.02, 0.3, 'posterior regions', size=13)
fig.lines.extend([l1, anterior_path, posterior_path])
cmap_connections = plt.cm.Set1_r
custom_lines = [matplotlib.lines.Line2D([0], [0], color=cmap_connections(0), lw=4),
matplotlib.lines.Line2D([0], [0], color='black', lw=4, linestyle=':'),
matplotlib.lines.Line2D([0], [0], color=cmap_connections(10), lw=4)]
fig.legend(custom_lines, ['intra-hemispheric', 'hemisphere border', 'inter-hemispheric'], bbox_to_anchor=(1.15, 0.07), loc='lower center', frameon=False)
if name is None:
title = 'Auditory cortex parcellation and connections'
else:
title='Auditory cortex parcellation and connections - %s' % name
if n_lines is not None:
n_lines=n_lines
plot_connectivity_circle(network_connections, regions,
node_angles=node_angles, n_lines=n_lines,
fig=fig, title=title, fontsize_title=20,
colorbar=False, padding=0.7, fontsize_names=10,
facecolor='white', textcolor='black', node_edgecolor='white',
node_colors=node_color, colormap=cmap_connections)
Puh, that was something else, eh? Let’s check it out:
plot_auditory_cortex_connectome(network_structure_full, name='fully connected')
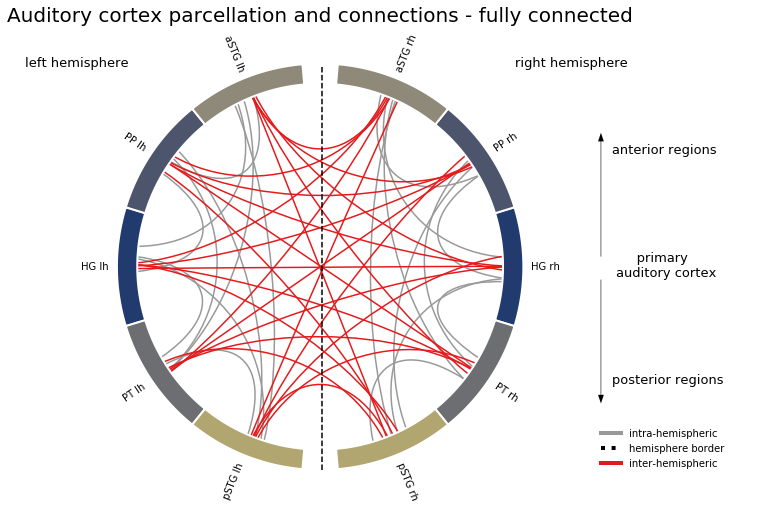
But the graphic is quite nice and informative (at least in my obviously unbiased opinion). We can see the structure of the network based on the parcellation (atlas) of the auditory cortex we used. We marked the different hemispheres as well as the corresponding connections between their homotopic and heterotopic regions. We also included information with regard to spatial aspects (anterior vs. posterior pathways).
Now it’s time to go to the next section, that is adapting our event files
to be compliant with the NiBetaSeries
package.
Event file adaption¶
As we intend to use NiBetaSeries
, we need to adapt our event files
as it assumes, or rather expects a certain structure as described in the corresponding section of the NiBetaSeries
docs. Luckily, our event files are already BIDS compliant which should bring us almost all the way to be NiBetaSeries compliant
. Let’s check what the latter needs and what we already have.
From the NiBetaSeries
docs we know that our event files
need to have the following information: onset
, duration
, trial_type
and correct
. Great, now we can check and establish the adaption using the event files
of sub-01
. First, we load the event file
of the first run using pandas:
sub01_events = pd.read_csv(opj(data_path, 'sub-01/func/sub-01_task-mvs_run-01_events.tsv'), sep='\t')
Now, let’s inspect it:
sub01_events
Unnamed: 0 | trial_type | onset | duration | |
---|---|---|---|---|
0 | 0 | Music | 4.710 | 1.87 |
1 | 1 | Music | 8.726 | 1.76 |
2 | 2 | Music | 20.776 | 1.68 |
3 | 3 | Music | 24.792 | 1.76 |
4 | 4 | Music | 28.809 | 1.57 |
... | ... | ... | ... | ... |
85 | 85 | Voice | 337.496 | 1.39 |
86 | 86 | Voice | 341.513 | 1.97 |
87 | 87 | Voice | 353.546 | 1.71 |
88 | 88 | Voice | 357.562 | 1.43 |
89 | 89 | Voice | 361.562 | 1.86 |
90 rows × 4 columns
As predicted, we are almost there already, as the event files
have onset
, duration
and trial_type
. We’re only missing correct
. We can add this as easy as:
sub01_events['correct'] = 'Y'
sub01_events
Unnamed: 0 | trial_type | onset | duration | correct | |
---|---|---|---|---|---|
0 | 0 | Music | 4.710 | 1.87 | Y |
1 | 1 | Music | 8.726 | 1.76 | Y |
2 | 2 | Music | 20.776 | 1.68 | Y |
3 | 3 | Music | 24.792 | 1.76 | Y |
4 | 4 | Music | 28.809 | 1.57 | Y |
... | ... | ... | ... | ... | ... |
85 | 85 | Voice | 337.496 | 1.39 | Y |
86 | 86 | Voice | 341.513 | 1.97 | Y |
87 | 87 | Voice | 353.546 | 1.71 | Y |
88 | 88 | Voice | 357.562 | 1.43 | Y |
89 | 89 | Voice | 361.562 | 1.86 | Y |
90 rows × 5 columns
The beauty of pandas
(meant here is the python package, but the same holds true for the animal as well)… .
While we’re at it, we can address the mysterious Unnamed: 0
column. This is the result of saving pandas DataFrame
to file without specifying the following index=False
. Instead of correcting it and working with “fitting” files from the beginning, I decided to leave them as is to show that many problems/errors can occur along the way of an analysis, but as long as you are aware of them and address them in a reproducible and open way, it’s all good. These things happen, even to the most experienced folks (to which I don’t belong). As it doesn’t contain useful information, but redundant, we can just delete it. Again, with pandas
it’s as easy as:
del sub01_events['Unnamed: 0']
Now, everything we need to do is to save our modified events file
. While it’s strictly not “wrong” to just overwrite the original file within the BIDS root
directory, I decided to place them in the derivatives
directory that entails the data processed within the NiBetaSeries
analyses. Thus, we will add the desc
identifier to note this accordingly:
sub01_events.to_csv(opj(derivatives_path, 'data_prerequisites', 'sub-01_task-mvs_run-01_events_desc-nibetaseries.tsv'))
To avoid doing this for all participants and their event files
manually (and a lot of potential errors within that process), we’ll define a short function:
def adapt_eventfiles_nibetaseries(event_file):
import os.path
filename_tmp = event_file[event_file.rfind('/')+1:]
participant_tmp = event_file[event_file.rfind('/')+1:event_file.rfind('/')+7]
event_file_tmp = pd.read_csv(event_file, sep='\t')
event_file_tmp['correct'] = 'Y'
del event_file_tmp['Unnamed: 0']
if not os.path.exists(opj(derivatives_path, 'data_prerequisites/event_files/%s' %participant_tmp)):
makedirs(opj(derivatives_path, 'data_prerequisites/event_files/%s' %participant_tmp))
event_file_tmp.to_csv(event_file, index=False, sep='\t')
Now, we only have to gather all event files
using glob:
list_event_files = glob(opj(data_path, '*/*/*events.tsv'))
list_event_files.sort()
and apply our function to every single one using a for loop
:
for event_file in list_event_files:
adapt_eventfiles_nibetaseries(event_file)
Check confounds¶
As for all other types of analyses within the realm of linear models applied to fMRI data, checking and incorporating confounds is necessary and important. Following BIDS
, they are (should be) stored alongside the preprocessed images in the preprocessing
corresponding folder of the /derivatives
directory. For the data at hand, let’s say the first participant sub-01
they are called: sub-01_task-mvs_run-01_desc-confounds_regressors.tsv
and contain the following information:
pd.read_csv('/data/mvs/preprocessing/sub-01/func/sub-01_task-mvs_run-01_desc-confounds_regressors.tsv', sep="\t")
trans_x | trans_y | trans_z | rot_x | rot_y | rot_z | white_matter | csf | |
---|---|---|---|---|---|---|---|---|
0 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | -1.502010 | -0.423443 |
1 | 0.006862 | -0.039911 | -0.023868 | 0.000537 | -0.000208 | 0.000173 | -1.665055 | -0.481281 |
2 | 0.000248 | -0.033173 | -0.020424 | 0.000789 | -0.000198 | 0.000198 | -1.455152 | -1.819274 |
3 | 0.000808 | -0.010968 | -0.005223 | 0.001199 | -0.000459 | 0.000025 | -1.621729 | -1.624893 |
4 | -0.002599 | 0.083570 | 0.030288 | -0.000253 | 0.000043 | -0.000099 | -1.288876 | -0.910469 |
... | ... | ... | ... | ... | ... | ... | ... | ... |
785 | -0.108433 | -0.068326 | -0.110301 | 0.001941 | -0.001557 | -0.002760 | 0.937084 | 1.691596 |
786 | -0.103787 | -0.046885 | -0.108684 | 0.001634 | -0.001611 | -0.002875 | 0.438706 | 1.077597 |
787 | -0.120564 | -0.015005 | -0.100488 | 0.001792 | -0.001597 | -0.003026 | 0.157408 | 1.916833 |
788 | -0.118234 | 0.020811 | -0.107692 | 0.000639 | -0.001524 | -0.003150 | -0.176196 | 0.789305 |
789 | -0.123682 | -0.045449 | -0.106099 | 0.001523 | -0.001420 | -0.003110 | 0.391247 | 1.270234 |
790 rows × 8 columns
As you can see, the preprocessing pipeline provided us with 8 confounds: trans_x
, trans_y
, trans_z
, rot_x
, rot_y
, rot_z
, white_matter
and csf
for each of the acquired and preprocessed 790 images. While the first six are motion related confounds
obtained through motion correction entailing the deviation from the mean image by means of rotation
and translation
in x
, y
, z
, the last two entail the z-scored
mean signal present in white_matter
and csf
. The question of which and how many confound regressors should be included in a given model is discussed in a very active debatte with no clear “one-approach-fits-all” outcome as everything is dependent on the data and factors like the correlation between confounds. Compared to comprehensive pipelines like fmriprep we have only a few, but however the most common ones on which important’s most folks converge. To keep things comparable to the model we run during the univariate analyses as the basis for the MVPA, we will include only the motion related confounds
within the subsequent NiBetaSeries
analysis for which we are now ready.
Please note: As mentioned before, the to-be computed beta series
are the derivatives we can share and thus work with here. Based on that and given that the computation of these files is super heavy (computationally speaking), we will only show and explain how we computed them, but not actually run code. As in the MVPA, we will provide code that will download these files from the project’s OSF page.
NiBetaSeries¶
As we now have and checked everything we need to run the planned NiBetaSeries
analysis, we can set it up accordingly hereby closely following the NiBetaSeries
docs. In general, we’ll conduct a two step procedure: first, we’ll compute responses to our presented conditions per participant, trial and run and second, transform the resulting beta series images
to the MNI152 template space
. The latter is required as the first step will be conducted in each participant’s native functional space
. Comparable to the univariate results, we will apply spatial transformations only to model outcomes
(here beta series
). For the analyses, we need to define some paths that point to the data:
out_dir = '/data/mvs/derivatives/connectivity/results/nibetaseries'
work_dir = '/data/mvs/derivatives/connectivity/reults/nibetaseries/work'
data_dir = '/data/mvs'
Model fitting¶
Next, we define how NiBetaSeries
should be run, printing the resulting bash
command for evaluation:
cmd = """\
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y \
--participant-label 01 \
--estimator lss \
--hrf-model spm \
-w {work_dir} \
-sp {space_label} \
--sm {smoothing} \
--description-label 'preproc' \
{bids_dir} \
preprocessing \
{out_dir} \
participant
""".format(bids_dir=data_dir,
out_dir=out_dir,
work_dir=work_dir,
space_label='individual',
smoothing=0.0)
print("The NiBetaSeries Command looks as follows:\n", cmd)
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label 01 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
In more detail, we include our six confounds rot_x, rot_y, rot_z, trans_x, trans_y and trans_y
, running the analysis for sub-01
. For the model, we use a lss
(least squares separate
) estimator and the SPM hemodynamic model
and indicate the space
we are running the analysis in (individual
). We set smoothing
to 0.0
as we already applied a 6 mm FWHM
Gaussian smoothing kernel to the images during preprocessing. We furthermore set the description label
to use preproc
(preprocessed
) data and define working
, data
and output
directories, as well as the name of the utilized preprocessing pipeline
(preprocessing
) and that we want to run the analysis on the participant
level. We don’t specify a high-pass
filter as NiBetaSeries
default of 0.0078 Hz
is what we want anyway.
So far, so good. Let’s run it:
p = Popen(cmd, shell=True, stdout=PIPE, stderr=STDOUT)
while True:
line = p.stdout.readline()
if not line:
break
print(line)
b'200625-20:03:42,408 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200625-20:03:42,773 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200625-20:03:42,796 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200625-20:03:42,802 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200625-20:03:43,30 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/betaseries_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/betaseries_node".\n'
b'200625-20:03:43,31 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/betaseries_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/betaseries_node".\n'
b'200625-20:03:43,37 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-20:03:43,37 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-20:03:44,809 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 64 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200625-20:55:28,491 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node".\n'
b'200625-20:55:29,519 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node).\n'
b'200625-20:55:29,523 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node\n'
b'200625-20:55:31,528 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node\n'
b'200625-20:55:31,617 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-20:55:31,621 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:55:31,623 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-20:55:31,623 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-20:55:31,627 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:55:31,628 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:55:31,696 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-20:55:31,696 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-20:55:31,742 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-20:55:33,535 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200625-20:55:33,536 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200625-20:55:33,536 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200625-20:55:33,538 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node\n'
b'200625-20:55:33,689 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file".\n'
b'200625-20:55:33,701 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-20:55:33,707 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:55:33,843 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-20:55:33,845 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-20:55:33,853 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:55:33,944 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-20:55:33,946 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-20:55:33,949 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:55:34,14 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-20:55:34,18 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file".\n'
b'200625-20:55:35,543 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file).\n'
b'200625-20:55:35,545 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200625-20:56:45,939 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node".\n'
b'200625-20:56:47,831 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node).\n'
b'200625-20:56:47,834 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-20:56:49,839 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-20:56:49,915 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-20:56:49,915 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-20:56:49,915 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-20:56:49,919 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:56:49,919 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:56:49,920 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:56:49,975 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-20:56:49,977 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-20:56:49,977 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-20:56:51,847 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200625-20:56:51,848 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200625-20:56:51,848 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200625-20:56:51,850 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-20:56:51,928 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file".\n'
b'200625-20:56:51,936 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-20:56:51,940 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:56:52,8 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-20:56:52,10 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-20:56:52,13 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:56:52,290 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-20:56:52,292 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-20:56:52,295 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-20:56:52,355 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-20:56:52,359 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file".\n'
b'200625-20:56:53,855 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file).\n'
b'200625-20:56:53,857 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
That seems to work, great! Now we set up a little for-loop
that runs NiBetaSeries
for each participant
:
for part in ['sub-0%s' %s for s in range(1,10)] + ['sub-%s' %s for s in range(10,25)]:
cmd = """\
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y \
--participant-label {part} \
--estimator lss \
--hrf-model spm \
-w {work_dir} \
-sp {space_label} \
--sm {smoothing} \
--description-label 'preproc' \
{bids_dir} \
preprocessing \
{out_dir} \
participant
""".format(part=part,
bids_dir=data_dir,
out_dir=out_dir,
work_dir=work_dir,
space_label='individual',
smoothing=0.0)
print("The NiBetaSeries Command looks as follows:\n", cmd)
p = Popen(cmd, shell=True, stdout=PIPE, stderr=STDOUT)
while True:
line = p.stdout.readline()
if not line:
break
print(line)
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-01 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200625-21:03:24,424 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200625-21:03:24,786 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200625-21:03:24,797 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200625-21:03:24,801 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-21:03:24,901 nipype.workflow INFO:\n'
b'\t [Job 0] Cached (nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node).\n'
b'200625-21:03:24,903 nipype.workflow INFO:\n'
b'\t [Job 2] Cached (nibetaseries_participant_wf.single_subject01_wf.betaseries_wf.betaseries_node).\n'
b'200625-21:03:28,816 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 6 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200625-21:03:29,82 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-21:03:29,87 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:29,88 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-21:03:29,92 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:29,92 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-21:03:29,93 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-21:03:29,94 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-21:03:29,95 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-21:03:29,96 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:29,97 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:29,98 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:29,99 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:29,163 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-21:03:29,164 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-21:03:29,165 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-21:03:29,451 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-21:03:29,579 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-21:03:29,585 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-21:03:30,824 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200625-21:03:30,825 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200625-21:03:30,826 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200625-21:03:30,827 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200625-21:03:30,827 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200625-21:03:30,828 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200625-21:03:30,830 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-21:03:30,952 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file".\n'
b'200625-21:03:30,954 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file".\n'
b'200625-21:03:30,960 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-21:03:30,961 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-21:03:30,964 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:30,964 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:31,182 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-21:03:31,184 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-21:03:31,184 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-21:03:31,186 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-21:03:31,186 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:31,189 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:31,248 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-21:03:31,249 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/46c932c050b74635cf7e7b4f5f5070056ae6700f/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-21:03:31,252 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:31,253 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-21:03:31,254 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject01_wf/41f1685cf5629f60d8b0e7b251d76d90a539d725/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-21:03:31,257 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-21:03:31,311 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-21:03:31,314 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file".\n'
b'200625-21:03:31,467 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-21:03:31,470 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file".\n'
b'200625-21:03:32,831 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file).\n'
b'200625-21:03:32,832 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject01_wf.ds_betaseries_file).\n'
b'200625-21:03:32,834 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-02 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200625-21:03:46,36 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200625-21:03:46,438 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200625-21:03:46,470 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200625-21:03:46,474 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200625-21:03:46,704 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/betaseries_wf/e9b87896768d4f8c2569df1ad0dcf3d6482b9e32/betaseries_node".\n'
b'200625-21:03:46,709 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/betaseries_wf/9dd8ef680c5b675b7d4d87f65955305d466f0bab/betaseries_node".\n'
b'200625-21:03:46,711 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-21:03:46,715 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-21:03:48,482 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200625-22:01:26,698 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node".\n'
b'200625-22:01:28,351 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node).\n'
b'200625-22:01:28,354 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node\n'
b'200625-22:01:30,360 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node\n'
b'200625-22:01:30,447 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/9dd8ef680c5b675b7d4d87f65955305d466f0bab/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-22:01:30,447 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/9dd8ef680c5b675b7d4d87f65955305d466f0bab/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-22:01:30,448 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/9dd8ef680c5b675b7d4d87f65955305d466f0bab/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-22:01:30,998 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:31,2 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:31,2 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:31,57 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-22:01:31,61 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-22:01:31,70 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-22:01:32,367 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200625-22:01:32,368 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200625-22:01:32,368 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200625-22:01:32,370 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node\n'
b'200625-22:01:32,456 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject02_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/9dd8ef680c5b675b7d4d87f65955305d466f0bab/ds_betaseries_file".\n'
b'200625-22:01:32,462 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/9dd8ef680c5b675b7d4d87f65955305d466f0bab/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-22:01:32,465 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:32,680 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-22:01:32,682 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/9dd8ef680c5b675b7d4d87f65955305d466f0bab/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-22:01:32,685 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:32,759 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-22:01:32,761 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/9dd8ef680c5b675b7d4d87f65955305d466f0bab/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-22:01:32,764 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:32,823 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-22:01:32,826 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject02_wf.ds_betaseries_file".\n'
b'200625-22:01:34,375 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject02_wf.ds_betaseries_file).\n'
b'200625-22:01:34,377 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node\n'
b'200625-22:01:46,228 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node".\n'
b'200625-22:01:46,423 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject02_wf.betaseries_wf.betaseries_node).\n'
b'200625-22:01:46,425 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-22:01:48,432 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-22:01:48,512 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/e9b87896768d4f8c2569df1ad0dcf3d6482b9e32/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-22:01:48,514 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/e9b87896768d4f8c2569df1ad0dcf3d6482b9e32/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-22:01:48,515 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/e9b87896768d4f8c2569df1ad0dcf3d6482b9e32/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-22:01:48,516 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:48,518 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:48,518 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:48,576 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-22:01:48,577 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-22:01:48,581 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-22:01:50,439 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200625-22:01:50,440 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200625-22:01:50,440 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200625-22:01:50,442 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-22:01:50,548 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject02_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/e9b87896768d4f8c2569df1ad0dcf3d6482b9e32/ds_betaseries_file".\n'
b'200625-22:01:50,556 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/e9b87896768d4f8c2569df1ad0dcf3d6482b9e32/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-22:01:50,559 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:50,627 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-22:01:50,629 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/e9b87896768d4f8c2569df1ad0dcf3d6482b9e32/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-22:01:50,632 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:50,907 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-22:01:50,909 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject02_wf/e9b87896768d4f8c2569df1ad0dcf3d6482b9e32/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-22:01:50,912 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-22:01:50,982 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-22:01:50,986 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject02_wf.ds_betaseries_file".\n'
b'200625-22:01:52,447 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject02_wf.ds_betaseries_file).\n'
b'200625-22:01:52,455 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-03 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200625-22:02:05,265 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200625-22:02:05,613 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200625-22:02:05,629 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200625-22:02:05,633 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200625-22:02:05,852 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/betaseries_wf/5ecbed86770e5c7a8d7eff21d3a172e567b9864a/betaseries_node".\n'
b'200625-22:02:05,854 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/betaseries_wf/2a102ec8eb043337471ce6cd7a099be27192049a/betaseries_node".\n'
b'200625-22:02:05,858 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-22:02:05,861 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-22:02:07,641 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200625-23:00:21,937 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node".\n'
b'200625-23:00:23,651 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node).\n'
b'200625-23:00:23,653 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node\n'
b'200625-23:00:25,659 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node\n'
b'200625-23:00:25,741 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/5ecbed86770e5c7a8d7eff21d3a172e567b9864a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-23:00:25,746 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:25,748 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/5ecbed86770e5c7a8d7eff21d3a172e567b9864a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-23:00:25,748 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/5ecbed86770e5c7a8d7eff21d3a172e567b9864a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-23:00:25,752 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:25,753 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:25,811 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-23:00:25,812 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-23:00:25,817 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-23:00:27,667 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200625-23:00:27,668 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200625-23:00:27,668 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200625-23:00:27,670 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node\n'
b'200625-23:00:27,747 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject03_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/5ecbed86770e5c7a8d7eff21d3a172e567b9864a/ds_betaseries_file".\n'
b'200625-23:00:27,954 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/5ecbed86770e5c7a8d7eff21d3a172e567b9864a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-23:00:27,957 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:28,17 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-23:00:28,19 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/5ecbed86770e5c7a8d7eff21d3a172e567b9864a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-23:00:28,22 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:28,74 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-23:00:28,75 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/5ecbed86770e5c7a8d7eff21d3a172e567b9864a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-23:00:28,78 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:28,290 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-23:00:28,293 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject03_wf.ds_betaseries_file".\n'
b'200625-23:00:29,675 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject03_wf.ds_betaseries_file).\n'
b'200625-23:00:29,677 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node\n'
b'200625-23:00:37,718 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node".\n'
b'200625-23:00:39,716 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject03_wf.betaseries_wf.betaseries_node).\n'
b'200625-23:00:39,718 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-23:00:41,724 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-23:00:41,805 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/2a102ec8eb043337471ce6cd7a099be27192049a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-23:00:41,809 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/2a102ec8eb043337471ce6cd7a099be27192049a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-23:00:41,809 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/2a102ec8eb043337471ce6cd7a099be27192049a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-23:00:41,810 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:41,813 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:41,813 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:41,868 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-23:00:41,870 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-23:00:41,874 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-23:00:43,731 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200625-23:00:43,732 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200625-23:00:43,732 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200625-23:00:43,734 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-23:00:43,816 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject03_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/2a102ec8eb043337471ce6cd7a099be27192049a/ds_betaseries_file".\n'
b'200625-23:00:43,825 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/2a102ec8eb043337471ce6cd7a099be27192049a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-23:00:43,828 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:43,894 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-23:00:43,896 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/2a102ec8eb043337471ce6cd7a099be27192049a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-23:00:43,899 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:44,429 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-23:00:44,430 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject03_wf/2a102ec8eb043337471ce6cd7a099be27192049a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-23:00:44,433 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:00:44,491 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-23:00:44,755 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject03_wf.ds_betaseries_file".\n'
b'200625-23:00:45,739 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject03_wf.ds_betaseries_file).\n'
b'200625-23:00:45,741 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-04 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200625-23:01:03,77 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200625-23:01:03,416 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200625-23:01:03,447 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200625-23:01:03,452 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200625-23:01:03,702 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/betaseries_wf/4bbfbfcd4e871c0d330d5c73604ff65516d3b561/betaseries_node".\n'
b'200625-23:01:03,705 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/betaseries_wf/5775cc599d4cb6183b8b3683ae4263782618aab4/betaseries_node".\n'
b'200625-23:01:03,712 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-23:01:03,712 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-23:01:05,461 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 20 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200625-23:56:15,746 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node".\n'
b'200625-23:56:16,787 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node).\n'
b'200625-23:56:16,790 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node\n'
b'200625-23:56:18,795 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node\n'
b'200625-23:56:18,869 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/5775cc599d4cb6183b8b3683ae4263782618aab4/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-23:56:18,871 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/5775cc599d4cb6183b8b3683ae4263782618aab4/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-23:56:18,874 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:18,876 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:18,876 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/5775cc599d4cb6183b8b3683ae4263782618aab4/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-23:56:18,880 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:18,927 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-23:56:18,939 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-23:56:18,944 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-23:56:20,803 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200625-23:56:20,804 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200625-23:56:20,805 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200625-23:56:20,807 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node\n'
b'200625-23:56:20,920 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject04_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/5775cc599d4cb6183b8b3683ae4263782618aab4/ds_betaseries_file".\n'
b'200625-23:56:20,929 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/5775cc599d4cb6183b8b3683ae4263782618aab4/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-23:56:20,932 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:20,993 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-23:56:20,996 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/5775cc599d4cb6183b8b3683ae4263782618aab4/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-23:56:20,999 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:21,53 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-23:56:21,54 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/5775cc599d4cb6183b8b3683ae4263782618aab4/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-23:56:21,57 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:21,279 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-23:56:21,282 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject04_wf.ds_betaseries_file".\n'
b'200625-23:56:22,811 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject04_wf.ds_betaseries_file).\n'
b'200625-23:56:22,813 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node\n'
b'200625-23:56:30,820 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node".\n'
b'200625-23:56:30,849 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject04_wf.betaseries_wf.betaseries_node).\n'
b'200625-23:56:30,851 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-23:56:32,856 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-23:56:32,969 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/4bbfbfcd4e871c0d330d5c73604ff65516d3b561/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-23:56:32,973 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/4bbfbfcd4e871c0d330d5c73604ff65516d3b561/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-23:56:32,973 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:32,973 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/4bbfbfcd4e871c0d330d5c73604ff65516d3b561/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-23:56:32,977 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:32,977 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:33,30 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-23:56:33,33 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-23:56:33,35 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-23:56:34,863 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200625-23:56:34,864 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200625-23:56:34,864 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200625-23:56:34,866 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200625-23:56:34,981 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject04_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/4bbfbfcd4e871c0d330d5c73604ff65516d3b561/ds_betaseries_file".\n'
b'200625-23:56:34,989 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/4bbfbfcd4e871c0d330d5c73604ff65516d3b561/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200625-23:56:34,993 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:35,274 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200625-23:56:35,276 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/4bbfbfcd4e871c0d330d5c73604ff65516d3b561/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200625-23:56:35,279 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:35,560 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200625-23:56:35,562 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject04_wf/4bbfbfcd4e871c0d330d5c73604ff65516d3b561/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200625-23:56:35,566 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200625-23:56:35,633 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200625-23:56:35,637 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject04_wf.ds_betaseries_file".\n'
b'200625-23:56:36,871 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject04_wf.ds_betaseries_file).\n'
b'200625-23:56:36,874 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-05 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200625-23:56:50,411 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200625-23:56:50,800 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200625-23:56:50,813 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200625-23:56:50,816 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200625-23:56:51,55 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/betaseries_wf/90a5b29dd9af792a53810f7481e30667e3507490/betaseries_node".\n'
b'200625-23:56:51,61 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/betaseries_wf/870c69db8abe524f5425abe1f1bdf95cc041662b/betaseries_node".\n'
b'200625-23:56:51,63 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-23:56:51,68 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200625-23:56:52,825 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-00:34:50,126 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node".\n'
b'200626-00:34:52,35 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node).\n'
b'200626-00:34:52,37 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node\n'
b'200626-00:34:54,48 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node\n'
b'200626-00:34:54,144 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/870c69db8abe524f5425abe1f1bdf95cc041662b/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-00:34:54,146 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/870c69db8abe524f5425abe1f1bdf95cc041662b/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-00:34:54,147 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/870c69db8abe524f5425abe1f1bdf95cc041662b/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-00:34:54,149 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:34:54,151 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:34:54,152 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:34:54,208 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-00:34:54,213 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-00:34:54,217 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-00:34:56,55 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-00:34:56,56 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-00:34:56,56 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-00:34:56,58 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node\n'
b'200626-00:34:56,141 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject05_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/870c69db8abe524f5425abe1f1bdf95cc041662b/ds_betaseries_file".\n'
b'200626-00:34:56,149 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/870c69db8abe524f5425abe1f1bdf95cc041662b/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-00:34:56,152 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:34:56,219 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-00:34:56,222 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/870c69db8abe524f5425abe1f1bdf95cc041662b/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-00:34:56,224 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:34:56,514 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-00:34:56,516 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/870c69db8abe524f5425abe1f1bdf95cc041662b/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-00:34:56,519 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:34:56,691 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-00:34:56,694 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject05_wf.ds_betaseries_file".\n'
b'200626-00:34:58,63 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject05_wf.ds_betaseries_file).\n'
b'200626-00:34:58,65 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-00:51:40,588 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node".\n'
b'200626-00:51:42,99 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject05_wf.betaseries_wf.betaseries_node).\n'
b'200626-00:51:42,101 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-00:51:44,108 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-00:51:44,191 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/90a5b29dd9af792a53810f7481e30667e3507490/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-00:51:44,191 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/90a5b29dd9af792a53810f7481e30667e3507490/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-00:51:44,192 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/90a5b29dd9af792a53810f7481e30667e3507490/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-00:51:44,195 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:51:44,196 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:51:44,196 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:51:44,253 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-00:51:44,254 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-00:51:44,254 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-00:51:46,115 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-00:51:46,116 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-00:51:46,116 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-00:51:46,119 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-00:51:46,201 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject05_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/90a5b29dd9af792a53810f7481e30667e3507490/ds_betaseries_file".\n'
b'200626-00:51:46,209 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/90a5b29dd9af792a53810f7481e30667e3507490/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-00:51:46,212 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:51:46,270 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-00:51:46,272 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/90a5b29dd9af792a53810f7481e30667e3507490/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-00:51:46,275 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:51:46,578 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-00:51:46,580 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject05_wf/90a5b29dd9af792a53810f7481e30667e3507490/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-00:51:46,587 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-00:51:46,742 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-00:51:46,745 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject05_wf.ds_betaseries_file".\n'
b'200626-00:51:48,123 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject05_wf.ds_betaseries_file).\n'
b'200626-00:51:48,125 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-06 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-00:52:00,376 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-00:52:00,745 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-00:52:00,759 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-00:52:00,762 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-00:52:01,11 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/betaseries_wf/e7f8b531849c8bec8fb1516ea84c260e5a493169/betaseries_node".\n'
b'200626-00:52:01,14 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/betaseries_wf/87635a7c9694753fcdd29a126012f20639475ce0/betaseries_node".\n'
b'200626-00:52:01,17 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-00:52:01,20 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-00:52:02,770 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 21 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-01:30:40,520 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node".\n'
b'200626-01:30:42,151 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node).\n'
b'200626-01:30:42,154 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node\n'
b'200626-01:30:44,160 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node\n'
b'200626-01:30:44,244 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/e7f8b531849c8bec8fb1516ea84c260e5a493169/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-01:30:44,247 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/e7f8b531849c8bec8fb1516ea84c260e5a493169/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-01:30:44,248 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/e7f8b531849c8bec8fb1516ea84c260e5a493169/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-01:30:44,252 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:30:44,252 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:30:44,254 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:30:44,309 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-01:30:44,311 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-01:30:44,311 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-01:30:46,168 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-01:30:46,169 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-01:30:46,169 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-01:30:46,171 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node\n'
b'200626-01:30:46,253 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject06_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/e7f8b531849c8bec8fb1516ea84c260e5a493169/ds_betaseries_file".\n'
b'200626-01:30:46,262 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/e7f8b531849c8bec8fb1516ea84c260e5a493169/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-01:30:46,265 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:30:46,334 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-01:30:46,336 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/e7f8b531849c8bec8fb1516ea84c260e5a493169/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-01:30:46,339 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:30:46,486 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-01:30:46,487 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/e7f8b531849c8bec8fb1516ea84c260e5a493169/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-01:30:46,490 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:30:46,546 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-01:30:46,549 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject06_wf.ds_betaseries_file".\n'
b'200626-01:30:48,175 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject06_wf.ds_betaseries_file).\n'
b'200626-01:30:48,177 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-01:49:56,149 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node".\n'
b'200626-01:49:56,778 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject06_wf.betaseries_wf.betaseries_node).\n'
b'200626-01:49:56,780 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-01:49:58,788 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-01:49:58,871 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/87635a7c9694753fcdd29a126012f20639475ce0/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-01:49:58,872 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/87635a7c9694753fcdd29a126012f20639475ce0/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-01:49:58,872 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/87635a7c9694753fcdd29a126012f20639475ce0/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-01:49:58,876 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:49:58,876 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:49:58,876 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:49:58,945 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-01:49:58,945 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-01:49:58,945 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-01:50:00,795 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-01:50:00,796 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-01:50:00,797 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-01:50:00,799 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-01:50:00,889 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject06_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/87635a7c9694753fcdd29a126012f20639475ce0/ds_betaseries_file".\n'
b'200626-01:50:00,896 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/87635a7c9694753fcdd29a126012f20639475ce0/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-01:50:00,900 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:50:01,58 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-01:50:01,60 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/87635a7c9694753fcdd29a126012f20639475ce0/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-01:50:01,63 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:50:01,124 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-01:50:01,125 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject06_wf/87635a7c9694753fcdd29a126012f20639475ce0/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-01:50:01,128 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-01:50:01,186 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-01:50:02,804 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject06_wf.ds_betaseries_file\n'
b'200626-01:50:03,278 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject06_wf.ds_betaseries_file".\n'
b'200626-01:50:04,811 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject06_wf.ds_betaseries_file).\n'
b'200626-01:50:04,813 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-07 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-01:50:16,777 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-01:50:17,141 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-01:50:17,151 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-01:50:17,155 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-01:50:17,390 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/betaseries_wf/016a89127b172af618f8548aa6076b42a529c9e9/betaseries_node".\n'
b'200626-01:50:17,391 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/betaseries_wf/b61c30c7dbbad8d115b1fda5a00a43114f7654be/betaseries_node".\n'
b'200626-01:50:17,396 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-01:50:17,397 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-01:50:19,161 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 69 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 72 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 65 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 70 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 69 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 67 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 73 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 64 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 65 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 64 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 68 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 64 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 71 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 78 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 70 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 73 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 72 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 67 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 66 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 66 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 65 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 68 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 73 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 67 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 83 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 65 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 90 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 86 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 69 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 68 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 72 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 65 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-03:18:10,739 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node".\n'
b'200626-03:18:12,248 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node).\n'
b'200626-03:18:12,251 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node\n'
b'200626-03:18:14,256 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node\n'
b'200626-03:18:14,332 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/b61c30c7dbbad8d115b1fda5a00a43114f7654be/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-03:18:14,332 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/b61c30c7dbbad8d115b1fda5a00a43114f7654be/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-03:18:14,335 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/b61c30c7dbbad8d115b1fda5a00a43114f7654be/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-03:18:14,336 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:18:14,336 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:18:14,339 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:18:14,445 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-03:18:14,448 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-03:18:14,451 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-03:18:16,263 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-03:18:16,264 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-03:18:16,264 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-03:18:16,266 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node\n'
b'200626-03:18:16,347 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject07_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/b61c30c7dbbad8d115b1fda5a00a43114f7654be/ds_betaseries_file".\n'
b'200626-03:18:16,355 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/b61c30c7dbbad8d115b1fda5a00a43114f7654be/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-03:18:16,358 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:18:16,434 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-03:18:16,436 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/b61c30c7dbbad8d115b1fda5a00a43114f7654be/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-03:18:16,438 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:18:16,508 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-03:18:16,509 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/b61c30c7dbbad8d115b1fda5a00a43114f7654be/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-03:18:16,512 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:18:17,104 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-03:18:17,107 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject07_wf.ds_betaseries_file".\n'
b'200626-03:18:18,271 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject07_wf.ds_betaseries_file).\n'
b'200626-03:18:18,273 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node\n'
b'200626-03:19:02,77 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node".\n'
b'200626-03:19:02,446 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject07_wf.betaseries_wf.betaseries_node).\n'
b'200626-03:19:02,448 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-03:19:04,456 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-03:19:04,538 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/016a89127b172af618f8548aa6076b42a529c9e9/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-03:19:04,539 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/016a89127b172af618f8548aa6076b42a529c9e9/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-03:19:04,541 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/016a89127b172af618f8548aa6076b42a529c9e9/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-03:19:04,542 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:19:04,543 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:19:04,545 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:19:04,607 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-03:19:04,611 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-03:19:04,618 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-03:19:06,463 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-03:19:06,464 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-03:19:06,464 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-03:19:06,466 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-03:19:06,576 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject07_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/016a89127b172af618f8548aa6076b42a529c9e9/ds_betaseries_file".\n'
b'200626-03:19:06,584 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/016a89127b172af618f8548aa6076b42a529c9e9/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-03:19:06,588 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:19:06,666 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-03:19:06,667 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/016a89127b172af618f8548aa6076b42a529c9e9/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-03:19:06,670 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:19:06,952 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-03:19:06,954 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject07_wf/016a89127b172af618f8548aa6076b42a529c9e9/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-03:19:06,957 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:19:07,22 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-03:19:07,24 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject07_wf.ds_betaseries_file".\n'
b'200626-03:19:08,473 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject07_wf.ds_betaseries_file).\n'
b'200626-03:19:08,475 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-08 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-03:19:26,628 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-03:19:27,311 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-03:19:27,329 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-03:19:27,333 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-03:19:27,685 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/betaseries_wf/4d51d90309303ab6edf2de2446a02330e4437ae3/betaseries_node".\n'
b'200626-03:19:27,686 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/betaseries_wf/3d2e1987a1f86b8badee7cfdec81d54c1554785d/betaseries_node".\n'
b'200626-03:19:27,692 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-03:19:27,692 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-03:19:29,341 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-03:58:29,698 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node".\n'
b'200626-03:58:30,791 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node).\n'
b'200626-03:58:30,793 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node\n'
b'200626-03:58:32,799 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node\n'
b'200626-03:58:32,877 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/3d2e1987a1f86b8badee7cfdec81d54c1554785d/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-03:58:32,879 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/3d2e1987a1f86b8badee7cfdec81d54c1554785d/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-03:58:32,880 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/3d2e1987a1f86b8badee7cfdec81d54c1554785d/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-03:58:32,883 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:58:32,884 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:58:32,884 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:58:32,940 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-03:58:32,944 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-03:58:32,954 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-03:58:34,807 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-03:58:34,808 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-03:58:34,808 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-03:58:34,810 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node\n'
b'200626-03:58:34,891 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject08_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/3d2e1987a1f86b8badee7cfdec81d54c1554785d/ds_betaseries_file".\n'
b'200626-03:58:34,900 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/3d2e1987a1f86b8badee7cfdec81d54c1554785d/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-03:58:34,903 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:58:34,969 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-03:58:34,971 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/3d2e1987a1f86b8badee7cfdec81d54c1554785d/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-03:58:34,974 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:58:35,37 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-03:58:35,39 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/3d2e1987a1f86b8badee7cfdec81d54c1554785d/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-03:58:35,41 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-03:58:35,108 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-03:58:35,111 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject08_wf.ds_betaseries_file".\n'
b'200626-03:58:36,815 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject08_wf.ds_betaseries_file).\n'
b'200626-03:58:36,817 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-04:20:26,218 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node".\n'
b'200626-04:20:28,107 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject08_wf.betaseries_wf.betaseries_node).\n'
b'200626-04:20:28,109 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-04:20:30,116 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-04:20:30,197 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/4d51d90309303ab6edf2de2446a02330e4437ae3/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-04:20:30,198 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/4d51d90309303ab6edf2de2446a02330e4437ae3/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-04:20:30,198 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/4d51d90309303ab6edf2de2446a02330e4437ae3/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-04:20:30,202 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:20:30,202 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:20:30,202 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:20:30,262 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-04:20:30,265 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-04:20:30,266 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-04:20:32,123 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-04:20:32,124 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-04:20:32,124 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-04:20:32,126 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-04:20:32,207 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject08_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/4d51d90309303ab6edf2de2446a02330e4437ae3/ds_betaseries_file".\n'
b'200626-04:20:32,215 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/4d51d90309303ab6edf2de2446a02330e4437ae3/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-04:20:32,218 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:20:32,279 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-04:20:32,280 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/4d51d90309303ab6edf2de2446a02330e4437ae3/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-04:20:32,283 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:20:32,346 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-04:20:32,348 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject08_wf/4d51d90309303ab6edf2de2446a02330e4437ae3/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-04:20:32,351 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:20:32,566 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-04:20:32,569 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject08_wf.ds_betaseries_file".\n'
b'200626-04:20:34,131 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject08_wf.ds_betaseries_file).\n'
b'200626-04:20:34,133 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-09 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-04:20:47,396 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-04:20:47,764 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-04:20:47,775 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-04:20:47,778 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-04:20:48,50 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/betaseries_wf/da10012dd3509357401220a9723ec8da7c75287b/betaseries_node".\n'
b'200626-04:20:48,56 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-04:20:48,57 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/betaseries_wf/f0e2c11dac5da820f6fd38b11077ddf21ae12782/betaseries_node".\n'
b'200626-04:20:48,66 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-04:20:49,786 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 24 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 23 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-04:59:55,617 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node".\n'
b'200626-04:59:57,259 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node).\n'
b'200626-04:59:57,261 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node\n'
b'200626-04:59:59,268 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node\n'
b'200626-04:59:59,349 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/da10012dd3509357401220a9723ec8da7c75287b/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-04:59:59,353 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/da10012dd3509357401220a9723ec8da7c75287b/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-04:59:59,355 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:59:59,360 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/da10012dd3509357401220a9723ec8da7c75287b/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-04:59:59,362 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:59:59,365 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-04:59:59,417 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-04:59:59,418 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-04:59:59,467 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-05:00:01,275 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-05:00:01,275 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-05:00:01,276 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-05:00:01,277 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node\n'
b'200626-05:00:01,358 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject09_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/da10012dd3509357401220a9723ec8da7c75287b/ds_betaseries_file".\n'
b'200626-05:00:01,368 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/da10012dd3509357401220a9723ec8da7c75287b/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-05:00:01,371 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:00:01,440 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-05:00:01,441 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/da10012dd3509357401220a9723ec8da7c75287b/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-05:00:01,444 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:00:01,503 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-05:00:01,504 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/da10012dd3509357401220a9723ec8da7c75287b/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-05:00:01,507 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:00:01,729 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-05:00:01,732 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject09_wf.ds_betaseries_file".\n'
b'200626-05:00:03,283 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject09_wf.ds_betaseries_file).\n'
b'200626-05:00:03,285 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-05:21:02,449 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node".\n'
b'200626-05:21:04,355 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject09_wf.betaseries_wf.betaseries_node).\n'
b'200626-05:21:04,357 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-05:21:06,364 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-05:21:06,448 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/f0e2c11dac5da820f6fd38b11077ddf21ae12782/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-05:21:06,449 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/f0e2c11dac5da820f6fd38b11077ddf21ae12782/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-05:21:06,454 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:21:06,453 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/f0e2c11dac5da820f6fd38b11077ddf21ae12782/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-05:21:06,454 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:21:06,460 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:21:06,513 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-05:21:06,516 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-05:21:06,519 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-05:21:08,371 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-05:21:08,372 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-05:21:08,372 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-05:21:08,374 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-05:21:08,451 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject09_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/f0e2c11dac5da820f6fd38b11077ddf21ae12782/ds_betaseries_file".\n'
b'200626-05:21:08,461 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/f0e2c11dac5da820f6fd38b11077ddf21ae12782/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-05:21:08,465 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:21:08,535 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-05:21:08,537 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/f0e2c11dac5da820f6fd38b11077ddf21ae12782/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-05:21:08,539 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:21:08,602 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-05:21:08,603 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject09_wf/f0e2c11dac5da820f6fd38b11077ddf21ae12782/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-05:21:08,606 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-05:21:08,669 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-05:21:09,566 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject09_wf.ds_betaseries_file".\n'
b'200626-05:21:10,379 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject09_wf.ds_betaseries_file).\n'
b'200626-05:21:10,381 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-10 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-05:21:22,595 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-05:21:22,969 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-05:21:22,982 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-05:21:22,986 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-05:21:23,226 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/betaseries_wf/9a2c2ad3f8cdf397de104fae52b2189d917eccaa/betaseries_node".\n'
b'200626-05:21:23,230 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/betaseries_wf/2ed1ce320ad35b42894b0bbb60f743552040d87b/betaseries_node".\n'
b'200626-05:21:23,233 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-05:21:23,236 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-05:21:24,993 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 69 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-06:31:46,330 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node".\n'
b'200626-06:31:48,39 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node).\n'
b'200626-06:31:48,41 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node\n'
b'200626-06:31:50,48 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node\n'
b'200626-06:31:50,130 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/2ed1ce320ad35b42894b0bbb60f743552040d87b/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-06:31:50,130 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/2ed1ce320ad35b42894b0bbb60f743552040d87b/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-06:31:50,131 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/2ed1ce320ad35b42894b0bbb60f743552040d87b/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-06:31:50,135 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:31:50,135 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:31:50,135 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:31:50,199 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-06:31:50,207 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-06:31:50,208 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-06:31:52,55 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-06:31:52,56 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-06:31:52,56 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-06:31:52,59 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node\n'
b'200626-06:31:52,146 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject10_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/2ed1ce320ad35b42894b0bbb60f743552040d87b/ds_betaseries_file".\n'
b'200626-06:31:52,153 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/2ed1ce320ad35b42894b0bbb60f743552040d87b/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-06:31:52,156 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:31:52,229 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-06:31:52,232 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/2ed1ce320ad35b42894b0bbb60f743552040d87b/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-06:31:52,235 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:31:52,302 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-06:31:52,303 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/2ed1ce320ad35b42894b0bbb60f743552040d87b/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-06:31:52,306 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:31:52,367 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-06:31:52,370 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject10_wf.ds_betaseries_file".\n'
b'200626-06:31:54,64 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject10_wf.ds_betaseries_file).\n'
b'200626-06:31:54,66 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node\n'
b'200626-06:32:54,48 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node".\n'
b'200626-06:32:54,303 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject10_wf.betaseries_wf.betaseries_node).\n'
b'200626-06:32:54,305 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-06:32:56,312 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-06:32:56,386 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/9a2c2ad3f8cdf397de104fae52b2189d917eccaa/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-06:32:56,387 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/9a2c2ad3f8cdf397de104fae52b2189d917eccaa/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-06:32:56,389 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/9a2c2ad3f8cdf397de104fae52b2189d917eccaa/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-06:32:56,456 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:32:56,456 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:32:56,457 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:32:56,520 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-06:32:56,521 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-06:32:56,521 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-06:32:58,319 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-06:32:58,320 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-06:32:58,320 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-06:32:58,322 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-06:32:58,398 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject10_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/9a2c2ad3f8cdf397de104fae52b2189d917eccaa/ds_betaseries_file".\n'
b'200626-06:32:58,405 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/9a2c2ad3f8cdf397de104fae52b2189d917eccaa/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-06:32:58,409 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:32:58,475 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-06:32:58,477 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/9a2c2ad3f8cdf397de104fae52b2189d917eccaa/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-06:32:58,480 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:32:58,629 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-06:32:58,630 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject10_wf/9a2c2ad3f8cdf397de104fae52b2189d917eccaa/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-06:32:58,633 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-06:32:58,696 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-06:32:58,699 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject10_wf.ds_betaseries_file".\n'
b'200626-06:33:00,327 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject10_wf.ds_betaseries_file).\n'
b'200626-06:33:00,330 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-11 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-06:33:12,592 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-06:33:12,952 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-06:33:12,965 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-06:33:12,968 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-06:33:13,208 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/betaseries_wf/8b7e28c28a34109cc6a3797a24334bdd6a808f35/betaseries_node".\n'
b'200626-06:33:13,208 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/betaseries_wf/1bc3dd8921900b90745f9162b1ac7046667a457a/betaseries_node".\n'
b'200626-06:33:13,213 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-06:33:13,214 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-06:33:14,977 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 63 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-07:50:36,713 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node".\n'
b'200626-07:50:37,755 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node).\n'
b'200626-07:50:37,757 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node\n'
b'200626-07:50:39,764 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node\n'
b'200626-07:50:39,843 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/1bc3dd8921900b90745f9162b1ac7046667a457a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-07:50:39,844 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/1bc3dd8921900b90745f9162b1ac7046667a457a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-07:50:39,845 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/1bc3dd8921900b90745f9162b1ac7046667a457a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-07:50:39,848 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:50:39,848 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:50:39,849 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:50:39,906 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-07:50:39,910 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-07:50:39,920 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-07:50:41,771 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-07:50:41,772 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-07:50:41,772 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-07:50:41,774 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node\n'
b'200626-07:50:41,852 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject11_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/1bc3dd8921900b90745f9162b1ac7046667a457a/ds_betaseries_file".\n'
b'200626-07:50:41,859 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/1bc3dd8921900b90745f9162b1ac7046667a457a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-07:50:41,862 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:50:41,931 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-07:50:41,933 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/1bc3dd8921900b90745f9162b1ac7046667a457a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-07:50:41,936 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:50:42,211 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-07:50:42,212 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/1bc3dd8921900b90745f9162b1ac7046667a457a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-07:50:42,214 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:50:42,278 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-07:50:42,281 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject11_wf.ds_betaseries_file".\n'
b'200626-07:50:43,779 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject11_wf.ds_betaseries_file).\n'
b'200626-07:50:43,780 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node\n'
b'200626-07:51:24,584 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node".\n'
b'200626-07:51:25,951 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject11_wf.betaseries_wf.betaseries_node).\n'
b'200626-07:51:25,953 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-07:51:27,960 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-07:51:28,38 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/8b7e28c28a34109cc6a3797a24334bdd6a808f35/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-07:51:28,43 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:51:28,43 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/8b7e28c28a34109cc6a3797a24334bdd6a808f35/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-07:51:28,43 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/8b7e28c28a34109cc6a3797a24334bdd6a808f35/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-07:51:28,47 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:51:28,47 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:51:28,95 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-07:51:28,107 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-07:51:28,114 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-07:51:29,967 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-07:51:29,968 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-07:51:29,968 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-07:51:29,970 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-07:51:30,51 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject11_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/8b7e28c28a34109cc6a3797a24334bdd6a808f35/ds_betaseries_file".\n'
b'200626-07:51:30,59 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/8b7e28c28a34109cc6a3797a24334bdd6a808f35/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-07:51:30,62 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:51:30,135 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-07:51:30,137 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/8b7e28c28a34109cc6a3797a24334bdd6a808f35/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-07:51:30,139 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:51:30,205 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-07:51:30,207 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject11_wf/8b7e28c28a34109cc6a3797a24334bdd6a808f35/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-07:51:30,209 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-07:51:30,264 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-07:51:30,267 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject11_wf.ds_betaseries_file".\n'
b'200626-07:51:31,975 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject11_wf.ds_betaseries_file).\n'
b'200626-07:51:31,977 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-12 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-07:51:44,81 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-07:51:44,460 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-07:51:44,471 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-07:51:44,474 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-07:51:44,736 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/betaseries_wf/6deda2f3368e7a4c03b901590cb532643e62c5b4/betaseries_node".\n'
b'200626-07:51:44,736 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/betaseries_wf/3513898370e9b0674769ba308a93636017349132/betaseries_node".\n'
b'200626-07:51:44,742 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-07:51:44,742 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-07:51:46,480 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-08:51:50,298 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node".\n'
b'200626-08:51:50,899 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node).\n'
b'200626-08:51:50,902 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node\n'
b'200626-08:51:52,908 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node\n'
b'200626-08:51:52,986 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/6deda2f3368e7a4c03b901590cb532643e62c5b4/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-08:51:52,990 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:51:52,992 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/6deda2f3368e7a4c03b901590cb532643e62c5b4/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-08:51:52,993 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/6deda2f3368e7a4c03b901590cb532643e62c5b4/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-08:51:52,996 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:51:52,997 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:51:53,43 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-08:51:53,53 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-08:51:53,54 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-08:51:54,915 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-08:51:54,916 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-08:51:54,916 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-08:51:54,918 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node\n'
b'200626-08:51:55,0 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject12_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/6deda2f3368e7a4c03b901590cb532643e62c5b4/ds_betaseries_file".\n'
b'200626-08:51:55,8 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/6deda2f3368e7a4c03b901590cb532643e62c5b4/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-08:51:55,339 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:51:55,400 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-08:51:55,402 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/6deda2f3368e7a4c03b901590cb532643e62c5b4/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-08:51:55,405 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:51:55,712 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-08:51:55,713 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/6deda2f3368e7a4c03b901590cb532643e62c5b4/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-08:51:55,716 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:51:55,773 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-08:51:55,776 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject12_wf.ds_betaseries_file".\n'
b'200626-08:51:56,923 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject12_wf.ds_betaseries_file).\n'
b'200626-08:51:56,925 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node\n'
b'200626-08:52:38,473 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node".\n'
b'200626-08:52:39,91 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject12_wf.betaseries_wf.betaseries_node).\n'
b'200626-08:52:39,93 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-08:52:41,99 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-08:52:41,188 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/3513898370e9b0674769ba308a93636017349132/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-08:52:41,189 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/3513898370e9b0674769ba308a93636017349132/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-08:52:41,191 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/3513898370e9b0674769ba308a93636017349132/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-08:52:41,193 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:52:41,193 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:52:41,195 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:52:41,244 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-08:52:41,251 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-08:52:41,260 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-08:52:43,106 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-08:52:43,107 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-08:52:43,108 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-08:52:43,109 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-08:52:43,193 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject12_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/3513898370e9b0674769ba308a93636017349132/ds_betaseries_file".\n'
b'200626-08:52:43,200 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/3513898370e9b0674769ba308a93636017349132/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-08:52:43,203 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:52:43,259 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-08:52:43,261 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/3513898370e9b0674769ba308a93636017349132/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-08:52:43,264 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:52:43,538 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-08:52:43,540 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject12_wf/3513898370e9b0674769ba308a93636017349132/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-08:52:43,543 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-08:52:43,599 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-08:52:43,602 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject12_wf.ds_betaseries_file".\n'
b'200626-08:52:45,117 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject12_wf.ds_betaseries_file).\n'
b'200626-08:52:45,119 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-13 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-08:52:58,355 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-08:52:58,729 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-08:52:58,742 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-08:52:58,746 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-08:52:58,977 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/betaseries_wf/a85630755c272b966edf43f6ad3c64e0f148c629/betaseries_node".\n'
b'200626-08:52:58,978 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/betaseries_wf/3b043dbee7157234e7b930a99e13c46317bffa54/betaseries_node".\n'
b'200626-08:52:58,983 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-08:52:58,984 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-08:53:00,752 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-09:55:10,691 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node".\n'
b'200626-09:55:11,787 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node).\n'
b'200626-09:55:11,789 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node\n'
b'200626-09:55:13,796 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node\n'
b'200626-09:55:13,935 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/3b043dbee7157234e7b930a99e13c46317bffa54/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-09:55:13,939 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/3b043dbee7157234e7b930a99e13c46317bffa54/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-09:55:13,940 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/3b043dbee7157234e7b930a99e13c46317bffa54/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-09:55:13,944 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:55:13,946 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:55:13,948 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:55:14,13 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-09:55:14,21 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-09:55:14,22 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-09:55:15,804 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-09:55:15,805 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-09:55:15,805 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-09:55:15,807 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node\n'
b'200626-09:55:15,955 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject13_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/3b043dbee7157234e7b930a99e13c46317bffa54/ds_betaseries_file".\n'
b'200626-09:55:15,966 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/3b043dbee7157234e7b930a99e13c46317bffa54/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-09:55:15,972 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:55:16,52 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-09:55:16,55 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/3b043dbee7157234e7b930a99e13c46317bffa54/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-09:55:16,58 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:55:16,131 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-09:55:16,133 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/3b043dbee7157234e7b930a99e13c46317bffa54/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-09:55:16,137 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:55:16,481 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-09:55:16,484 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject13_wf.ds_betaseries_file".\n'
b'200626-09:55:17,811 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject13_wf.ds_betaseries_file).\n'
b'200626-09:55:17,814 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-09:56:57,391 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node".\n'
b'200626-09:56:58,211 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject13_wf.betaseries_wf.betaseries_node).\n'
b'200626-09:56:58,213 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-09:57:00,220 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-09:57:00,301 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/a85630755c272b966edf43f6ad3c64e0f148c629/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-09:57:00,303 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/a85630755c272b966edf43f6ad3c64e0f148c629/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-09:57:00,304 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/a85630755c272b966edf43f6ad3c64e0f148c629/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-09:57:00,306 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:57:00,307 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:57:00,308 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:57:00,374 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-09:57:00,375 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-09:57:00,377 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-09:57:02,227 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-09:57:02,227 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-09:57:02,228 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-09:57:02,230 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-09:57:02,307 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject13_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/a85630755c272b966edf43f6ad3c64e0f148c629/ds_betaseries_file".\n'
b'200626-09:57:02,314 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/a85630755c272b966edf43f6ad3c64e0f148c629/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-09:57:02,318 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:57:02,393 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-09:57:02,395 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/a85630755c272b966edf43f6ad3c64e0f148c629/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-09:57:02,398 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:57:02,466 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-09:57:02,467 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject13_wf/a85630755c272b966edf43f6ad3c64e0f148c629/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-09:57:02,470 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-09:57:02,677 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-09:57:02,679 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject13_wf.ds_betaseries_file".\n'
b'200626-09:57:04,235 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject13_wf.ds_betaseries_file).\n'
b'200626-09:57:04,237 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-14 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-09:57:16,658 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-09:57:17,27 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-09:57:17,40 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-09:57:17,44 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-09:57:17,254 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/betaseries_wf/8415f3c56f05bbd6f0fc3ef57cff3610f1929233/betaseries_node".\n'
b'200626-09:57:17,255 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/betaseries_wf/98e427f9888cca23ec5d8a8a06532cbd6f9e1b1d/betaseries_node".\n'
b'200626-09:57:17,260 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-09:57:17,261 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-09:57:19,53 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-10:59:58,45 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node".\n'
b'200626-10:59:58,315 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node).\n'
b'200626-10:59:58,318 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node\n'
b'200626-11:00:00,324 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node\n'
b'200626-11:00:00,409 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/8415f3c56f05bbd6f0fc3ef57cff3610f1929233/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-11:00:00,412 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/8415f3c56f05bbd6f0fc3ef57cff3610f1929233/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-11:00:00,413 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/8415f3c56f05bbd6f0fc3ef57cff3610f1929233/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-11:00:00,414 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:00,417 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:00,418 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:00,479 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-11:00:00,481 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-11:00:00,483 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-11:00:02,331 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-11:00:02,331 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-11:00:02,332 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-11:00:02,333 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node\n'
b'200626-11:00:02,414 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject14_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/8415f3c56f05bbd6f0fc3ef57cff3610f1929233/ds_betaseries_file".\n'
b'200626-11:00:02,423 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/8415f3c56f05bbd6f0fc3ef57cff3610f1929233/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-11:00:02,426 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:02,498 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-11:00:02,500 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/8415f3c56f05bbd6f0fc3ef57cff3610f1929233/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-11:00:02,503 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:02,568 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-11:00:02,569 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/8415f3c56f05bbd6f0fc3ef57cff3610f1929233/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-11:00:02,572 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:02,928 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-11:00:02,931 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject14_wf.ds_betaseries_file".\n'
b'200626-11:00:04,339 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject14_wf.ds_betaseries_file).\n'
b'200626-11:00:04,341 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node\n'
b'200626-11:00:30,156 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node".\n'
b'200626-11:00:30,443 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject14_wf.betaseries_wf.betaseries_node).\n'
b'200626-11:00:30,445 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-11:00:32,452 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-11:00:32,531 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/98e427f9888cca23ec5d8a8a06532cbd6f9e1b1d/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-11:00:32,536 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/98e427f9888cca23ec5d8a8a06532cbd6f9e1b1d/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-11:00:32,537 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/98e427f9888cca23ec5d8a8a06532cbd6f9e1b1d/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-11:00:32,540 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:32,541 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:32,544 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:32,593 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-11:00:32,597 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-11:00:32,603 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-11:00:34,461 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-11:00:34,461 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-11:00:34,462 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-11:00:34,463 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-11:00:34,540 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject14_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/98e427f9888cca23ec5d8a8a06532cbd6f9e1b1d/ds_betaseries_file".\n'
b'200626-11:00:34,547 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/98e427f9888cca23ec5d8a8a06532cbd6f9e1b1d/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-11:00:34,550 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:34,613 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-11:00:34,615 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/98e427f9888cca23ec5d8a8a06532cbd6f9e1b1d/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-11:00:34,618 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:34,673 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-11:00:34,674 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject14_wf/98e427f9888cca23ec5d8a8a06532cbd6f9e1b1d/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-11:00:34,676 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-11:00:34,904 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-11:00:34,908 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject14_wf.ds_betaseries_file".\n'
b'200626-11:00:36,467 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject14_wf.ds_betaseries_file).\n'
b'200626-11:00:36,469 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-15 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-11:00:48,542 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-11:00:48,876 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-11:00:48,885 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-11:00:48,889 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-11:00:49,109 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/betaseries_wf/b6cc736869378f0175424ba595b61373eb204e0a/betaseries_node".\n'
b'200626-11:00:49,110 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/betaseries_wf/a774f22e9c6c9bfabcc4942b0fe8e905aa136059/betaseries_node".\n'
b'200626-11:00:49,165 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-11:00:49,165 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-11:00:50,897 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-12:06:07,558 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node".\n'
b'200626-12:06:08,607 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node).\n'
b'200626-12:06:08,610 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node\n'
b'200626-12:06:10,616 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node\n'
b'200626-12:06:10,697 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/a774f22e9c6c9bfabcc4942b0fe8e905aa136059/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-12:06:10,698 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/a774f22e9c6c9bfabcc4942b0fe8e905aa136059/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-12:06:10,702 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:06:10,704 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/a774f22e9c6c9bfabcc4942b0fe8e905aa136059/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-12:06:10,709 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:06:10,709 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:06:10,788 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-12:06:10,791 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-12:06:10,793 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-12:06:12,623 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-12:06:12,624 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-12:06:12,624 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-12:06:12,626 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node\n'
b'200626-12:06:12,710 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject15_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/a774f22e9c6c9bfabcc4942b0fe8e905aa136059/ds_betaseries_file".\n'
b'200626-12:06:12,720 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/a774f22e9c6c9bfabcc4942b0fe8e905aa136059/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-12:06:12,723 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:06:12,796 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-12:06:12,799 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/a774f22e9c6c9bfabcc4942b0fe8e905aa136059/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-12:06:12,802 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:06:12,865 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-12:06:12,866 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/a774f22e9c6c9bfabcc4942b0fe8e905aa136059/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-12:06:12,869 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:06:12,929 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-12:06:12,932 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject15_wf.ds_betaseries_file".\n'
b'200626-12:06:14,631 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject15_wf.ds_betaseries_file).\n'
b'200626-12:06:14,633 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 66 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-12:07:24,596 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node".\n'
b'200626-12:07:24,907 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject15_wf.betaseries_wf.betaseries_node).\n'
b'200626-12:07:24,909 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-12:07:26,917 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-12:07:26,992 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/b6cc736869378f0175424ba595b61373eb204e0a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-12:07:26,994 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/b6cc736869378f0175424ba595b61373eb204e0a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-12:07:26,995 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/b6cc736869378f0175424ba595b61373eb204e0a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-12:07:26,998 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:07:27,3 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:07:27,4 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:07:27,57 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-12:07:27,61 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-12:07:27,73 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-12:07:28,923 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-12:07:28,924 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-12:07:28,924 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-12:07:28,926 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-12:07:29,9 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject15_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/b6cc736869378f0175424ba595b61373eb204e0a/ds_betaseries_file".\n'
b'200626-12:07:29,17 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/b6cc736869378f0175424ba595b61373eb204e0a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-12:07:29,21 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:07:29,86 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-12:07:29,88 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/b6cc736869378f0175424ba595b61373eb204e0a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-12:07:29,91 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:07:29,267 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-12:07:29,268 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject15_wf/b6cc736869378f0175424ba595b61373eb204e0a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-12:07:29,270 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-12:07:29,329 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-12:07:30,931 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject15_wf.ds_betaseries_file\n'
b'200626-12:07:31,512 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject15_wf.ds_betaseries_file".\n'
b'200626-12:07:32,939 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject15_wf.ds_betaseries_file).\n'
b'200626-12:07:32,941 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-16 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-12:07:47,953 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-12:07:48,646 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-12:07:48,663 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-12:07:48,667 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-12:07:48,930 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/betaseries_wf/2ab28aea303ad2ab0d680ac70f3d33dca76d0517/betaseries_node".\n'
b'200626-12:07:48,931 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/betaseries_wf/1c0721330be7c1930cce26d214c5fe5fde73fc61/betaseries_node".\n'
b'200626-12:07:48,935 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-12:07:48,936 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-12:07:50,676 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-13:07:12,617 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node".\n'
b'200626-13:07:13,299 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node).\n'
b'200626-13:07:13,301 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node\n'
b'200626-13:07:15,308 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node\n'
b'200626-13:07:15,387 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/1c0721330be7c1930cce26d214c5fe5fde73fc61/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-13:07:15,394 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:07:15,394 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/1c0721330be7c1930cce26d214c5fe5fde73fc61/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-13:07:15,398 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:07:15,402 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/1c0721330be7c1930cce26d214c5fe5fde73fc61/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-13:07:15,405 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:07:15,457 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-13:07:15,464 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-13:07:15,467 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-13:07:17,317 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-13:07:17,317 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-13:07:17,318 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-13:07:17,320 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node\n'
b'200626-13:07:17,406 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject16_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/1c0721330be7c1930cce26d214c5fe5fde73fc61/ds_betaseries_file".\n'
b'200626-13:07:17,413 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/1c0721330be7c1930cce26d214c5fe5fde73fc61/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-13:07:17,416 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:07:17,488 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-13:07:17,489 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/1c0721330be7c1930cce26d214c5fe5fde73fc61/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-13:07:17,493 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:07:17,562 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-13:07:17,563 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/1c0721330be7c1930cce26d214c5fe5fde73fc61/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-13:07:17,566 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:07:17,916 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-13:07:17,919 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject16_wf.ds_betaseries_file".\n'
b'200626-13:07:19,323 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject16_wf.ds_betaseries_file).\n'
b'200626-13:07:19,325 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-13:08:46,999 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node".\n'
b'200626-13:08:47,671 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject16_wf.betaseries_wf.betaseries_node).\n'
b'200626-13:08:47,673 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-13:08:49,680 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-13:08:49,759 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/2ab28aea303ad2ab0d680ac70f3d33dca76d0517/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-13:08:49,762 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/2ab28aea303ad2ab0d680ac70f3d33dca76d0517/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-13:08:49,763 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:08:49,768 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/2ab28aea303ad2ab0d680ac70f3d33dca76d0517/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-13:08:49,772 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:08:49,774 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:08:49,826 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-13:08:49,858 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-13:08:49,858 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-13:08:51,687 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-13:08:51,688 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-13:08:51,688 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-13:08:51,689 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-13:08:51,763 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject16_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/2ab28aea303ad2ab0d680ac70f3d33dca76d0517/ds_betaseries_file".\n'
b'200626-13:08:51,772 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/2ab28aea303ad2ab0d680ac70f3d33dca76d0517/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-13:08:51,776 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:08:51,851 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-13:08:51,853 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/2ab28aea303ad2ab0d680ac70f3d33dca76d0517/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-13:08:51,856 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:08:51,925 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-13:08:51,927 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject16_wf/2ab28aea303ad2ab0d680ac70f3d33dca76d0517/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-13:08:51,930 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-13:08:52,277 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-13:08:52,280 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject16_wf.ds_betaseries_file".\n'
b'200626-13:08:53,695 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject16_wf.ds_betaseries_file).\n'
b'200626-13:08:53,697 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-17 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-13:09:05,727 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-13:09:06,115 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-13:09:06,124 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-13:09:06,127 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-13:09:06,377 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/betaseries_wf/bb91f9b860de5af9298d262f7889ad2dbf32d303/betaseries_node".\n'
b'200626-13:09:06,379 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/betaseries_wf/4e0400b9030f7008295b30adf21bc14b4b5e150c/betaseries_node".\n'
b'200626-13:09:06,383 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-13:09:06,384 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-13:09:08,138 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 22 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-14:00:16,646 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node".\n'
b'200626-14:00:18,455 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node).\n'
b'200626-14:00:18,457 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node\n'
b'200626-14:00:20,464 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node\n'
b'200626-14:00:20,553 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/bb91f9b860de5af9298d262f7889ad2dbf32d303/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-14:00:20,556 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/bb91f9b860de5af9298d262f7889ad2dbf32d303/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-14:00:20,557 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:20,559 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/bb91f9b860de5af9298d262f7889ad2dbf32d303/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-14:00:20,563 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:20,563 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:20,615 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-14:00:20,622 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-14:00:20,625 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-14:00:22,471 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-14:00:22,472 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-14:00:22,473 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-14:00:22,476 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node\n'
b'200626-14:00:22,568 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject17_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/bb91f9b860de5af9298d262f7889ad2dbf32d303/ds_betaseries_file".\n'
b'200626-14:00:22,576 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/bb91f9b860de5af9298d262f7889ad2dbf32d303/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-14:00:22,579 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:22,707 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-14:00:22,709 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/bb91f9b860de5af9298d262f7889ad2dbf32d303/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-14:00:22,712 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:22,770 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-14:00:22,772 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/bb91f9b860de5af9298d262f7889ad2dbf32d303/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-14:00:22,774 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:22,831 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-14:00:24,481 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject17_wf.ds_betaseries_file\n'
b' * nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node\n'
b'200626-14:00:24,547 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject17_wf.ds_betaseries_file".\n'
b'200626-14:00:26,487 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject17_wf.ds_betaseries_file).\n'
b'200626-14:00:26,489 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node\n'
b'200626-14:00:29,553 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node".\n'
b'200626-14:00:30,503 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject17_wf.betaseries_wf.betaseries_node).\n'
b'200626-14:00:30,505 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-14:00:32,511 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-14:00:32,592 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/4e0400b9030f7008295b30adf21bc14b4b5e150c/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-14:00:32,595 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/4e0400b9030f7008295b30adf21bc14b4b5e150c/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-14:00:32,595 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/4e0400b9030f7008295b30adf21bc14b4b5e150c/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-14:00:32,596 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:32,598 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:32,599 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:32,657 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-14:00:32,662 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-14:00:32,663 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-14:00:34,519 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-14:00:34,520 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-14:00:34,520 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-14:00:34,522 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-14:00:34,602 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject17_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/4e0400b9030f7008295b30adf21bc14b4b5e150c/ds_betaseries_file".\n'
b'200626-14:00:34,610 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/4e0400b9030f7008295b30adf21bc14b4b5e150c/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-14:00:34,613 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:34,680 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-14:00:34,682 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/4e0400b9030f7008295b30adf21bc14b4b5e150c/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-14:00:34,684 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:34,741 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-14:00:34,742 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject17_wf/4e0400b9030f7008295b30adf21bc14b4b5e150c/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-14:00:34,745 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:00:34,924 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-14:00:35,773 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject17_wf.ds_betaseries_file".\n'
b'200626-14:00:36,527 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject17_wf.ds_betaseries_file).\n'
b'200626-14:00:36,530 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-18 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-14:00:48,479 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-14:00:48,804 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-14:00:48,813 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-14:00:48,816 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-14:00:49,91 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/betaseries_wf/5ec991d8b37f2a9962a937e4be55c32af7a5c909/betaseries_node".\n'
b'200626-14:00:49,93 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/betaseries_wf/bd7d8f590e79b04a77d42cbc710814d1cdbe2cac/betaseries_node".\n'
b'200626-14:00:49,99 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-14:00:49,99 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-14:00:50,826 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 26 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-14:58:24,776 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node".\n'
b'200626-14:58:26,659 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node).\n'
b'200626-14:58:26,661 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node\n'
b'200626-14:58:28,668 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node\n'
b'200626-14:58:28,749 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/bd7d8f590e79b04a77d42cbc710814d1cdbe2cac/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-14:58:28,752 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/bd7d8f590e79b04a77d42cbc710814d1cdbe2cac/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-14:58:28,753 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:58:28,754 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/bd7d8f590e79b04a77d42cbc710814d1cdbe2cac/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-14:58:28,756 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:58:28,758 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:58:28,819 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-14:58:28,821 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-14:58:28,823 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-14:58:30,677 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-14:58:30,678 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-14:58:30,679 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-14:58:30,681 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node\n'
b'200626-14:58:30,747 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject18_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/bd7d8f590e79b04a77d42cbc710814d1cdbe2cac/ds_betaseries_file".\n'
b'200626-14:58:30,754 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/bd7d8f590e79b04a77d42cbc710814d1cdbe2cac/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-14:58:30,756 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:58:30,964 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-14:58:30,966 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/bd7d8f590e79b04a77d42cbc710814d1cdbe2cac/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-14:58:30,969 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:58:31,179 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-14:58:31,180 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/bd7d8f590e79b04a77d42cbc710814d1cdbe2cac/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-14:58:31,183 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:58:31,241 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-14:58:31,244 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject18_wf.ds_betaseries_file".\n'
b'200626-14:58:32,683 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject18_wf.ds_betaseries_file).\n'
b'200626-14:58:32,685 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node\n'
b'200626-14:59:04,486 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node".\n'
b'200626-14:59:04,810 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject18_wf.betaseries_wf.betaseries_node).\n'
b'200626-14:59:04,812 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-14:59:06,820 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-14:59:06,899 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/5ec991d8b37f2a9962a937e4be55c32af7a5c909/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-14:59:06,900 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/5ec991d8b37f2a9962a937e4be55c32af7a5c909/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-14:59:06,901 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/5ec991d8b37f2a9962a937e4be55c32af7a5c909/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-14:59:06,904 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:59:06,904 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:59:06,905 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:59:06,958 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-14:59:06,964 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-14:59:06,966 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-14:59:08,827 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-14:59:08,828 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-14:59:08,828 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-14:59:08,829 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-14:59:08,911 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject18_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/5ec991d8b37f2a9962a937e4be55c32af7a5c909/ds_betaseries_file".\n'
b'200626-14:59:08,921 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/5ec991d8b37f2a9962a937e4be55c32af7a5c909/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-14:59:08,925 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:59:08,993 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-14:59:08,995 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/5ec991d8b37f2a9962a937e4be55c32af7a5c909/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-14:59:08,998 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:59:09,59 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-14:59:09,60 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject18_wf/5ec991d8b37f2a9962a937e4be55c32af7a5c909/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-14:59:09,64 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-14:59:09,125 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-14:59:09,129 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject18_wf.ds_betaseries_file".\n'
b'200626-14:59:10,835 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject18_wf.ds_betaseries_file).\n'
b'200626-14:59:10,836 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-19 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-14:59:23,5 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-14:59:23,354 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-14:59:23,365 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-14:59:23,369 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-14:59:23,638 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/betaseries_wf/c664b5a3b5da0c4ed2f0c5969f587c1640684c84/betaseries_node".\n'
b'200626-14:59:23,641 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/betaseries_wf/8801cfc88ac4ffe5d9f98fa2cc3b4d4fcd7f0cda/betaseries_node".\n'
b'200626-14:59:23,646 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-14:59:23,648 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-14:59:25,377 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 28 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 25 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-15:57:25,645 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node".\n'
b'200626-15:57:27,315 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node).\n'
b'200626-15:57:27,318 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node\n'
b'200626-15:57:29,325 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node\n'
b'200626-15:57:29,421 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/8801cfc88ac4ffe5d9f98fa2cc3b4d4fcd7f0cda/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-15:57:29,422 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/8801cfc88ac4ffe5d9f98fa2cc3b4d4fcd7f0cda/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-15:57:29,423 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/8801cfc88ac4ffe5d9f98fa2cc3b4d4fcd7f0cda/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-15:57:29,426 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:57:29,428 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:57:29,429 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:57:29,507 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-15:57:29,510 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-15:57:29,512 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-15:57:31,331 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-15:57:31,331 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-15:57:31,332 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-15:57:31,333 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node\n'
b'200626-15:57:31,416 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject19_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/8801cfc88ac4ffe5d9f98fa2cc3b4d4fcd7f0cda/ds_betaseries_file".\n'
b'200626-15:57:31,423 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/8801cfc88ac4ffe5d9f98fa2cc3b4d4fcd7f0cda/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-15:57:31,426 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:57:31,491 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-15:57:31,493 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/8801cfc88ac4ffe5d9f98fa2cc3b4d4fcd7f0cda/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-15:57:31,495 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:57:31,553 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-15:57:31,554 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/8801cfc88ac4ffe5d9f98fa2cc3b4d4fcd7f0cda/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-15:57:31,557 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:57:31,621 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-15:57:31,624 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject19_wf.ds_betaseries_file".\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-15:57:33,339 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject19_wf.ds_betaseries_file).\n'
b'200626-15:57:33,342 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node\n'
b'200626-15:58:39,352 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node".\n'
b'200626-15:58:39,591 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject19_wf.betaseries_wf.betaseries_node).\n'
b'200626-15:58:39,593 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-15:58:41,600 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-15:58:41,696 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/c664b5a3b5da0c4ed2f0c5969f587c1640684c84/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-15:58:41,697 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/c664b5a3b5da0c4ed2f0c5969f587c1640684c84/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-15:58:41,697 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/c664b5a3b5da0c4ed2f0c5969f587c1640684c84/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-15:58:41,701 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:58:41,703 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:58:41,703 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:58:41,751 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-15:58:41,776 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-15:58:41,778 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-15:58:43,607 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-15:58:43,607 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-15:58:43,608 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-15:58:43,609 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-15:58:43,707 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject19_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/c664b5a3b5da0c4ed2f0c5969f587c1640684c84/ds_betaseries_file".\n'
b'200626-15:58:43,716 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/c664b5a3b5da0c4ed2f0c5969f587c1640684c84/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-15:58:43,720 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:58:43,793 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-15:58:43,795 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/c664b5a3b5da0c4ed2f0c5969f587c1640684c84/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-15:58:43,798 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:58:44,39 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-15:58:44,40 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject19_wf/c664b5a3b5da0c4ed2f0c5969f587c1640684c84/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-15:58:44,43 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-15:58:44,105 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-15:58:45,616 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject19_wf.ds_betaseries_file\n'
b'200626-15:58:46,3 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject19_wf.ds_betaseries_file".\n'
b'200626-15:58:47,623 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject19_wf.ds_betaseries_file).\n'
b'200626-15:58:47,625 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-20 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-15:59:03,426 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-15:59:03,948 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-15:59:03,960 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-15:59:03,964 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-15:59:04,246 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/betaseries_wf/d03b96174661c5c6e3083b91053d06819b37bec0/betaseries_node".\n'
b'200626-15:59:04,248 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/betaseries_wf/a2c4a0b1c62396eabd022619d3bd18a6f6af345a/betaseries_node".\n'
b'200626-15:59:04,253 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-15:59:04,254 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-15:59:05,973 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 27 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-16:59:51,775 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node".\n'
b'200626-16:59:52,703 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node).\n'
b'200626-16:59:52,706 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node\n'
b'200626-16:59:54,711 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node\n'
b'200626-16:59:54,792 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/d03b96174661c5c6e3083b91053d06819b37bec0/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-16:59:54,792 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/d03b96174661c5c6e3083b91053d06819b37bec0/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-16:59:54,792 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/d03b96174661c5c6e3083b91053d06819b37bec0/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-16:59:54,796 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-16:59:54,796 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-16:59:54,796 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-16:59:54,925 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-16:59:54,930 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-16:59:54,930 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-16:59:56,719 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-16:59:56,720 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-16:59:56,720 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-16:59:56,722 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node\n'
b'200626-16:59:56,805 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject20_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/d03b96174661c5c6e3083b91053d06819b37bec0/ds_betaseries_file".\n'
b'200626-16:59:56,813 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/d03b96174661c5c6e3083b91053d06819b37bec0/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-16:59:56,815 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-16:59:56,884 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-16:59:56,886 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/d03b96174661c5c6e3083b91053d06819b37bec0/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-16:59:56,888 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-16:59:56,948 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-16:59:56,949 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/d03b96174661c5c6e3083b91053d06819b37bec0/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-16:59:56,952 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-16:59:57,15 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-16:59:57,19 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject20_wf.ds_betaseries_file".\n'
b'200626-16:59:58,727 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject20_wf.ds_betaseries_file).\n'
b'200626-16:59:58,729 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node\n'
b'200626-17:00:05,608 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node".\n'
b'200626-17:00:06,759 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject20_wf.betaseries_wf.betaseries_node).\n'
b'200626-17:00:06,761 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-17:00:08,768 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-17:00:08,846 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/a2c4a0b1c62396eabd022619d3bd18a6f6af345a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-17:00:08,850 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/a2c4a0b1c62396eabd022619d3bd18a6f6af345a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-17:00:08,850 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/a2c4a0b1c62396eabd022619d3bd18a6f6af345a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-17:00:08,906 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:00:08,907 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:00:08,911 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:00:08,968 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-17:00:08,974 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-17:00:08,975 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-17:00:10,775 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-17:00:10,776 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-17:00:10,776 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-17:00:10,779 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-17:00:10,859 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject20_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/a2c4a0b1c62396eabd022619d3bd18a6f6af345a/ds_betaseries_file".\n'
b'200626-17:00:10,867 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/a2c4a0b1c62396eabd022619d3bd18a6f6af345a/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-17:00:10,871 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:00:10,952 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-17:00:10,955 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/a2c4a0b1c62396eabd022619d3bd18a6f6af345a/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-17:00:10,958 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:00:11,15 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-17:00:11,17 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject20_wf/a2c4a0b1c62396eabd022619d3bd18a6f6af345a/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-17:00:11,19 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:00:11,73 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-17:00:11,76 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject20_wf.ds_betaseries_file".\n'
b'200626-17:00:12,782 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject20_wf.ds_betaseries_file).\n'
b'200626-17:00:12,785 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-21 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-17:00:27,184 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-17:00:27,596 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-17:00:27,609 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-17:00:27,612 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-17:00:27,848 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/betaseries_wf/5eb04c4da950e0730416387fab4d7a6754640980/betaseries_node".\n'
b'200626-17:00:27,851 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/betaseries_wf/d9e33f33b72476e339679e75c3b501e28af5ab09/betaseries_node".\n'
b'200626-17:00:27,854 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-17:00:27,857 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-17:00:29,621 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 31 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 29 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 30 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-17:58:15,514 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node".\n'
b'200626-17:58:15,599 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node).\n'
b'200626-17:58:15,601 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node\n'
b'200626-17:58:17,608 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node\n'
b'200626-17:58:17,693 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/d9e33f33b72476e339679e75c3b501e28af5ab09/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-17:58:17,694 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/d9e33f33b72476e339679e75c3b501e28af5ab09/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-17:58:17,698 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:58:17,698 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:58:17,700 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/d9e33f33b72476e339679e75c3b501e28af5ab09/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-17:58:17,705 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:58:17,761 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-17:58:17,769 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-17:58:17,775 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 35 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-17:58:19,615 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-17:58:19,616 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-17:58:19,616 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-17:58:19,618 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node\n'
b'200626-17:58:19,705 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject21_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/d9e33f33b72476e339679e75c3b501e28af5ab09/ds_betaseries_file".\n'
b'200626-17:58:19,714 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/d9e33f33b72476e339679e75c3b501e28af5ab09/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-17:58:19,718 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:58:19,793 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-17:58:19,794 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/d9e33f33b72476e339679e75c3b501e28af5ab09/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-17:58:19,797 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:58:20,79 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-17:58:20,81 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/d9e33f33b72476e339679e75c3b501e28af5ab09/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-17:58:20,83 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:58:20,150 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-17:58:20,152 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject21_wf.ds_betaseries_file".\n'
b'200626-17:58:21,624 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject21_wf.ds_betaseries_file).\n'
b'200626-17:58:21,626 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node\n'
b'200626-17:59:31,797 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node".\n'
b'200626-17:59:31,903 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject21_wf.betaseries_wf.betaseries_node).\n'
b'200626-17:59:31,905 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-17:59:33,912 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-17:59:33,990 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/5eb04c4da950e0730416387fab4d7a6754640980/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-17:59:33,991 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/5eb04c4da950e0730416387fab4d7a6754640980/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-17:59:33,995 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:59:33,995 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:59:33,996 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/5eb04c4da950e0730416387fab4d7a6754640980/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-17:59:34,0 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:59:34,65 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-17:59:34,75 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-17:59:34,78 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-17:59:35,920 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-17:59:35,921 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-17:59:35,921 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-17:59:35,923 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-17:59:36,4 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject21_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/5eb04c4da950e0730416387fab4d7a6754640980/ds_betaseries_file".\n'
b'200626-17:59:36,12 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/5eb04c4da950e0730416387fab4d7a6754640980/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-17:59:36,15 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:59:36,87 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-17:59:36,89 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/5eb04c4da950e0730416387fab4d7a6754640980/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-17:59:36,92 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:59:36,368 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-17:59:36,370 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject21_wf/5eb04c4da950e0730416387fab4d7a6754640980/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-17:59:36,373 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-17:59:36,441 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-17:59:36,444 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject21_wf.ds_betaseries_file".\n'
b'200626-17:59:37,927 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject21_wf.ds_betaseries_file).\n'
b'200626-17:59:37,929 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-22 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-17:59:50,77 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-17:59:50,438 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-17:59:50,448 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-17:59:50,451 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-17:59:50,656 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/betaseries_wf/037a25bfa0a029e37407f1a4a31b8160042fdb22/betaseries_node".\n'
b'200626-17:59:50,657 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/betaseries_wf/1ff01a43b915d6f7c39187040534dc12341db5f0/betaseries_node".\n'
b'200626-17:59:50,663 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-17:59:50,663 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-17:59:52,461 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 34 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 65 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 69 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 70 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 72 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 71 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 72 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 73 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 78 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 83 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 85 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 83 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 84 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 87 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 87 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 91 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 89 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 90 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 86 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 85 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 91 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 86 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 84 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 88 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 86 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 85 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 84 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 83 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 85 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 64 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 68 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 67 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 67 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 72 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 68 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 73 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 78 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 86 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 85 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 88 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 78 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 76 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 85 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 87 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 87 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 90 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 88 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 91 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 83 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 84 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 84 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 87 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 84 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 88 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 87 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 86 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 84 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 83 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 87 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 89 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 88 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 83 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 78 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 84 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 75 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 69 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 66 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 70 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 81 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 83 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 67 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 69 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 77 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 78 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 85 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 70 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 68 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 70 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 71 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 68 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 67 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 79 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 73 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 89 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 74 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 82 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 64 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 72 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 70 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 80 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-19:55:16,377 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node".\n'
b'200626-19:55:18,97 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node).\n'
b'200626-19:55:18,136 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node\n'
b'200626-19:55:20,103 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node\n'
b'200626-19:55:20,220 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/1ff01a43b915d6f7c39187040534dc12341db5f0/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-19:55:20,222 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/1ff01a43b915d6f7c39187040534dc12341db5f0/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-19:55:20,227 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/1ff01a43b915d6f7c39187040534dc12341db5f0/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-19:55:20,243 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:55:20,243 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:55:20,243 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:55:20,404 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-19:55:20,406 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-19:55:20,405 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-19:55:22,111 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-19:55:22,113 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-19:55:22,113 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-19:55:22,115 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node\n'
b'200626-19:55:22,214 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject22_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/1ff01a43b915d6f7c39187040534dc12341db5f0/ds_betaseries_file".\n'
b'200626-19:55:22,234 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/1ff01a43b915d6f7c39187040534dc12341db5f0/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-19:55:22,237 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:55:22,451 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-19:55:22,453 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/1ff01a43b915d6f7c39187040534dc12341db5f0/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-19:55:22,456 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:55:22,519 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-19:55:22,520 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/1ff01a43b915d6f7c39187040534dc12341db5f0/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-19:55:22,523 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:55:22,583 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-19:55:22,586 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject22_wf.ds_betaseries_file".\n'
b'200626-19:55:24,119 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject22_wf.ds_betaseries_file).\n'
b'200626-19:55:24,121 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node\n'
b'200626-19:56:17,862 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node".\n'
b'200626-19:56:18,339 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject22_wf.betaseries_wf.betaseries_node).\n'
b'200626-19:56:18,340 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-19:56:20,350 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-19:56:20,435 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/037a25bfa0a029e37407f1a4a31b8160042fdb22/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-19:56:20,444 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/037a25bfa0a029e37407f1a4a31b8160042fdb22/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-19:56:20,452 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:56:20,452 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/037a25bfa0a029e37407f1a4a31b8160042fdb22/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-19:56:20,461 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:56:20,476 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:56:20,524 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-19:56:20,529 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-19:56:20,544 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-19:56:22,355 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-19:56:22,356 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-19:56:22,356 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-19:56:22,358 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-19:56:22,484 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject22_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/037a25bfa0a029e37407f1a4a31b8160042fdb22/ds_betaseries_file".\n'
b'200626-19:56:22,504 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/037a25bfa0a029e37407f1a4a31b8160042fdb22/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-19:56:22,509 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:56:22,585 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-19:56:22,587 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/037a25bfa0a029e37407f1a4a31b8160042fdb22/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-19:56:22,589 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:56:22,649 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-19:56:22,650 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject22_wf/037a25bfa0a029e37407f1a4a31b8160042fdb22/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-19:56:22,652 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-19:56:22,976 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-19:56:22,980 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject22_wf.ds_betaseries_file".\n'
b'200626-19:56:24,363 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject22_wf.ds_betaseries_file).\n'
b'200626-19:56:24,366 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-23 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-19:56:46,110 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-19:56:47,180 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-19:56:47,211 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-19:56:47,232 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-19:56:47,470 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/betaseries_wf/aaa20f2784e8b6147ee0b1eecf488e960fdd84ac/betaseries_node".\n'
b'200626-19:56:47,472 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/betaseries_wf/47bb299a9dc547ecd088ee081d9af4372e95b0fd/betaseries_node".\n'
b'200626-19:56:47,479 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-19:56:47,479 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-19:56:49,241 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 32 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 37 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 39 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 33 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 38 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 36 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-21:08:58,110 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node".\n'
b'200626-21:08:58,659 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node).\n'
b'200626-21:08:58,661 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node\n'
b'200626-21:09:00,668 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node\n'
b'200626-21:09:00,746 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/aaa20f2784e8b6147ee0b1eecf488e960fdd84ac/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-21:09:00,748 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/aaa20f2784e8b6147ee0b1eecf488e960fdd84ac/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-21:09:00,749 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/aaa20f2784e8b6147ee0b1eecf488e960fdd84ac/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-21:09:00,751 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:00,753 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:00,754 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:02,676 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 4 tasks, and 0 jobs ready. Free memory (GB): 112.42/113.22, Free processors: 36/40.\n'
b' Currently running:\n'
b' * _ds_betaseries_file2\n'
b' * _ds_betaseries_file1\n'
b' * _ds_betaseries_file0\n'
b' * nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node\n'
b'200626-21:09:04,119 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-21:09:04,119 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-21:09:04,120 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-21:09:04,684 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-21:09:04,685 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-21:09:04,686 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-21:09:04,688 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node\n'
b'200626-21:09:04,785 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject23_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/aaa20f2784e8b6147ee0b1eecf488e960fdd84ac/ds_betaseries_file".\n'
b'200626-21:09:04,794 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/aaa20f2784e8b6147ee0b1eecf488e960fdd84ac/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-21:09:04,797 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:04,869 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-21:09:04,870 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/aaa20f2784e8b6147ee0b1eecf488e960fdd84ac/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-21:09:04,873 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:04,943 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-21:09:04,944 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/aaa20f2784e8b6147ee0b1eecf488e960fdd84ac/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-21:09:04,947 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:05,293 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-21:09:05,298 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject23_wf.ds_betaseries_file".\n'
b'200626-21:09:06,691 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject23_wf.ds_betaseries_file).\n'
b'200626-21:09:06,693 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node\n'
b'200626-21:09:41,359 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node".\n'
b'200626-21:09:42,839 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject23_wf.betaseries_wf.betaseries_node).\n'
b'200626-21:09:42,841 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-21:09:44,849 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-21:09:44,926 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/47bb299a9dc547ecd088ee081d9af4372e95b0fd/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-21:09:44,928 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/47bb299a9dc547ecd088ee081d9af4372e95b0fd/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-21:09:44,928 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/47bb299a9dc547ecd088ee081d9af4372e95b0fd/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-21:09:44,931 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:44,933 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:44,933 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:45,1 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-21:09:45,5 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-21:09:45,6 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-21:09:46,855 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-21:09:46,856 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-21:09:46,857 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-21:09:46,859 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-21:09:46,941 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject23_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/47bb299a9dc547ecd088ee081d9af4372e95b0fd/ds_betaseries_file".\n'
b'200626-21:09:46,949 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/47bb299a9dc547ecd088ee081d9af4372e95b0fd/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-21:09:46,952 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:47,20 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-21:09:47,22 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/47bb299a9dc547ecd088ee081d9af4372e95b0fd/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-21:09:47,25 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:47,93 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-21:09:47,94 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject23_wf/47bb299a9dc547ecd088ee081d9af4372e95b0fd/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-21:09:47,97 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-21:09:47,159 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-21:09:47,162 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject23_wf.ds_betaseries_file".\n'
b'200626-21:09:48,863 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject23_wf.ds_betaseries_file).\n'
b'200626-21:09:48,865 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
The NiBetaSeries Command looks as follows:
nibs -c rot_x rot_y rot_z trans_x trans_y trans_y --participant-label sub-24 --estimator lss --hrf-model spm -w /data/mvs/derivatives/connectivity/reults/nibetaseries/work -sp individual --sm 0.0 --description-label 'preproc' /data/mvs/ preprocessing /data/mvs/derivatives/connectivity/results/nibetaseries/ participant
b'200626-21:10:04,845 nipype.utils INFO:\n'
b'\t Running nipype version 1.4.2 (latest: 1.5.0)\n'
b'200626-21:10:05,373 nipype.workflow INFO:\n'
b"\t Workflow nibetaseries_participant_wf settings: ['check', 'execution', 'logging', 'monitoring']\n"
b'200626-21:10:05,381 nipype.workflow INFO:\n'
b'\t Running in parallel.\n'
b'200626-21:10:05,384 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['task'] instead of .task.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['run'] instead of .run.\n"
b' % (attr, attr))\n'
b"opt/miniconda3/lib/python3.7/site-packages/bids/layout/models.py:147: UserWarning: Accessing entities as attributes is deprecated as of 0.7. Please use the .entities dictionary instead (i.e., .entities['space'] instead of .space.\n"
b' % (attr, attr))\n'
b'200626-21:10:05,620 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/betaseries_wf/404b8b09e303ce148a76cbd3113c48c63e6cbfa2/betaseries_node".\n'
b'200626-21:10:05,622 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/betaseries_wf/534ba0d3cf3b75c435367898cbe23546597ad1dc/betaseries_node".\n'
b'200626-21:10:05,627 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-21:10:05,629 nipype.workflow INFO:\n'
b'\t [Node] Running "betaseries_node" ("nibetaseries.interfaces.nistats.LSSBetaSeries")\n'
b'200626-21:10:07,396 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 112.82/113.22, Free processors: 38/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node\n'
b' * nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 64 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 43 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'opt/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.\n'
b' warnings.warn(msg, category=DeprecationWarning)\n'
b'opt/miniconda3/lib/python3.7/site-packages/nibetaseries/interfaces/nistats.py:82: DeprecationWarning: The parameter "mask" will be removed in next release of Nistats. Please use the parameter "mask_img" instead.\n'
b' minimize_memory=False,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b"opt/miniconda3/lib/python3.7/site-packages/nilearn/image/image.py:214: UserWarning: The parameter 'fwhm' for smoothing is specified as 0.0. Setting it to None (no smoothing will be performed)\n"
b' .format(fwhm))\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b"opt/miniconda3/lib/python3.7/site-packages/nistats/regression.py:339: FutureWarning: 'resid' from RegressionResults has been deprecated and will be removed. Please use 'residuals' instead.\n"
b' FutureWarning,\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 40 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 46 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 42 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 63 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 44 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 65 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 41 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 49 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 47 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 48 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 63 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 66 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 63 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 50 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 51 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 65 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 54 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 55 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 66 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 63 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'\n'
b'Computation of 1 runs done in 45 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 53 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 61 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 56 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 52 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 63 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 59 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 66 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 62 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 58 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 63 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 57 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 60 seconds\n'
b'\n'
b'Computing run 1 out of 1 runs (go take a coffee, a big one)\n'
b'\n'
b'Computation of 1 runs done in 68 seconds\n'
b'\n'
b'sizeof_hdr should be 540; set sizeof_hdr to 540\n'
b'200626-22:34:36,689 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node".\n'
b'200626-22:34:37,728 nipype.workflow INFO:\n'
b'\t [Job 0] Completed (nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node).\n'
b'200626-22:34:37,750 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node\n'
b'200626-22:34:39,738 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 3 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node\n'
b'200626-22:34:39,818 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/404b8b09e303ce148a76cbd3113c48c63e6cbfa2/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-22:34:39,819 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/404b8b09e303ce148a76cbd3113c48c63e6cbfa2/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-22:34:39,820 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/404b8b09e303ce148a76cbd3113c48c63e6cbfa2/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-22:34:39,822 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:34:39,824 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:34:39,825 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:34:39,903 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-22:34:39,903 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-22:34:39,907 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-22:34:41,743 nipype.workflow INFO:\n'
b'\t [Job 4] Completed (_ds_betaseries_file0).\n'
b'200626-22:34:41,744 nipype.workflow INFO:\n'
b'\t [Job 5] Completed (_ds_betaseries_file1).\n'
b'200626-22:34:41,745 nipype.workflow INFO:\n'
b'\t [Job 6] Completed (_ds_betaseries_file2).\n'
b'200626-22:34:41,747 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node\n'
b'200626-22:34:41,868 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject24_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/404b8b09e303ce148a76cbd3113c48c63e6cbfa2/ds_betaseries_file".\n'
b'200626-22:34:41,878 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/404b8b09e303ce148a76cbd3113c48c63e6cbfa2/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-22:34:41,882 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:34:41,962 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-22:34:41,964 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/404b8b09e303ce148a76cbd3113c48c63e6cbfa2/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-22:34:41,967 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:34:42,242 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-22:34:42,244 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/404b8b09e303ce148a76cbd3113c48c63e6cbfa2/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-22:34:42,247 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:34:42,321 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-22:34:42,325 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject24_wf.ds_betaseries_file".\n'
b'200626-22:34:43,751 nipype.workflow INFO:\n'
b'\t [Job 1] Completed (nibetaseries_participant_wf.single_subject24_wf.ds_betaseries_file).\n'
b'200626-22:34:43,758 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 113.02/113.22, Free processors: 39/40.\n'
b' Currently running:\n'
b' * nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node\n'
b'200626-22:35:28,518 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node".\n'
b'200626-22:35:29,931 nipype.workflow INFO:\n'
b'\t [Job 2] Completed (nibetaseries_participant_wf.single_subject24_wf.betaseries_wf.betaseries_node).\n'
b'200626-22:35:29,932 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-22:35:31,939 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-22:35:32,13 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/534ba0d3cf3b75c435367898cbe23546597ad1dc/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-22:35:32,15 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/534ba0d3cf3b75c435367898cbe23546597ad1dc/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-22:35:32,16 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/534ba0d3cf3b75c435367898cbe23546597ad1dc/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-22:35:32,19 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:35:32,19 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:35:32,21 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:35:32,87 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-22:35:32,94 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-22:35:32,94 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-22:35:33,940 nipype.workflow INFO:\n'
b'\t [Job 7] Completed (_ds_betaseries_file0).\n'
b'200626-22:35:33,941 nipype.workflow INFO:\n'
b'\t [Job 8] Completed (_ds_betaseries_file1).\n'
b'200626-22:35:33,942 nipype.workflow INFO:\n'
b'\t [Job 9] Completed (_ds_betaseries_file2).\n'
b'200626-22:35:33,943 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
b'200626-22:35:34,21 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "nibetaseries_participant_wf.single_subject24_wf.ds_betaseries_file" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/534ba0d3cf3b75c435367898cbe23546597ad1dc/ds_betaseries_file".\n'
b'200626-22:35:34,30 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file0" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/534ba0d3cf3b75c435367898cbe23546597ad1dc/ds_betaseries_file/mapflow/_ds_betaseries_file0".\n'
b'200626-22:35:34,34 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file0" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:35:34,111 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file0".\n'
b'200626-22:35:34,113 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file1" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/534ba0d3cf3b75c435367898cbe23546597ad1dc/ds_betaseries_file/mapflow/_ds_betaseries_file1".\n'
b'200626-22:35:34,116 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file1" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:35:34,188 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file1".\n'
b'200626-22:35:34,189 nipype.workflow INFO:\n'
b'\t [Node] Setting-up "_ds_betaseries_file2" in "/data/mvs/derivatives/connectivity/reults/nibetaseries/work/NiBetaSeries_work/nibetaseries_participant_wf/single_subject24_wf/534ba0d3cf3b75c435367898cbe23546597ad1dc/ds_betaseries_file/mapflow/_ds_betaseries_file2".\n'
b'200626-22:35:34,192 nipype.workflow INFO:\n'
b'\t [Node] Running "_ds_betaseries_file2" ("nibetaseries.interfaces.bids.DerivativesDataSink")\n'
b'200626-22:35:34,260 nipype.workflow INFO:\n'
b'\t [Node] Finished "_ds_betaseries_file2".\n'
b'200626-22:35:34,263 nipype.workflow INFO:\n'
b'\t [Node] Finished "nibetaseries_participant_wf.single_subject24_wf.ds_betaseries_file".\n'
b'200626-22:35:35,946 nipype.workflow INFO:\n'
b'\t [Job 3] Completed (nibetaseries_participant_wf.single_subject24_wf.ds_betaseries_file).\n'
b'200626-22:35:35,948 nipype.workflow INFO:\n'
b'\t [MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 113.22/113.22, Free processors: 40/40.\n'
What we have as output are betaseries
files per condition
and run
, each containing n
images where n
reflects the number of presentations of a given condition per run. Thus, we have six betaseries
: for each of two runs, one for music
, singing
and voice
respectively.
listdir(opj(out_dir, 'sub-01/func'))
['sub-01_task-mvs_run-2_space-individual_desc-Singing_betaseries.nii.gz',
'sub-01_task-mvs_run-1_space-individual_desc-Music_betaseries.nii.gz',
'sub-01_task-mvs_run-1_space-individual_desc-Singing_betaseries.nii.gz',
'sub-01_task-mvs_run-2_space-individual_desc-Music_betaseries.nii.gz',
'sub-01_task-mvs_run-1_space-individual_desc-Voice_betaseries.nii.gz',
'sub-01_task-mvs_run-2_space-individual_desc-Voice_betaseries.nii.gz']
And each betaseries
consists of 30
images which matches with how often the conditions were presented:
list_beta = glob(opj(out_dir, 'nibetaseries_transform/*/*.nii.gz'))
list_beta.sort()
for betaseries in list_beta:
print(nb.load(betaseries).shape)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
(97, 115, 97, 30)
We can double check the number of presentations of a given condition
using sub-01
and the music condition
as an example:
pd.read_csv(opj(data_path, 'sub-01/func/sub-01_task-mvs_run-01_events.tsv'), sep='\t').query('trial_type == "Music"').trial_type.count()
30
As done in the MVPA, we will exclude the data of sub-06
as no reliable activation could be detected in the univariate analyses
:
del list_beta[30:36]
Spatial beta series transformation¶
Given that the beta series
are in each participant’s native functional space
, but our auditory cortex atlas
from which we want to extract ROI
values is in MNI152 template space
, we need to apply some sort of spatial transformation
between these spaces
. In general, we have two options: transform
the auditory cortex atlas
into each participant’s functional native space
or transform
each participant’s beta series
into the MNI152 template space
. Following the MVPA, we will go with the second option as it will streamline the planned analyses a bit more. Luckily, we already computed everything we need during the preprocessing. This includes the native functional space to native anatomical space registration
and the native anatomical space to template space registration
. We can even re-use the majority of the code from the corresponding section in preprocessing and individual level analyses.As done there, we will gather the respective transformation matrices
, combine them and then apply them to the beta series
. A first, we are going to import the necessary modules
and functions
:
from os.path import join as opj
from os import mkdir, remove
from nipype.interfaces.ants import ApplyTransforms
from nipype.interfaces.freesurfer import FSCommand, Binarize, Label2Label
from nipype.interfaces.utility import Function, IdentityInterface, Merge
from nipype.interfaces.io import SelectFiles, FreeSurferSource, DataSink
from nipype.pipeline.engine import Workflow, Node, MapNode
from nipype.interfaces.fsl import FLIRT
from glob import glob
from shutil import copy
Next, we will set up the additional paths
we need, including the registration
directory.
input_dir_reg = '/data/mvs/derivatives/registration' # name of registration output folder
input_dir_betaseries = '/data/mvs/derivatives/nibetaseries/' # location of ROI folder
Where should the outputs be stored? How about here:
output_dir = 'derivatives/nibetaseries/nibetaseries_transform' # name of norm output folder
working_dir = 'derivatives/nibetaseries/nibetaseries_transform/working_dir' # name of norm working directory
We also need to define a list of participants
we want to iterate over:
subject_list = [] # create the subject_list variable
#create a study specific subject list
for i in range(1,10):
subject_list.append('sub-0%s' %i)
for i in range(10,25):
subject_list.append('sub-%s' %i)
For the transformation
we only need two processing nodes
: one that merges
the transformation matrices
and one that transforms
the beta series
. For the first, we will use Nipype’s Merge function and for the second ANTs’ ApplyTransforms function. We define and set them up as we did during the preprocessing and individual level analyses section, starting with the merge
node:
merge = Node(Merge(2), iterfield=['in2'], name='mergexfm')
No problem here. The ApplyTransforms
node looked like this:
transform_betaseries = MapNode(ApplyTransforms(args='--float',
input_image_type=3,
interpolation='NearestNeighbor',
invert_transform_flags=[False, False],
num_threads=1,
terminal_output='file'),
name='transform_betaseries', iterfield=['input_image'])
Done. We can already create the workflow
:
transform_betaseries_ANTS_flow = Workflow(name='transform_betaseries_ANTS_flow')
transform_betaseries_ANTS_flow.base_dir = opj(derivatives_path, working_dir)
To get data in our workflow, we also need some input nodes
, starting with infosource
to iterate over participants
:
infosource = Node(IdentityInterface(fields=['subject_id']),
name="infosource")
infosource.iterables = [('subject_id', subject_list)]
We also need the templates
which we will use within the SelectFiles node
:
transform_composite = opj(input_dir_reg, 'antsreg', '{subject_id}', 'transformComposite.h5')
convert2itk = opj(input_dir_reg, 'convert2itk', '{subject_id}', 'affine.txt')
target_func = opj(input_dir_betaseries, '{subject_id}', 'func', '*.nii.gz')
mni_volume_atlas = opj(prereq_path, 'atlas/tpl-MNI152NLin2009cAsym_res-02_desc-ACatlas.nii.gz')
templates = {'transform_composite' : transform_composite,
'convert2itk' : convert2itk,
'target_func': target_func,
'mni_volume_atlas': mni_volume_atlas,
}
And the SelectFiles
node itself:
selectfiles = Node(SelectFiles(templates,
base_directory=derivatives_path),
name="selectfiles")
The input
to our workflow
is set, but we still need the output
. Here our old friend, the datasink node
comes in handy:
datasink = Node(DataSink(base_directory=derivatives_path,
container=output_dir),
name="datasink")
While we are at it, we will also set some substitutions
for our output files
:
substitutions = [('-individual_', '-MNI152NLin2009cAsym_'),
('_trans.nii.gz', '.nii.gz'),
('_subject_id_', '')]
datasink.inputs.substitutions = substitutions
WIth that, we have every information and node we need. Thus, we connect everything within our workflow
:
transform_betaseries_ANTS_flow.connect([(infosource, selectfiles, [('subject_id', 'subject_id')]),
(selectfiles, transform_betaseries,
[('target_func', 'input_image')]),
(selectfiles, transform_betaseries,
[('mni_volume_atlas', 'reference_image')]),
(selectfiles, merge,
[('convert2itk', 'in2')]),
(selectfiles, merge,
[('transform_composite', 'in1')]),
(merge, transform_betaseries, [
('out', 'transforms')]),
(transform_betaseries, datasink,
[('output_image', 'transformed')]),
])
Let’s check if everything fits via plotting the workflow
:
transform_betaseries_ANTS_flow.write_graph(graph2use='colored',format='png', simple_form=True)
transform_betaseries_ANTS_flow.write_graph(graph2use='flat',format='png', simple_form=True)
210212-17:54:02,235 nipype.workflow INFO:
Generated workflow graph: /data/mvs/derivatives/derivatives/nibetaseries/nibetaseries_transform/working_dir/transform_betaseries_ANTS_flow/graph.png (graph2use=colored, simple_form=True).
210212-17:54:03,634 nipype.workflow INFO:
Generated workflow graph: /data/mvs/derivatives/derivatives/nibetaseries/nibetaseries_transform/working_dir/transform_betaseries_ANTS_flow/graph.png (graph2use=flat, simple_form=True).
'/data/mvs/derivatives/derivatives/nibetaseries/nibetaseries_transform/working_dir/transform_betaseries_ANTS_flow/graph.png'
First, the less detailed one:
from IPython.display import Image
Image(filename="/data/mvs/derivatives/derivatives/nibetaseries/nibetaseries_transform/working_dir/transform_betaseries_ANTS_flow/graph.png")
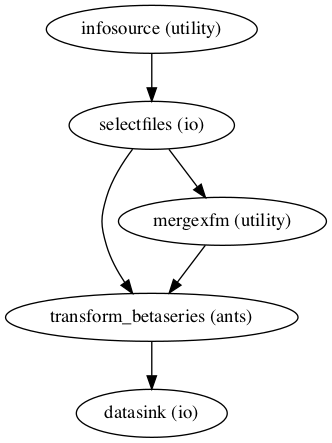
and second, the detailed one:
Image(filename="/data/mvs/derivatives/derivatives/nibetaseries/nibetaseries_transform/working_dir/transform_betaseries_ANTS_flow/graph_detailed.png")
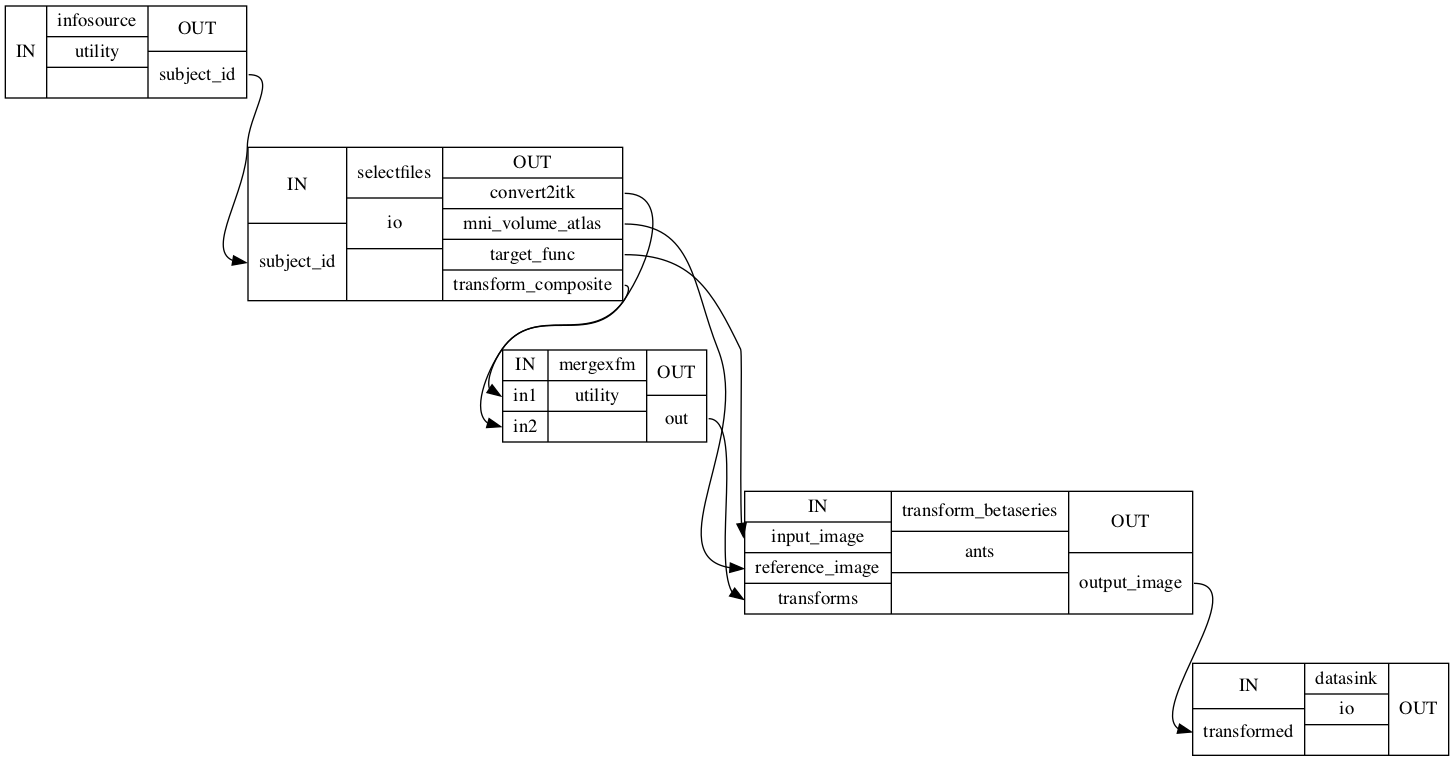
Again, we will not run this part here, but us precomputed outputs as shown in the next step. However, here is how this was done:
transform_betaseries_ANTS_flow.run('MultiProc', plugin_args={'n_procs':4})
Speaking of which, it’s finally time to download the respective files from the project’s OSF page via the osf client. The files we are going to download are the outcomes of the steps above. Please note, that they are rather large in size (~4.5 GB
) and thus things will take a while.
osf -p <projectid> fetch remote/path.txt local/file.txt
The outputs from the this step will now be used to conduct two complementary correlation analyses: ROI to ROI
and voxel to voxel correlations
. Both aim to provide insights into the condition specific information flow
within the auditory cortex
, however at different spatial scales.