Hands-on 1: How to create a fMRI preprocessing workflow¶
The purpose of this section is that you set-up a complete fMRI analysis workflow yourself. So that in the end, you are able to perform the analysis from A-Z, i.e. from preprocessing to group analysis. This section will cover the preprocessing part, and the section Hands-on 2: Analysis will handle the analysis part.
We will use this opportunity to show you some nice additional interfaces/nodes that might not be relevant to your usual analysis. But it’s always nice to know that they exist. And hopefully, this will encourage you to investigate all other interfaces that Nipype can bring to the tip of your finger.
Preparation¶
Before we can start with anything we first need to download the data. For this hands-on, we will only use the right-handed subjects 2-4 and 7-9. This can be done very quickly with the following datalad
command.
Note: This might take a while, as datalad needs to download ~200MB of data
%%bash
datalad get -J 4 -d /home/neuro/workshop/data/ds000114 \
/home/neuro/workshop/data/ds000114/sub-0[234789]/ses-test/anat/sub-0[234789]_ses-test_T1w.nii.gz \
/home/neuro/workshop/data/ds000114/sub-0[234789]/ses-test/func/sub-0[234789]_ses-test_task-fingerfootlips_bold.nii.gz
Preprocessing Workflow Structure¶
So let’s get our hands dirty. First things first, it’s always good to know which interfaces you want to use in your workflow and in which order you want to execute them. For the preprocessing workflow, I recommend that we use the following nodes:
1. Gunzip (Nipype)
2. Drop Dummy Scans (FSL)
3. Slice Time Correction (SPM)
4. Motion Correction (SPM)
5. Artifact Detection
6. Segmentation (SPM)
7. Coregistration (FSL)
8. Smoothing (FSL)
9. Apply Binary Mask (FSL)
10. Remove Linear Trends (Nipype)
Note: This workflow might be overkill concerning data manipulation, but it hopefully serves as a good Nipype exercise.
Imports¶
It’s always best to have all relevant module imports at the beginning of your script. So let’s import what we most certainly need.
from nilearn import plotting
%matplotlib inline
# Get the Node and Workflow object
from nipype import Node, Workflow
# Specify which SPM to use
from nipype.interfaces.matlab import MatlabCommand
MatlabCommand.set_default_paths('/opt/spm12-r7219/spm12_mcr/spm12')
Note: Ideally you would also put the imports of all the interfaces that you use here at the top. But as we will develop the workflow step by step, we can also import the relevant modules as we go.
Create Nodes and Workflow connections¶
Let’s create all the nodes that we need! Make sure to specify all relevant inputs and keep in mind which ones you later on need to connect in your pipeline.
Workflow¶
We recommend to create the workflow and establish all its connections at a later place in your script. This helps to have everything nicely together. But for this hands-on example, it makes sense to establish the connections between the nodes as we go.
And for this, we first need to create a workflow:
# Create the workflow here
# Hint: use 'base_dir' to specify where to store the working directory
preproc = Workflow(name='work_preproc', base_dir='/output/')
Gunzip¶
I’ve already created the Gunzip
node as a template for the other nodes. Also, we’ve specified an in_file
here so that we can directly test the nodes without worrying about the Input/Output data stream to the workflow. This will be taken care of in a later section.
from nipype.algorithms.misc import Gunzip
# Specify example input file
func_file = '/home/neuro/workshop/data/ds000114/sub-07/ses-test/func/sub-07_ses-test_task-fingerfootlips_bold.nii.gz'
# Initiate Gunzip node
gunzip_func = Node(Gunzip(in_file=func_file), name='gunzip_func')
Drop Dummy Scans¶
The functional images of this dataset were recorded with 4 dummy scans at the beginning (see the corresponding publication). But those dummy scans were not yet taken out from the functional images.
To better illustrate this, let’s plot the time course of a random voxel of the just defined func_file
:
import nibabel as nb
%matplotlib inline
import matplotlib.pyplot as plt
plt.plot(nb.load(func_file).get_fdata()[32, 32, 15, :]);
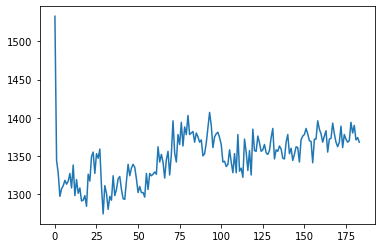
In the figure above, we see that at the very beginning there are extreme values, which hint to the fact that steady state wasn’t reached yet. Therefore, we want to exclude the dummy scans from the original data. This can be achieved with FSL’s ExtractROI
.
from nipype.interfaces.fsl import ExtractROI
extract = Node(ExtractROI(t_min=4, t_size=-1, output_type='NIFTI'),
name="extract")
This ExtractROI
node can now be connected to the gunzip_func
node from above. To do this, we use the following command:
preproc.connect([(gunzip_func, extract, [('out_file', 'in_file')])])
Slice Time Correction¶
Now to the next step. Let’s us SPM’s SliceTiming
to correct for slice wise acquisition of the volumes. As a reminder, the tutorial dataset was recorded…
with a time repetition (TR) of 2.5 seconds
with 30 slices per volume
in an interleaved fashion, i.e. slice order is [1, 3, 5, 7, …, 2, 4, 6, …, 30]
with a time acquisition (TA) of 2.4167 seconds, i.e.
TR-(TR/num_slices)
from nipype.interfaces.spm import SliceTiming
slice_order = list(range(1, 31, 2)) + list(range(2, 31, 2))
print(slice_order)
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24, 26, 28, 30]
# Initiate SliceTiming node here
slicetime = Node(SliceTiming(num_slices=30,
ref_slice=15,
slice_order=slice_order,
time_repetition=2.5,
time_acquisition=2.5-(2.5/30)),
name='slicetime')
Now the next step is to connect the SliceTiming
node to the rest of the workflow, i.e. the ExtractROI
node.
# Connect SliceTiming node to the other nodes here
preproc.connect([(extract, slicetime, [('roi_file', 'in_files')])])
Motion Correction¶
To correct for motion in the scanner, we will be using FSL’s MCFLIRT
.
from nipype.interfaces.fsl import MCFLIRT
# Initate MCFLIRT node here
mcflirt = Node(MCFLIRT(mean_vol=True,
save_plots=True),
name="mcflirt")
Connect the MCFLIRT
node to the rest of the workflow.
# Connect MCFLIRT node to the other nodes here
preproc.connect([(slicetime, mcflirt, [('timecorrected_files', 'in_file')])])
Artifact Detection¶
We will use the really cool and useful ArtifactDetection
tool from Nipype to detect motion and intensity outliers in the functional images. The interface is initiated as follows:
from nipype.algorithms.rapidart import ArtifactDetect
art = Node(ArtifactDetect(norm_threshold=2,
zintensity_threshold=3,
mask_type='spm_global',
parameter_source='FSL',
use_differences=[True, False],
plot_type='svg'),
name="art")
The parameters above mean the following:
norm_threshold
- Threshold to use to detect motion-related outliers when composite motion is being usedzintensity_threshold
- Intensity Z-threshold use to detection images that deviate from the meanmask_type
- Type of mask that should be used to mask the functional data. spm_global uses an spm_global like calculation to determine the brain maskparameter_source
- Source of movement parametersuse_differences
- If you want to use differences between successive motion (first element) and intensity parameter (second element) estimates in order to determine outliers
And this is how you connect this node to the rest of the workflow:
preproc.connect([(mcflirt, art, [('out_file', 'realigned_files'),
('par_file', 'realignment_parameters')])
])
Segmentation of anatomical image¶
Now let’s work on the anatomical image. In particular, let’s use SPM’s NewSegment
to create probability maps for the gray matter, white matter tissue and CSF.
from nipype.interfaces.spm import NewSegment
# Use the following tissue specification to get a GM and WM probability map
tpm_img ='/opt/spm12-r7219/spm12_mcr/spm12/tpm/TPM.nii'
tissue1 = ((tpm_img, 1), 1, (True,False), (False, False))
tissue2 = ((tpm_img, 2), 1, (True,False), (False, False))
tissue3 = ((tpm_img, 3), 2, (True,False), (False, False))
tissue4 = ((tpm_img, 4), 3, (False,False), (False, False))
tissue5 = ((tpm_img, 5), 4, (False,False), (False, False))
tissue6 = ((tpm_img, 6), 2, (False,False), (False, False))
tissues = [tissue1, tissue2, tissue3, tissue4, tissue5, tissue6]
# Initiate NewSegment node here
segment = Node(NewSegment(tissues=tissues), name='segment')
We will again be using a Gunzip
node to unzip the anatomical image that we then want to use as input to the segmentation node. We again also need to specify the anatomical image that we want to use in this case. As before, this will later also be handled directly by the Input/Output stream.
# Specify example input file
anat_file = '/home/neuro/workshop/data/ds000114/sub-07/ses-test/anat/sub-07_ses-test_T1w.nii.gz'
# Initiate Gunzip node
gunzip_anat = Node(Gunzip(in_file=anat_file), name='gunzip_anat')
Now we can connect the NewSegment
node to the rest of the workflow.
# Connect NewSegment node to the other nodes here
preproc.connect([(gunzip_anat, segment, [('out_file', 'channel_files')])])
Compute Coregistration Matrix¶
As a next step, we will make sure that the functional images are coregistered to the anatomical image. For this, we will use FSL’s FLIRT
function. As we just created a white matter probability map, we can use this together with the Boundary-Based Registration (BBR) cost function to optimize the image coregistration. As some helpful notes…
use a degree of freedom of 6
specify the cost function as
bbr
use the
schedule='/usr/share/fsl/5.0/etc/flirtsch/bbr.sch'
from nipype.interfaces.fsl import FLIRT
# Initiate FLIRT node here
coreg = Node(FLIRT(dof=6,
cost='bbr',
schedule='/usr/share/fsl/5.0/etc/flirtsch/bbr.sch',
output_type='NIFTI'),
name="coreg")
# Connect FLIRT node to the other nodes here
preproc.connect([(gunzip_anat, coreg, [('out_file', 'reference')]),
(mcflirt, coreg, [('mean_img', 'in_file')])
])
As mentioned above, the bbr
routine can use the subject-specific white matter probability map to guide the coregistration. But for this, we need to create a binary mask out of the WM probability map. This can easily be done by FSL’s Threshold
interface.
from nipype.interfaces.fsl import Threshold
# Threshold - Threshold WM probability image
threshold_WM = Node(Threshold(thresh=0.5,
args='-bin',
output_type='NIFTI'),
name="threshold_WM")
Now, to select the WM probability map that the NewSegment
node created, we need some helper function. Because the output field partial_volume_files
form the segmentation node, will give us a list of files, i.e. [[GM_prob], [WM_prob], [], [], [], []]
. Therefore, using the following function, we can select only the last element of this list.
# Select WM segmentation file from segmentation output
def get_wm(files):
return files[1][0]
# Connecting the segmentation node with the threshold node
preproc.connect([(segment, threshold_WM, [(('native_class_images', get_wm),
'in_file')])])
Now we can just connect this Threshold
node to the coregistration node from above.
# Connect Threshold node to coregistration node above here
preproc.connect([(threshold_WM, coreg, [('out_file', 'wm_seg')])])
Apply Coregistration Matrix to functional image¶
Now that we know the coregistration matrix to correctly overlay the functional mean image on the subject-specific anatomy, we need to apply to coregistration to the whole time series. This can be achieved with FSL’s FLIRT
as follows:
# Specify the isometric voxel resolution you want after coregistration
desired_voxel_iso = 4
# Apply coregistration warp to functional images
applywarp = Node(FLIRT(interp='spline',
apply_isoxfm=desired_voxel_iso,
output_type='NIFTI'),
name="applywarp")
Important: As you can see above, we also specified a variable desired_voxel_iso
. This is very important at this stage, otherwise FLIRT
will transform your functional images to a resolution of the anatomical image, which will dramatically increase the file size (e.g. to 1-10GB per file). If you don’t want to change the voxel resolution, use the additional parameter no_resample=True
. Important, for this to work, you still need to define apply_isoxfm
.
# Connecting the ApplyWarp node to all the other nodes
preproc.connect([(mcflirt, applywarp, [('out_file', 'in_file')]),
(coreg, applywarp, [('out_matrix_file', 'in_matrix_file')]),
(gunzip_anat, applywarp, [('out_file', 'reference')])
])
Smoothing¶
Next step is image smoothing. The most simple way to do this is to use FSL’s or SPM’s Smooth
function. But for learning purposes, let’s use FSL’s SUSAN
workflow as it is implemented in Nipype. Note that this time, we are importing a workflow instead of an interface.
from niflow.nipype1.workflows.fmri.fsl.preprocess import create_susan_smooth
If you type create_susan_smooth?
you can see how to specify the input variables to the susan workflow. In particular, they are…
fwhm
: set this value to 4 (or whichever value you want)mask_file
: will be created in a later stepin_file
: will be handled while connection to other nodes in the preproc workflow
# Initiate SUSAN workflow here
susan = create_susan_smooth(name='susan')
susan.inputs.inputnode.fwhm = 4
# Connect Threshold node to coregistration node above here
preproc.connect([(applywarp, susan, [('out_file', 'inputnode.in_files')])])
Create Binary Mask¶
There are many possible approaches on how you can mask your functional images. One of them is not at all, one is with a simple brain mask and one that only considers certain kind of brain tissue, e.g. gray matter.
For the current example, we want to create a dilated gray matter mask. For this purpose we need to:
Resample the gray matter probability map to the same resolution as the functional images
Threshold this resampled probability map at a specific value
Dilate this mask by some voxels to make the mask less conservative and more inclusive
The first step can be done in many ways (eg. using freesurfer’s mri_convert
, nibabel
) but in our case, we will use FSL’s FLIRT
. The trick is to use the probability mask, as input file and a reference file.
from nipype.interfaces.fsl import FLIRT
# Initiate resample node
resample = Node(FLIRT(apply_isoxfm=desired_voxel_iso,
output_type='NIFTI'),
name="resample")
The second and third step can luckily be done with just one node. We can take almost the same Threshold
node as above. We just need to add another additional argument: -dilF
- which applies a maximum filtering of all voxels.
from nipype.interfaces.fsl import Threshold
# Threshold - Threshold GM probability image
mask_GM = Node(Threshold(thresh=0.5,
args='-bin -dilF',
output_type='NIFTI'),
name="mask_GM")
# Select GM segmentation file from segmentation output
def get_gm(files):
return files[0][0]
Now we can connect the resample and the gray matter mask node to the segmentation node and each other.
preproc.connect([(segment, resample, [(('native_class_images', get_gm), 'in_file'),
(('native_class_images', get_gm), 'reference')
]),
(resample, mask_GM, [('out_file', 'in_file')])
])
This should do the trick.
Apply the binary mask¶
Now we can connect this dilated gray matter mask to the susan node, as well as actually applying this to the resulting smoothed images.
# Connect gray matter Mask node to the susan workflow here
preproc.connect([(mask_GM, susan, [('out_file', 'inputnode.mask_file')])])
To apply the mask to the smoothed functional images, we will use FSL’s ApplyMask
interface.
from nipype.interfaces.fsl import ApplyMask
Important: The susan workflow gives out a list of files, i.e. [smoothed_func.nii]
instead of just the filename directly. If we would use a normal Node
for ApplyMask
this would lead to the following error:
TraitError: The 'in_file' trait of an ApplyMaskInput instance must be an existing file name, but a value of ['/output/work_preproc/susan/smooth/mapflow/_smooth0/asub-07_ses-test_task-fingerfootlips_bold_mcf_flirt_smooth.nii.gz'] <class 'list'> was specified.
To prevent this we will be using a MapNode
and specify the in_file
as it’s iterfield. Like this, the node is capable to handle a list of inputs as it will know that it has to apply itself iteratively to the list of inputs.
from nipype import MapNode
# Initiate ApplyMask node here
mask_func = MapNode(ApplyMask(output_type='NIFTI'),
name="mask_func",
iterfield=["in_file"])
# Connect smoothed susan output file to ApplyMask node here
preproc.connect([(susan, mask_func, [('outputnode.smoothed_files', 'in_file')]),
(mask_GM, mask_func, [('out_file', 'mask_file')])
])
Remove linear trends in functional images¶
Last but not least. Let’s use Nipype’s TSNR
module to remove linear and quadratic trends in the functionally smoothed images. For this, you only have to specify the regress_poly
parameter in the node initiation.
from nipype.algorithms.confounds import TSNR
# Initiate TSNR node here
detrend = Node(TSNR(regress_poly=2), name="detrend")
# Connect the detrend node to the other nodes here
preproc.connect([(mask_func, detrend, [('out_file', 'in_file')])])
Datainput with SelectFiles
and iterables
¶
This is all nice and well. But so far we still had to specify the input values for gunzip_anat
and gunzip_func
ourselves. How can we scale this up to multiple subjects and/or multiple functional images and make the workflow take the input directly from the BIDS dataset?
For this, we need SelectFiles
and iterables
! It’s rather simple, specify a template and fill-up the placeholder variables.
# Import the SelectFiles
from nipype import SelectFiles
# String template with {}-based strings
templates = {'anat': 'sub-{subject_id}/ses-{ses_id}/anat/'
'sub-{subject_id}_ses-test_T1w.nii.gz',
'func': 'sub-{subject_id}/ses-{ses_id}/func/'
'sub-{subject_id}_ses-{ses_id}_task-{task_id}_bold.nii.gz'}
# Create SelectFiles node
sf = Node(SelectFiles(templates,
base_directory='/home/neuro/workshop/data/ds000114',
sort_filelist=True),
name='selectfiles')
sf.inputs.ses_id='test'
sf.inputs.task_id='fingerfootlips'
Now we can specify over which subjects the workflow should iterate. To test the workflow, let’s still just look at subject 7.
subject_list = ['07']
sf.iterables = [('subject_id', subject_list)]
# Connect SelectFiles node to the other nodes here
preproc.connect([(sf, gunzip_anat, [('anat', 'in_file')]),
(sf, gunzip_func, [('func', 'in_file')])])
Visualize the workflow¶
Now that we’re done. Let’s look at the workflow that we just created.
# Create preproc output graph
preproc.write_graph(graph2use='colored', format='png', simple_form=True)
# Visualize the graph
from IPython.display import Image
Image(filename='/output/work_preproc/graph.png', width=750)
211018-09:51:19,868 nipype.workflow INFO:
Generated workflow graph: /output/work_preproc/graph.png (graph2use=colored, simple_form=True).
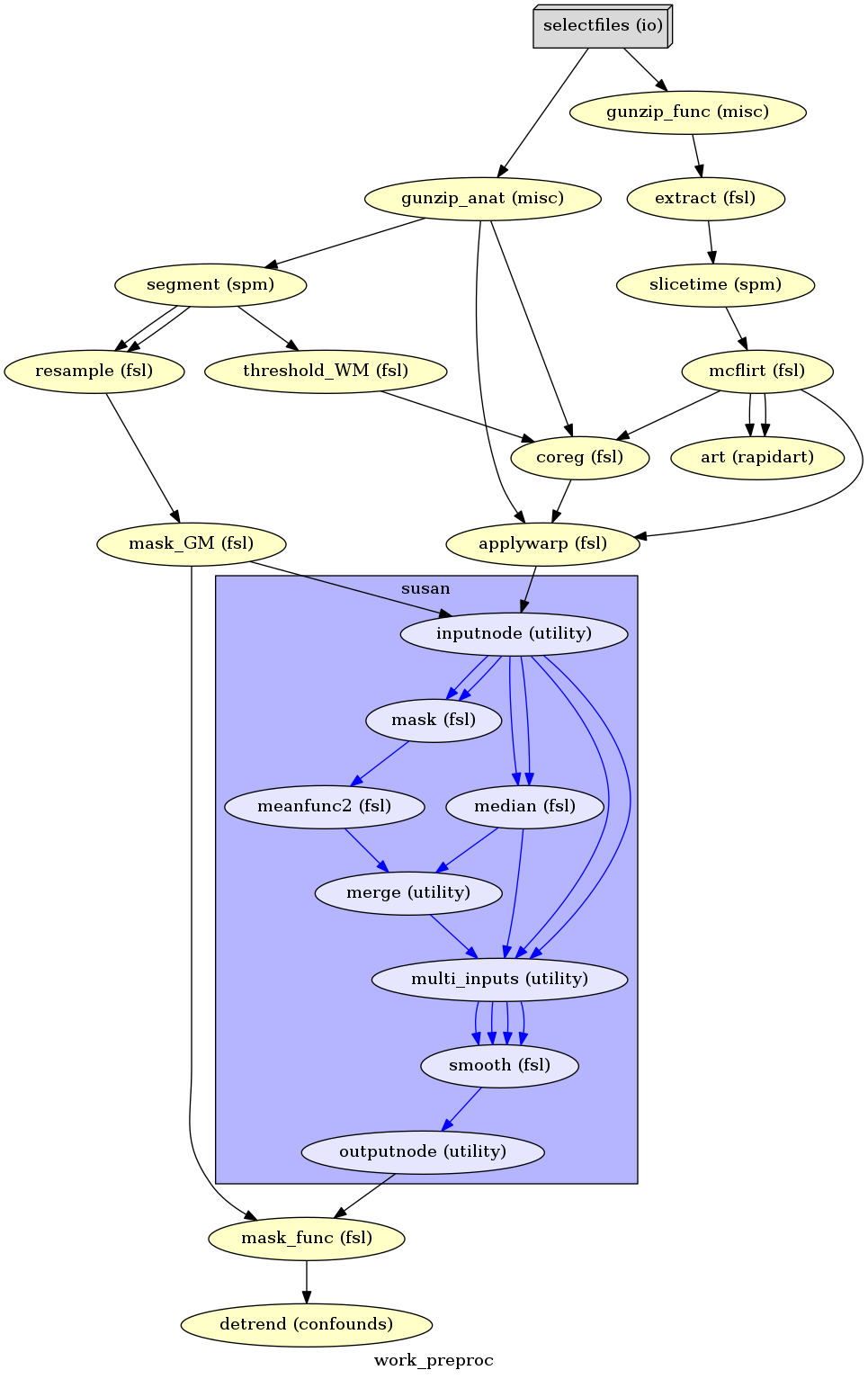
Run the Workflow¶
Now we are ready to run the workflow! Be careful about the n_procs
parameter if you run a workflow in 'MultiProc'
mode. n_procs
specifies the number of jobs/cores your computer will use to run the workflow. If this number is too high your computer will try to execute too many things at once and will most likely crash.
Note: If you’re using a Docker container and FLIRT fails to run without any good reason, you might need to change memory settings in the Docker preferences (6 GB should be enough for this workflow).
preproc.run('MultiProc', plugin_args={'n_procs': 4})
211018-09:51:19,971 nipype.workflow INFO:
Workflow work_preproc settings: ['check', 'execution', 'logging', 'monitoring']
211018-09:51:20,17 nipype.workflow INFO:
Running in parallel.
211018-09:51:20,27 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:51:20,176 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_07/selectfiles".
211018-09:51:20,196 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-09:51:20,209 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-09:51:22,31 nipype.workflow INFO:
[Job 0] Completed (work_preproc.selectfiles).
211018-09:51:22,37 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:51:22,191 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_anat" in "/output/work_preproc/_subject_id_07/gunzip_anat".
211018-09:51:22,191 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_func" in "/output/work_preproc/_subject_id_07/gunzip_func".
211018-09:51:22,207 nipype.workflow INFO:
[Node] Running "gunzip_anat" ("nipype.algorithms.misc.Gunzip")
211018-09:51:22,207 nipype.workflow INFO:
[Node] Running "gunzip_func" ("nipype.algorithms.misc.Gunzip")
211018-09:51:22,491 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_anat".
211018-09:51:22,685 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_func".
211018-09:51:24,30 nipype.workflow INFO:
[Job 1] Completed (work_preproc.gunzip_func).
211018-09:51:24,33 nipype.workflow INFO:
[Job 6] Completed (work_preproc.gunzip_anat).
211018-09:51:24,37 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:51:24,135 nipype.workflow INFO:
[Node] Setting-up "work_preproc.extract" in "/output/work_preproc/_subject_id_07/extract".
211018-09:51:24,137 nipype.workflow INFO:
[Node] Setting-up "work_preproc.segment" in "/output/work_preproc/_subject_id_07/segment".
211018-09:51:24,154 nipype.workflow INFO:
[Node] Running "segment" ("nipype.interfaces.spm.preprocess.NewSegment")
211018-09:51:24,156 nipype.workflow INFO:
[Node] Running "extract" ("nipype.interfaces.fsl.utils.ExtractROI"), a CommandLine Interface with command:
fslroi /output/work_preproc/_subject_id_07/gunzip_func/sub-07_ses-test_task-fingerfootlips_bold.nii /output/work_preproc/_subject_id_07/extract/sub-07_ses-test_task-fingerfootlips_bold_roi.nii 4 -1
211018-09:51:24,805 nipype.workflow INFO:
[Node] Finished "work_preproc.extract".
211018-09:51:26,32 nipype.workflow INFO:
[Job 2] Completed (work_preproc.extract).
211018-09:51:26,35 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.segment
211018-09:51:26,104 nipype.workflow INFO:
[Node] Setting-up "work_preproc.slicetime" in "/output/work_preproc/_subject_id_07/slicetime".
211018-09:51:26,145 nipype.workflow INFO:
[Node] Running "slicetime" ("nipype.interfaces.spm.preprocess.SliceTiming")
211018-09:51:28,35 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.slicetime
* work_preproc.segment
211018-09:51:43,788 nipype.workflow INFO:
[Node] Finished "work_preproc.slicetime".
211018-09:51:44,49 nipype.workflow INFO:
[Job 3] Completed (work_preproc.slicetime).
211018-09:51:44,51 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.segment
211018-09:51:44,128 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mcflirt" in "/output/work_preproc/_subject_id_07/mcflirt".
211018-09:51:44,138 nipype.workflow INFO:
[Node] Running "mcflirt" ("nipype.interfaces.fsl.preprocess.MCFLIRT"), a CommandLine Interface with command:
mcflirt -in /output/work_preproc/_subject_id_07/slicetime/asub-07_ses-test_task-fingerfootlips_bold_roi.nii -meanvol -out /output/work_preproc/_subject_id_07/mcflirt/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -plots
211018-09:51:46,52 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.segment
211018-09:53:00,251 nipype.workflow INFO:
[Node] Finished "work_preproc.mcflirt".
211018-09:53:02,130 nipype.workflow INFO:
[Job 4] Completed (work_preproc.mcflirt).
211018-09:53:02,133 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.segment
211018-09:53:02,216 nipype.workflow INFO:
[Node] Setting-up "work_preproc.art" in "/output/work_preproc/_subject_id_07/art".
211018-09:53:02,230 nipype.workflow INFO:
[Node] Running "art" ("nipype.algorithms.rapidart.ArtifactDetect")
211018-09:53:03,263 nipype.workflow INFO:
[Node] Finished "work_preproc.art".
211018-09:53:04,131 nipype.workflow INFO:
[Job 5] Completed (work_preproc.art).
211018-09:53:04,134 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.segment
211018-09:54:52,35 nipype.workflow INFO:
[Node] Finished "work_preproc.segment".
211018-09:54:52,242 nipype.workflow INFO:
[Job 7] Completed (work_preproc.segment).
211018-09:54:52,247 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:54:52,324 nipype.workflow INFO:
[Node] Setting-up "work_preproc.resample" in "/output/work_preproc/_subject_id_07/resample".
211018-09:54:52,327 nipype.workflow INFO:
[Node] Setting-up "work_preproc.threshold_WM" in "/output/work_preproc/_subject_id_07/threshold_WM".
211018-09:54:52,340 nipype.workflow INFO:
[Node] Running "threshold_WM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_07/segment/c2sub-07_ses-test_T1w.nii -thr 0.5000000000 -bin /output/work_preproc/_subject_id_07/threshold_WM/c2sub-07_ses-test_T1w_thresh.nii
211018-09:54:52,341 nipype.workflow INFO:
[Node] Running "resample" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_07/segment/c1sub-07_ses-test_T1w.nii -ref /output/work_preproc/_subject_id_07/segment/c1sub-07_ses-test_T1w.nii -out c1sub-07_ses-test_T1w_flirt.nii -omat c1sub-07_ses-test_T1w_flirt.mat -applyisoxfm 4.000000
211018-09:54:53,43 nipype.workflow INFO:
[Node] Finished "work_preproc.threshold_WM".
211018-09:54:54,244 nipype.workflow INFO:
[Job 10] Completed (work_preproc.threshold_WM).
211018-09:54:54,247 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.resample
211018-09:54:54,319 nipype.workflow INFO:
[Node] Setting-up "work_preproc.coreg" in "/output/work_preproc/_subject_id_07/coreg".
211018-09:54:54,334 nipype.workflow INFO:
[Node] Running "coreg" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_07/mcflirt/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg.nii.gz -ref /output/work_preproc/_subject_id_07/gunzip_anat/sub-07_ses-test_T1w.nii -out asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.nii -omat asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -cost bbr -dof 6 -schedule /usr/share/fsl/5.0/etc/flirtsch/bbr.sch -wmseg /output/work_preproc/_subject_id_07/threshold_WM/c2sub-07_ses-test_T1w_thresh.nii
211018-09:54:56,123 nipype.workflow INFO:
[Node] Finished "work_preproc.resample".
211018-09:54:56,247 nipype.workflow INFO:
[Job 8] Completed (work_preproc.resample).
211018-09:54:56,250 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-09:54:56,334 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_GM" in "/output/work_preproc/_subject_id_07/mask_GM".
211018-09:54:56,349 nipype.workflow INFO:
[Node] Running "mask_GM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_07/resample/c1sub-07_ses-test_T1w_flirt.nii -thr 0.5000000000 -bin -dilF /output/work_preproc/_subject_id_07/mask_GM/c1sub-07_ses-test_T1w_flirt_thresh.nii
211018-09:54:56,915 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_GM".
211018-09:54:58,249 nipype.workflow INFO:
[Job 9] Completed (work_preproc.mask_GM).
211018-09:54:58,252 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-09:56:44,907 nipype.workflow INFO:
[Node] Finished "work_preproc.coreg".
211018-09:56:46,372 nipype.workflow INFO:
[Job 11] Completed (work_preproc.coreg).
211018-09:56:46,378 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:56:46,495 nipype.workflow INFO:
[Node] Setting-up "work_preproc.applywarp" in "/output/work_preproc/_subject_id_07/applywarp".
211018-09:56:46,560 nipype.workflow INFO:
[Node] Running "applywarp" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_07/mcflirt/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -ref /output/work_preproc/_subject_id_07/gunzip_anat/sub-07_ses-test_T1w.nii -out asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -omat asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.mat -applyisoxfm 4.000000 -init /output/work_preproc/_subject_id_07/coreg/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -interp spline
211018-09:56:48,378 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.applywarp
211018-09:56:58,699 nipype.workflow INFO:
[Node] Finished "work_preproc.applywarp".
211018-09:57:00,389 nipype.workflow INFO:
[Job 12] Completed (work_preproc.applywarp).
211018-09:57:00,393 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:57:00,478 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.mask" in "/output/work_preproc/susan/_subject_id_07/mask".
211018-09:57:00,481 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.median" in "/output/work_preproc/susan/_subject_id_07/median".
211018-09:57:00,493 nipype.workflow INFO:
[Node] Setting-up "_mask0" in "/output/work_preproc/susan/_subject_id_07/mask/mapflow/_mask0".
211018-09:57:00,494 nipype.workflow INFO:
[Node] Setting-up "_median0" in "/output/work_preproc/susan/_subject_id_07/median/mapflow/_median0".
211018-09:57:00,502 nipype.workflow INFO:
[Node] Running "_mask0" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_07/applywarp/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -mas /output/work_preproc/_subject_id_07/mask_GM/c1sub-07_ses-test_T1w_flirt_thresh.nii /output/work_preproc/susan/_subject_id_07/mask/mapflow/_mask0/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz
211018-09:57:00,515 nipype.workflow INFO:
[Node] Running "_median0" ("nipype.interfaces.fsl.utils.ImageStats"), a CommandLine Interface with command:
fslstats /output/work_preproc/_subject_id_07/applywarp/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -k /output/work_preproc/_subject_id_07/mask_GM/c1sub-07_ses-test_T1w_flirt_thresh.nii -p 50
211018-09:57:02,96 nipype.workflow INFO:
[Node] Finished "_median0".
211018-09:57:02,99 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.median".
211018-09:57:02,391 nipype.workflow INFO:
[Job 15] Completed (work_preproc.susan.median).
211018-09:57:02,395 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.susan.mask
211018-09:57:03,799 nipype.workflow INFO:
[Node] Finished "_mask0".
211018-09:57:03,803 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.mask".
211018-09:57:04,398 nipype.workflow INFO:
[Job 13] Completed (work_preproc.susan.mask).
211018-09:57:04,401 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:57:04,493 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.meanfunc2" in "/output/work_preproc/susan/_subject_id_07/meanfunc2".
211018-09:57:04,504 nipype.workflow INFO:
[Node] Setting-up "_meanfunc20" in "/output/work_preproc/susan/_subject_id_07/meanfunc2/mapflow/_meanfunc20".
211018-09:57:04,512 nipype.workflow INFO:
[Node] Running "_meanfunc20" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_07/mask/mapflow/_mask0/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz -Tmean /output/work_preproc/susan/_subject_id_07/meanfunc2/mapflow/_meanfunc20/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz
211018-09:57:05,792 nipype.workflow INFO:
[Node] Finished "_meanfunc20".
211018-09:57:05,797 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.meanfunc2".
211018-09:57:06,401 nipype.workflow INFO:
[Job 14] Completed (work_preproc.susan.meanfunc2).
211018-09:57:06,406 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:57:06,480 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.merge" in "/output/work_preproc/susan/_subject_id_07/merge".
211018-09:57:06,493 nipype.workflow INFO:
[Node] Running "merge" ("nipype.interfaces.utility.base.Merge")
211018-09:57:06,501 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.merge".
211018-09:57:08,410 nipype.workflow INFO:
[Job 16] Completed (work_preproc.susan.merge).
211018-09:57:08,417 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:57:08,488 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.multi_inputs" in "/output/work_preproc/susan/_subject_id_07/multi_inputs".
211018-09:57:08,504 nipype.workflow INFO:
[Node] Running "multi_inputs" ("nipype.interfaces.utility.wrappers.Function")
211018-09:57:08,513 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.multi_inputs".
211018-09:57:10,413 nipype.workflow INFO:
[Job 17] Completed (work_preproc.susan.multi_inputs).
211018-09:57:10,418 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:57:10,495 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.smooth" in "/output/work_preproc/susan/_subject_id_07/smooth".
211018-09:57:10,505 nipype.workflow INFO:
[Node] Setting-up "_smooth0" in "/output/work_preproc/susan/_subject_id_07/smooth/mapflow/_smooth0".
211018-09:57:10,517 nipype.workflow INFO:
[Node] Running "_smooth0" ("nipype.interfaces.fsl.preprocess.SUSAN"), a CommandLine Interface with command:
susan /output/work_preproc/_subject_id_07/applywarp/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii 1046.2500000000 1.6986436006 3 1 1 /output/work_preproc/susan/_subject_id_07/meanfunc2/mapflow/_meanfunc20/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz 1046.2500000000 /output/work_preproc/susan/_subject_id_07/smooth/mapflow/_smooth0/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz
211018-09:57:12,414 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.susan.smooth
211018-09:57:47,781 nipype.workflow INFO:
[Node] Finished "_smooth0".
211018-09:57:47,785 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.smooth".
211018-09:57:48,460 nipype.workflow INFO:
[Job 18] Completed (work_preproc.susan.smooth).
211018-09:57:48,466 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:57:48,551 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_func" in "/output/work_preproc/_subject_id_07/mask_func".
211018-09:57:48,560 nipype.workflow INFO:
[Node] Setting-up "_mask_func0" in "/output/work_preproc/_subject_id_07/mask_func/mapflow/_mask_func0".
211018-09:57:48,581 nipype.workflow INFO:
[Node] Running "_mask_func0" ("nipype.interfaces.fsl.maths.ApplyMask"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_07/smooth/mapflow/_smooth0/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz -mas /output/work_preproc/_subject_id_07/mask_GM/c1sub-07_ses-test_T1w_flirt_thresh.nii /output/work_preproc/_subject_id_07/mask_func/mapflow/_mask_func0/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth_masked.nii
211018-09:57:49,588 nipype.workflow INFO:
[Node] Finished "_mask_func0".
211018-09:57:49,594 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_func".
211018-09:57:50,465 nipype.workflow INFO:
[Job 19] Completed (work_preproc.mask_func).
211018-09:57:50,469 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:57:50,544 nipype.workflow INFO:
[Node] Setting-up "work_preproc.detrend" in "/output/work_preproc/_subject_id_07/detrend".
211018-09:57:50,553 nipype.workflow INFO:
[Node] Running "detrend" ("nipype.algorithms.confounds.TSNR")
211018-09:57:52,466 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.detrend
211018-09:57:55,152 nipype.workflow INFO:
[Node] Finished "work_preproc.detrend".
211018-09:57:56,474 nipype.workflow INFO:
[Job 20] Completed (work_preproc.detrend).
211018-09:57:56,479 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
<networkx.classes.digraph.DiGraph at 0x7f475927a490>
Inspect output¶
What did we actually do? Let’s look at all the data that was created.
!tree /output/work_preproc/ -I '*js|*json|*pklz|_report|*dot|*html|*txt|*.m'
/output/work_preproc/
├── graph.png
├── _subject_id_07
│ ├── applywarp
│ │ ├── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.mat
│ │ └── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii
│ ├── art
│ │ ├── mask.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz
│ │ └── plot.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.svg
│ ├── coreg
│ │ ├── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat
│ │ └── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.nii
│ ├── detrend
│ │ ├── detrend.nii.gz
│ │ ├── mean.nii.gz
│ │ ├── stdev.nii.gz
│ │ └── tsnr.nii.gz
│ ├── extract
│ │ └── sub-07_ses-test_task-fingerfootlips_bold_roi.nii
│ ├── gunzip_anat
│ │ └── sub-07_ses-test_T1w.nii
│ ├── gunzip_func
│ │ └── sub-07_ses-test_task-fingerfootlips_bold.nii
│ ├── mask_func
│ │ └── mapflow
│ │ └── _mask_func0
│ │ └── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth_masked.nii
│ ├── mask_GM
│ │ └── c1sub-07_ses-test_T1w_flirt_thresh.nii
│ ├── mcflirt
│ │ ├── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz
│ │ ├── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg.nii.gz
│ │ └── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par
│ ├── resample
│ │ ├── c1sub-07_ses-test_T1w_flirt.mat
│ │ └── c1sub-07_ses-test_T1w_flirt.nii
│ ├── segment
│ │ ├── c1sub-07_ses-test_T1w.nii
│ │ ├── c2sub-07_ses-test_T1w.nii
│ │ └── c3sub-07_ses-test_T1w.nii
│ ├── selectfiles
│ ├── slicetime
│ │ └── asub-07_ses-test_task-fingerfootlips_bold_roi.nii
│ └── threshold_WM
│ └── c2sub-07_ses-test_T1w_thresh.nii
└── susan
└── _subject_id_07
├── mask
│ └── mapflow
│ └── _mask0
│ └── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz
├── meanfunc2
│ └── mapflow
│ └── _meanfunc20
│ └── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz
├── median
│ └── mapflow
│ └── _median0
├── merge
├── multi_inputs
└── smooth
└── mapflow
└── _smooth0
└── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz
34 directories, 29 files
But what did we do specifically? Well, let’s investigate.
Motion Correction and Artifact Detection¶
How much did the subject move in the scanner and where there any outliers in the functional images?
%matplotlib inline
# Plot the motion paramters
import numpy as np
import matplotlib.pyplot as plt
par = np.loadtxt('/output/work_preproc/_subject_id_07/mcflirt/'
'asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par')
fig, axes = plt.subplots(2, 1, figsize=(15, 5))
axes[0].set_ylabel('rotation (radians)')
axes[0].plot(par[0:, :3])
axes[1].plot(par[0:, 3:])
axes[1].set_xlabel('time (TR)')
axes[1].set_ylabel('translation (mm)');
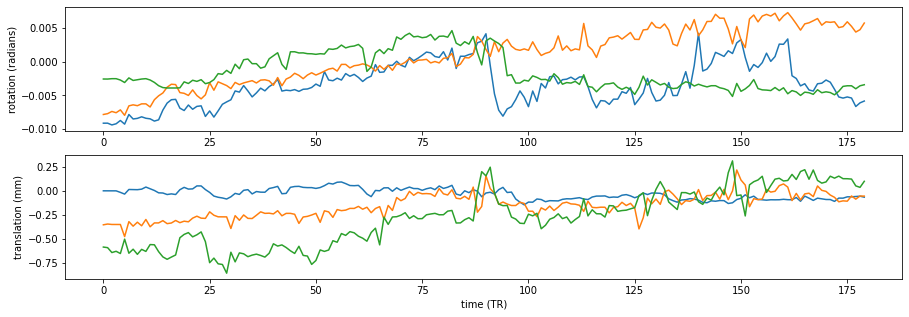
The motion parameters seems to look ok. What about the detection of artifacts?
# Showing the artifact detection output
from IPython.display import SVG
SVG(filename='/output/work_preproc/_subject_id_07/art/'
'plot.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.svg')
Which volumes are problematic?
outliers = np.loadtxt('/output/work_preproc/_subject_id_07/art/'
'art.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt')
list(outliers.astype('int'))
/opt/miniconda-latest/envs/neuro/lib/python3.7/site-packages/ipykernel_launcher.py:1: UserWarning: loadtxt: Empty input file: "/output/work_preproc/_subject_id_07/art/art.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt"
"""Entry point for launching an IPython kernel.
[]
Masks and Probability maps¶
Let’s see what all the masks and probability maps look like. For this, we will use nilearn
’s plot_anat
function.
from nilearn import image as nli
from nilearn.plotting import plot_stat_map
%matplotlib inline
output = '/output/work_preproc/_subject_id_07/'
First, let’s look at the tissue probability maps.
anat = output + 'gunzip_anat/sub-07_ses-test_T1w.nii'
plot_stat_map(
output + 'segment/c1sub-07_ses-test_T1w.nii', title='GM prob. map', cmap=plt.cm.magma,
threshold=0.5, bg_img=anat, display_mode='z', cut_coords=range(-35, 15, 10), dim=-1);
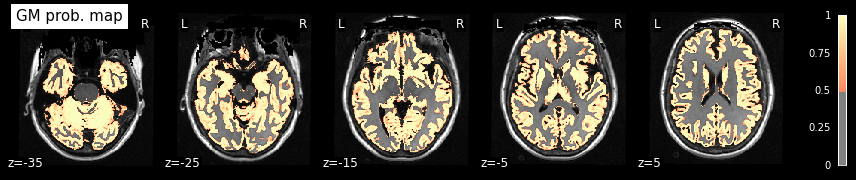
plot_stat_map(
output + 'segment/c2sub-07_ses-test_T1w.nii', title='WM prob. map', cmap=plt.cm.magma,
threshold=0.5, bg_img=anat, display_mode='z', cut_coords=range(-35, 15, 10), dim=-1);
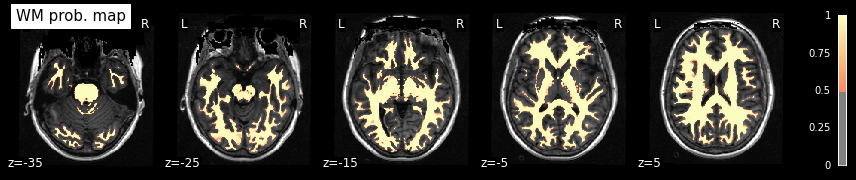
plot_stat_map(
output + 'segment/c3sub-07_ses-test_T1w.nii', title='CSF prob. map', cmap=plt.cm.magma,
threshold=0.5, bg_img=anat, display_mode='z', cut_coords=range(-35, 15, 10), dim=-1);
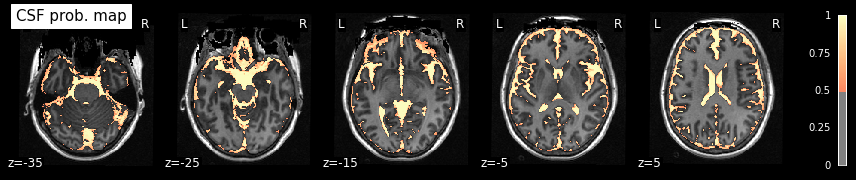
And how does the gray matter mask look like that we used on the functional images?
plot_stat_map(
output + 'mask_GM/c1sub-07_ses-test_T1w_flirt_thresh.nii', title='dilated GM Mask', cmap=plt.cm.magma,
threshold=0.5, bg_img=anat, display_mode='z', cut_coords=range(-35, 15, 10), dim=-1);
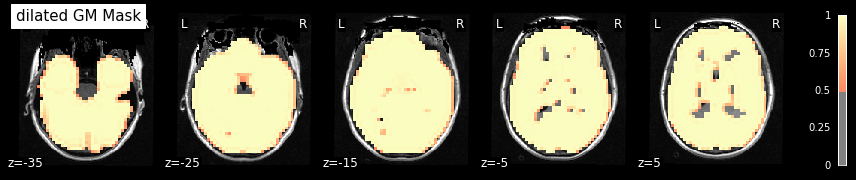
Functional Image transformations¶
Let’s also investigate the transformation that we applied to the functional images.
from nilearn import image as nli
from nilearn.plotting import plot_epi
%matplotlib inline
output = '/output/work_preproc/_subject_id_07/'
plot_epi(output + 'mcflirt/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg.nii.gz',
title='Motion Corrected mean image', display_mode='z', cut_coords=range(-40, 21, 15),
cmap=plt.cm.viridis);
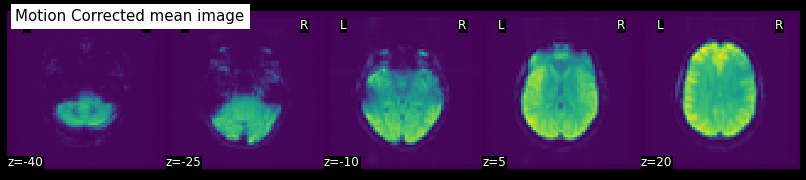
mean = nli.mean_img(output + 'applywarp/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii')
plot_epi(mean, title='Coregistred mean image', display_mode='z', cut_coords=range(-40, 21, 15),
cmap=plt.cm.viridis);
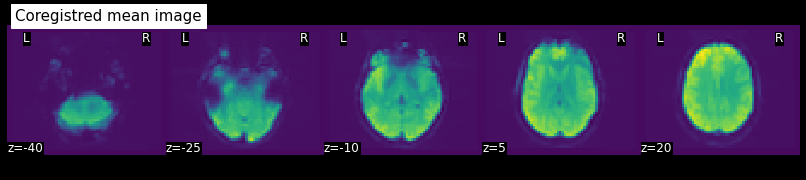
mean = nli.mean_img('/output/work_preproc/susan/_subject_id_07/smooth/mapflow/_smooth0/'
'asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz')
plot_epi(mean, title='Smoothed mean image', display_mode='z', cut_coords=range(-40, 21, 15),
cmap=plt.cm.viridis);
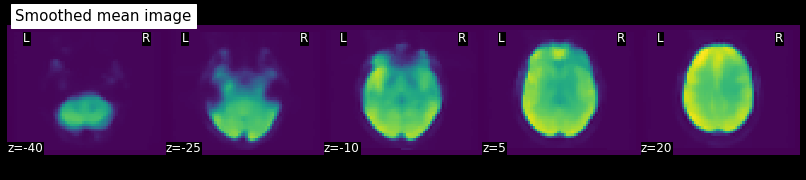
mean = nli.mean_img(output + 'mask_func/mapflow/_mask_func0/'
'asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth_masked.nii')
plot_epi(mean, title='Masked mean image', display_mode='z', cut_coords=range(-40, 21, 15),
cmap=plt.cm.viridis);
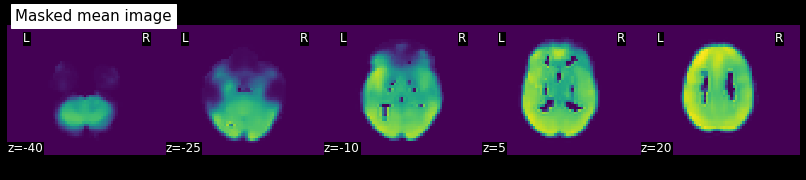
plot_epi(output + 'detrend/mean.nii.gz', title='Detrended mean image', display_mode='z',
cut_coords=range(-40, 21, 15), cmap=plt.cm.viridis);
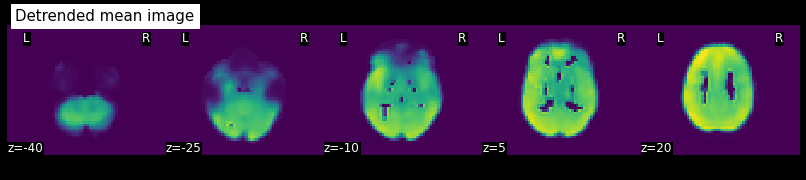
That’s all nice and beautiful, but what did smoothing and detrending actually do to the data?
import nibabel as nb
%matplotlib inline
output = '/output/work_preproc/_subject_id_07/'
# Load the relevant datasets
mc = nb.load(output + 'applywarp/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii')
smooth = nb.load('/output/work_preproc/susan/_subject_id_07/smooth/mapflow/'
'_smooth0/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz')
detrended_data = nb.load(output + 'detrend/detrend.nii.gz')
# Plot a representative voxel
x, y, z = 32, 34, 43
fig = plt.figure(figsize=(12, 4))
plt.plot(mc.get_fdata()[x, y, z, :])
plt.plot(smooth.get_fdata()[x, y, z, :])
plt.plot(detrended_data.get_fdata()[x, y, z, :])
plt.legend(['motion corrected', 'smoothed', 'detrended']);
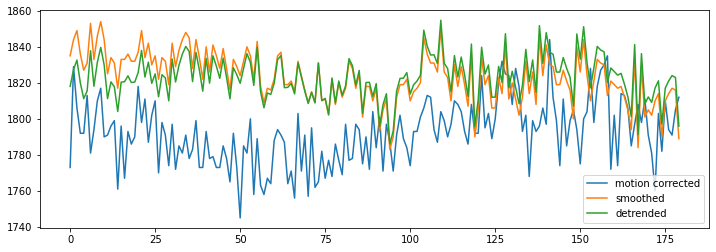
Data output with DataSink
¶
The results look fine, but we don’t need all those temporary files. So let’s use Datasink to keep only those files that we actually need for the 1st and 2nd level analysis.
from nipype.interfaces.io import DataSink
# Initiate the datasink node
output_folder = 'datasink_handson'
datasink = Node(DataSink(base_directory='/output/',
container=output_folder),
name="datasink")
Now the next step is to specify all the output that we want to keep in our output folder output
. Make sure to keep:
from the artifact detection node the outlier file as well as the outlier plot
from the motion correction node the motion parameters
from the last node, the detrended functional image
# Connect nodes to datasink here
preproc.connect([(art, datasink, [('outlier_files', 'preproc.@outlier_files'),
('plot_files', 'preproc.@plot_files')]),
(mcflirt, datasink, [('par_file', 'preproc.@par')]),
(detrend, datasink, [('detrended_file', 'preproc.@func')]),
])
Run the workflow¶
After adding the datasink folder, let’s run the preprocessing workflow again.
preproc.run('MultiProc', plugin_args={'n_procs': 4})
211018-09:58:37,891 nipype.workflow INFO:
Workflow work_preproc settings: ['check', 'execution', 'logging', 'monitoring']
211018-09:58:37,920 nipype.workflow INFO:
Running in parallel.
211018-09:58:37,923 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:58:38,30 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_07/selectfiles".
211018-09:58:38,49 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-09:58:38,62 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-09:58:39,929 nipype.workflow INFO:
[Job 0] Completed (work_preproc.selectfiles).
211018-09:58:39,934 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:58:40,57 nipype.workflow INFO:
[Job 1] Cached (work_preproc.gunzip_func).
211018-09:58:40,62 nipype.workflow INFO:
[Job 6] Cached (work_preproc.gunzip_anat).
211018-09:58:42,9 nipype.workflow INFO:
[Job 2] Cached (work_preproc.extract).
211018-09:58:42,14 nipype.workflow INFO:
[Job 7] Cached (work_preproc.segment).
211018-09:58:43,935 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:58:44,16 nipype.workflow INFO:
[Job 3] Cached (work_preproc.slicetime).
211018-09:58:44,23 nipype.workflow INFO:
[Job 8] Cached (work_preproc.resample).
211018-09:58:44,33 nipype.workflow INFO:
[Job 10] Cached (work_preproc.threshold_WM).
211018-09:58:45,935 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:58:46,24 nipype.workflow INFO:
[Job 4] Cached (work_preproc.mcflirt).
211018-09:58:46,31 nipype.workflow INFO:
[Job 9] Cached (work_preproc.mask_GM).
211018-09:58:48,19 nipype.workflow INFO:
[Node] Outdated cache found for "work_preproc.art".
211018-09:58:48,26 nipype.workflow INFO:
[Node] Setting-up "work_preproc.art" in "/output/work_preproc/_subject_id_07/art".
211018-09:58:48,38 nipype.workflow INFO:
[Node] Outdated cache found for "work_preproc.art".
211018-09:58:48,52 nipype.workflow INFO:
[Job 11] Cached (work_preproc.coreg).
211018-09:58:48,64 nipype.workflow INFO:
[Node] Running "art" ("nipype.algorithms.rapidart.ArtifactDetect")
211018-09:58:49,212 nipype.workflow INFO:
[Node] Finished "work_preproc.art".
211018-09:58:49,946 nipype.workflow INFO:
[Job 5] Completed (work_preproc.art).
211018-09:58:49,948 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:58:50,20 nipype.workflow INFO:
[Job 12] Cached (work_preproc.applywarp).
211018-09:58:51,980 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:58:52,259 nipype.workflow INFO:
[Job 13] Cached (work_preproc.susan.mask).
211018-09:58:52,276 nipype.workflow INFO:
[Job 15] Cached (work_preproc.susan.median).
211018-09:58:53,964 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:58:54,49 nipype.workflow INFO:
[Job 14] Cached (work_preproc.susan.meanfunc2).
211018-09:58:56,41 nipype.workflow INFO:
[Job 16] Cached (work_preproc.susan.merge).
211018-09:58:58,93 nipype.workflow INFO:
[Job 17] Cached (work_preproc.susan.multi_inputs).
211018-09:59:00,227 nipype.workflow INFO:
[Job 18] Cached (work_preproc.susan.smooth).
211018-09:59:02,93 nipype.workflow INFO:
[Job 19] Cached (work_preproc.mask_func).
211018-09:59:04,60 nipype.workflow INFO:
[Node] Outdated cache found for "work_preproc.detrend".
211018-09:59:04,66 nipype.workflow INFO:
[Node] Setting-up "work_preproc.detrend" in "/output/work_preproc/_subject_id_07/detrend".
211018-09:59:04,72 nipype.workflow INFO:
[Node] Outdated cache found for "work_preproc.detrend".
211018-09:59:04,83 nipype.workflow INFO:
[Node] Running "detrend" ("nipype.algorithms.confounds.TSNR")
211018-09:59:05,982 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.detrend
211018-09:59:09,199 nipype.workflow INFO:
[Node] Finished "work_preproc.detrend".
211018-09:59:09,987 nipype.workflow INFO:
[Job 20] Completed (work_preproc.detrend).
211018-09:59:09,991 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:10,80 nipype.workflow INFO:
[Node] Setting-up "work_preproc.datasink" in "/output/work_preproc/_subject_id_07/datasink".
211018-09:59:10,98 nipype.workflow INFO:
[Node] Running "datasink" ("nipype.interfaces.io.DataSink")
211018-09:59:10,111 nipype.workflow INFO:
[Node] Finished "work_preproc.datasink".
211018-09:59:11,993 nipype.workflow INFO:
[Job 21] Completed (work_preproc.datasink).
211018-09:59:11,998 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
<networkx.classes.digraph.DiGraph at 0x7f4758030b50>
Let’s look now at the output of this datasink folder.
!tree /output/datasink_handson -I '*js|*json|*pklz|_report|*dot|*html|*txt|*.m'
/output/datasink_handson
└── preproc
└── _subject_id_07
├── asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par
├── detrend.nii.gz
└── plot.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.svg
2 directories, 3 files
Much better! But we’re still not there yet. There are many unnecessary file specifiers that we can get rid off. To do so, we can use DataSink
’s substitutions
parameter. For this, we create a list of tuples: on the left, we specify the string that we want to replace and on the right, with what we want to replace it with.
## Use the following substitutions for the DataSink output
substitutions = [('asub', 'sub'),
('_ses-test_task-fingerfootlips_bold_roi_mcf', ''),
('.nii.gz.par', '.par'),
]
# To get rid of the folder '_subject_id_07' and renaming detrend
substitutions += [('_subject_id_%s/detrend' % s,
'_subject_id_%s/sub-%s_detrend' % (s, s)) for s in subject_list]
substitutions += [('_subject_id_%s/' % s, '') for s in subject_list]
datasink.inputs.substitutions = substitutions
Before we run the preprocessing workflow again, let’s first delete the current output folder:
# Delets the current output folder
!rm -rf /output/datasink_handson
# Runs the preprocessing workflow again, this time with substitutions
preproc.run('MultiProc', plugin_args={'n_procs': 4})
211018-09:59:15,336 nipype.workflow INFO:
Workflow work_preproc settings: ['check', 'execution', 'logging', 'monitoring']
211018-09:59:15,362 nipype.workflow INFO:
Running in parallel.
211018-09:59:15,365 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:15,497 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_07/selectfiles".
211018-09:59:15,511 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-09:59:15,521 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-09:59:17,370 nipype.workflow INFO:
[Job 0] Completed (work_preproc.selectfiles).
211018-09:59:17,375 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:17,491 nipype.workflow INFO:
[Job 1] Cached (work_preproc.gunzip_func).
211018-09:59:17,496 nipype.workflow INFO:
[Job 6] Cached (work_preproc.gunzip_anat).
211018-09:59:19,452 nipype.workflow INFO:
[Job 2] Cached (work_preproc.extract).
211018-09:59:19,457 nipype.workflow INFO:
[Job 7] Cached (work_preproc.segment).
211018-09:59:21,376 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 3 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:21,466 nipype.workflow INFO:
[Job 3] Cached (work_preproc.slicetime).
211018-09:59:21,471 nipype.workflow INFO:
[Job 8] Cached (work_preproc.resample).
211018-09:59:21,479 nipype.workflow INFO:
[Job 10] Cached (work_preproc.threshold_WM).
211018-09:59:23,382 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:23,534 nipype.workflow INFO:
[Job 4] Cached (work_preproc.mcflirt).
211018-09:59:23,544 nipype.workflow INFO:
[Job 9] Cached (work_preproc.mask_GM).
211018-09:59:25,478 nipype.workflow INFO:
[Job 5] Cached (work_preproc.art).
211018-09:59:25,487 nipype.workflow INFO:
[Job 11] Cached (work_preproc.coreg).
211018-09:59:27,387 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:27,468 nipype.workflow INFO:
[Job 12] Cached (work_preproc.applywarp).
211018-09:59:29,394 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:29,480 nipype.workflow INFO:
[Job 13] Cached (work_preproc.susan.mask).
211018-09:59:29,497 nipype.workflow INFO:
[Job 15] Cached (work_preproc.susan.median).
211018-09:59:31,401 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:31,479 nipype.workflow INFO:
[Job 14] Cached (work_preproc.susan.meanfunc2).
211018-09:59:33,491 nipype.workflow INFO:
[Job 16] Cached (work_preproc.susan.merge).
211018-09:59:35,490 nipype.workflow INFO:
[Job 17] Cached (work_preproc.susan.multi_inputs).
211018-09:59:37,496 nipype.workflow INFO:
[Job 18] Cached (work_preproc.susan.smooth).
211018-09:59:39,503 nipype.workflow INFO:
[Job 19] Cached (work_preproc.mask_func).
211018-09:59:41,498 nipype.workflow INFO:
[Job 20] Cached (work_preproc.detrend).
211018-09:59:43,510 nipype.workflow INFO:
[Node] Outdated cache found for "work_preproc.datasink".
211018-09:59:43,517 nipype.workflow INFO:
[Node] Setting-up "work_preproc.datasink" in "/output/work_preproc/_subject_id_07/datasink".
211018-09:59:43,522 nipype.workflow INFO:
[Node] Outdated cache found for "work_preproc.datasink".
211018-09:59:43,530 nipype.workflow INFO:
[Node] Running "datasink" ("nipype.interfaces.io.DataSink")
211018-09:59:43,534 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_07/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par -> /output/datasink_handson/preproc/sub-07.par
211018-09:59:43,537 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_07/art.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt -> /output/datasink_handson/preproc/art.sub-07_outliers.txt
211018-09:59:43,539 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_07/plot.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.svg -> /output/datasink_handson/preproc/plot.sub-07.svg
211018-09:59:43,542 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_07/detrend.nii.gz -> /output/datasink_handson/preproc/sub-07_detrend.nii.gz
211018-09:59:43,552 nipype.workflow INFO:
[Node] Finished "work_preproc.datasink".
211018-09:59:45,435 nipype.workflow INFO:
[Job 21] Completed (work_preproc.datasink).
211018-09:59:45,440 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
<networkx.classes.digraph.DiGraph at 0x7f47582b0250>
!tree /output/datasink_handson -I '*js|*json|*pklz|_report|*dot|*html|*.m'
/output/datasink_handson
└── preproc
├── art.sub-07_outliers.txt
├── plot.sub-07.svg
├── sub-07_detrend.nii.gz
└── sub-07.par
1 directory, 4 files
Run Preprocessing workflow on 6 right-handed subjects¶
Perfect! Now let’s run the whole workflow for right-handed subjects. For this, you just need to change the subject_list
variable and run again the places where this variable is used (i.e. sf.iterables
and in DataSink
substitutions
.
# Update 'subject_list' and its dependencies here
subject_list = ['02', '03', '04', '07', '08', '09']
sf.iterables = [('subject_id', subject_list)]
# To get rid of the folder '_subject_id_02' and renaming detrend
substitutions += [('_subject_id_%s/detrend' % s,
'_subject_id_%s/sub-%s_detrend' % (s, s)) for s in subject_list]
substitutions += [('_subject_id_%s/' % s, '') for s in subject_list]
datasink.inputs.substitutions = substitutions
Now we can run the workflow again, this time for all right handed subjects in parallel.
# Runs the preprocessing workflow again, this time with substitutions
preproc.run('MultiProc', plugin_args={'n_procs': 4})
211018-09:59:48,139 nipype.workflow INFO:
Workflow work_preproc settings: ['check', 'execution', 'logging', 'monitoring']
211018-09:59:48,249 nipype.workflow INFO:
Running in parallel.
211018-09:59:48,255 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 6 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:48,376 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_08/selectfiles".
211018-09:59:48,378 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_07/selectfiles".
211018-09:59:48,379 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_04/selectfiles".
211018-09:59:48,376 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_09/selectfiles".
211018-09:59:48,388 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-09:59:48,391 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-09:59:48,396 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-09:59:48,396 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-09:59:48,400 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-09:59:48,400 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-09:59:48,405 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-09:59:48,408 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-09:59:50,266 nipype.workflow INFO:
[Job 0] Completed (work_preproc.selectfiles).
211018-09:59:50,270 nipype.workflow INFO:
[Job 22] Completed (work_preproc.selectfiles).
211018-09:59:50,273 nipype.workflow INFO:
[Job 44] Completed (work_preproc.selectfiles).
211018-09:59:50,275 nipype.workflow INFO:
[Job 66] Completed (work_preproc.selectfiles).
211018-09:59:50,279 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 10 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:50,396 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_func" in "/output/work_preproc/_subject_id_09/gunzip_func".
211018-09:59:50,397 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_func" in "/output/work_preproc/_subject_id_08/gunzip_func".
211018-09:59:50,397 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_anat" in "/output/work_preproc/_subject_id_09/gunzip_anat".
211018-09:59:50,400 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_anat" in "/output/work_preproc/_subject_id_08/gunzip_anat".
211018-09:59:50,417 nipype.workflow INFO:
[Node] Running "gunzip_func" ("nipype.algorithms.misc.Gunzip")
211018-09:59:50,417 nipype.workflow INFO:
[Node] Running "gunzip_func" ("nipype.algorithms.misc.Gunzip")
211018-09:59:50,420 nipype.workflow INFO:
[Node] Running "gunzip_anat" ("nipype.algorithms.misc.Gunzip")
211018-09:59:50,420 nipype.workflow INFO:
[Node] Running "gunzip_anat" ("nipype.algorithms.misc.Gunzip")
211018-09:59:51,40 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_anat".
211018-09:59:51,99 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_anat".
211018-09:59:51,524 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_func".
211018-09:59:51,595 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_func".
211018-09:59:52,266 nipype.workflow INFO:
[Job 1] Completed (work_preproc.gunzip_func).
211018-09:59:52,269 nipype.workflow INFO:
[Job 6] Completed (work_preproc.gunzip_anat).
211018-09:59:52,272 nipype.workflow INFO:
[Job 23] Completed (work_preproc.gunzip_func).
211018-09:59:52,274 nipype.workflow INFO:
[Job 28] Completed (work_preproc.gunzip_anat).
211018-09:59:52,278 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 10 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-09:59:52,357 nipype.workflow INFO:
[Node] Setting-up "work_preproc.extract" in "/output/work_preproc/_subject_id_09/extract".
211018-09:59:52,365 nipype.workflow INFO:
[Node] Setting-up "work_preproc.extract" in "/output/work_preproc/_subject_id_08/extract".
211018-09:59:52,367 nipype.workflow INFO:
[Node] Setting-up "work_preproc.segment" in "/output/work_preproc/_subject_id_08/segment".
211018-09:59:52,366 nipype.workflow INFO:
[Node] Setting-up "work_preproc.segment" in "/output/work_preproc/_subject_id_09/segment".
211018-09:59:52,369 nipype.workflow INFO:
[Node] Running "extract" ("nipype.interfaces.fsl.utils.ExtractROI"), a CommandLine Interface with command:
fslroi /output/work_preproc/_subject_id_09/gunzip_func/sub-09_ses-test_task-fingerfootlips_bold.nii /output/work_preproc/_subject_id_09/extract/sub-09_ses-test_task-fingerfootlips_bold_roi.nii 4 -1
211018-09:59:52,379 nipype.workflow INFO:
[Node] Running "extract" ("nipype.interfaces.fsl.utils.ExtractROI"), a CommandLine Interface with command:
fslroi /output/work_preproc/_subject_id_08/gunzip_func/sub-08_ses-test_task-fingerfootlips_bold.nii /output/work_preproc/_subject_id_08/extract/sub-08_ses-test_task-fingerfootlips_bold_roi.nii 4 -1
211018-09:59:52,389 nipype.workflow INFO:
[Node] Running "segment" ("nipype.interfaces.spm.preprocess.NewSegment")
211018-09:59:52,389 nipype.workflow INFO:
[Node] Running "segment" ("nipype.interfaces.spm.preprocess.NewSegment")
211018-09:59:52,916 nipype.workflow INFO:
[Node] Finished "work_preproc.extract".
211018-09:59:52,917 nipype.workflow INFO:
[Node] Finished "work_preproc.extract".
211018-09:59:54,266 nipype.workflow INFO:
[Job 2] Completed (work_preproc.extract).
211018-09:59:54,269 nipype.workflow INFO:
[Job 24] Completed (work_preproc.extract).
211018-09:59:54,273 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 8 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-09:59:54,342 nipype.workflow INFO:
[Node] Setting-up "work_preproc.slicetime" in "/output/work_preproc/_subject_id_08/slicetime".
211018-09:59:54,341 nipype.workflow INFO:
[Node] Setting-up "work_preproc.slicetime" in "/output/work_preproc/_subject_id_09/slicetime".
211018-09:59:54,350 nipype.workflow INFO:
[Node] Running "slicetime" ("nipype.interfaces.spm.preprocess.SliceTiming")
211018-09:59:54,353 nipype.workflow INFO:
[Node] Running "slicetime" ("nipype.interfaces.spm.preprocess.SliceTiming")
211018-09:59:56,269 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 6 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.slicetime
* work_preproc.slicetime
* work_preproc.segment
* work_preproc.segment
211018-10:00:22,441 nipype.workflow INFO:
[Node] Finished "work_preproc.slicetime".
211018-10:00:22,510 nipype.workflow INFO:
[Node] Finished "work_preproc.slicetime".
211018-10:00:24,312 nipype.workflow INFO:
[Job 3] Completed (work_preproc.slicetime).
211018-10:00:24,314 nipype.workflow INFO:
[Job 25] Completed (work_preproc.slicetime).
211018-10:00:24,319 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 8 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:00:24,399 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mcflirt" in "/output/work_preproc/_subject_id_08/mcflirt".
211018-10:00:24,399 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mcflirt" in "/output/work_preproc/_subject_id_09/mcflirt".
211018-10:00:24,411 nipype.workflow INFO:
[Node] Running "mcflirt" ("nipype.interfaces.fsl.preprocess.MCFLIRT"), a CommandLine Interface with command:
mcflirt -in /output/work_preproc/_subject_id_09/slicetime/asub-09_ses-test_task-fingerfootlips_bold_roi.nii -meanvol -out /output/work_preproc/_subject_id_09/mcflirt/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -plots
211018-10:00:24,412 nipype.workflow INFO:
[Node] Running "mcflirt" ("nipype.interfaces.fsl.preprocess.MCFLIRT"), a CommandLine Interface with command:
mcflirt -in /output/work_preproc/_subject_id_08/slicetime/asub-08_ses-test_task-fingerfootlips_bold_roi.nii -meanvol -out /output/work_preproc/_subject_id_08/mcflirt/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -plots
211018-10:00:26,315 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 6 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.mcflirt
* work_preproc.segment
* work_preproc.segment
211018-10:02:00,567 nipype.workflow INFO:
[Node] Finished "work_preproc.mcflirt".
211018-10:02:00,597 nipype.workflow INFO:
[Node] Finished "work_preproc.mcflirt".
211018-10:02:02,404 nipype.workflow INFO:
[Job 4] Completed (work_preproc.mcflirt).
211018-10:02:02,410 nipype.workflow INFO:
[Job 26] Completed (work_preproc.mcflirt).
211018-10:02:02,420 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 8 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:02:02,525 nipype.workflow INFO:
[Node] Setting-up "work_preproc.art" in "/output/work_preproc/_subject_id_08/art".
211018-10:02:02,524 nipype.workflow INFO:
[Node] Setting-up "work_preproc.art" in "/output/work_preproc/_subject_id_09/art".
211018-10:02:02,543 nipype.workflow INFO:
[Node] Running "art" ("nipype.algorithms.rapidart.ArtifactDetect")
211018-10:02:02,544 nipype.workflow INFO:
[Node] Running "art" ("nipype.algorithms.rapidart.ArtifactDetect")
211018-10:02:03,725 nipype.workflow INFO:
[Node] Finished "work_preproc.art".
211018-10:02:03,726 nipype.workflow INFO:
[Node] Finished "work_preproc.art".
211018-10:02:04,406 nipype.workflow INFO:
[Job 5] Completed (work_preproc.art).
211018-10:02:04,408 nipype.workflow INFO:
[Job 27] Completed (work_preproc.art).
211018-10:02:04,411 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 6 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:02:04,480 nipype.workflow INFO:
[Job 45] Cached (work_preproc.gunzip_func).
211018-10:02:04,484 nipype.workflow INFO:
[Job 50] Cached (work_preproc.gunzip_anat).
211018-10:02:06,476 nipype.workflow INFO:
[Job 46] Cached (work_preproc.extract).
211018-10:02:06,480 nipype.workflow INFO:
[Job 51] Cached (work_preproc.segment).
211018-10:02:08,411 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 7 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:02:08,476 nipype.workflow INFO:
[Job 47] Cached (work_preproc.slicetime).
211018-10:02:08,481 nipype.workflow INFO:
[Job 52] Cached (work_preproc.resample).
211018-10:02:10,481 nipype.workflow INFO:
[Job 48] Cached (work_preproc.mcflirt).
211018-10:02:10,486 nipype.workflow INFO:
[Job 53] Cached (work_preproc.mask_GM).
211018-10:02:12,417 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 6 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:02:12,528 nipype.workflow INFO:
[Job 49] Cached (work_preproc.art).
211018-10:02:12,535 nipype.workflow INFO:
[Job 54] Cached (work_preproc.threshold_WM).
211018-10:02:14,419 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 5 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:02:14,506 nipype.workflow INFO:
[Job 55] Cached (work_preproc.coreg).
211018-10:02:14,509 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_func" in "/output/work_preproc/_subject_id_04/gunzip_func".
211018-10:02:14,518 nipype.workflow INFO:
[Node] Running "gunzip_func" ("nipype.algorithms.misc.Gunzip")
211018-10:02:15,610 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_func".
211018-10:02:16,422 nipype.workflow INFO:
[Job 67] Completed (work_preproc.gunzip_func).
211018-10:02:16,430 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 5 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:02:16,631 nipype.workflow INFO:
[Job 56] Cached (work_preproc.applywarp).
211018-10:02:16,641 nipype.workflow INFO:
[Node] Setting-up "work_preproc.extract" in "/output/work_preproc/_subject_id_04/extract".
211018-10:02:16,662 nipype.workflow INFO:
[Node] Running "extract" ("nipype.interfaces.fsl.utils.ExtractROI"), a CommandLine Interface with command:
fslroi /output/work_preproc/_subject_id_04/gunzip_func/sub-04_ses-test_task-fingerfootlips_bold.nii /output/work_preproc/_subject_id_04/extract/sub-04_ses-test_task-fingerfootlips_bold_roi.nii 4 -1
211018-10:02:17,228 nipype.workflow INFO:
[Node] Finished "work_preproc.extract".
211018-10:02:18,422 nipype.workflow INFO:
[Job 68] Completed (work_preproc.extract).
211018-10:02:18,428 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 6 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:02:18,552 nipype.workflow INFO:
[Job 57] Cached (work_preproc.susan.mask).
211018-10:02:18,564 nipype.workflow INFO:
[Job 59] Cached (work_preproc.susan.median).
211018-10:02:20,425 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 5 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:02:20,500 nipype.workflow INFO:
[Job 58] Cached (work_preproc.susan.meanfunc2).
211018-10:02:20,505 nipype.workflow INFO:
[Node] Setting-up "work_preproc.slicetime" in "/output/work_preproc/_subject_id_04/slicetime".
211018-10:02:20,519 nipype.workflow INFO:
[Node] Running "slicetime" ("nipype.interfaces.spm.preprocess.SliceTiming")
211018-10:02:22,427 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 4 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.slicetime
* work_preproc.segment
* work_preproc.segment
211018-10:02:22,521 nipype.workflow INFO:
[Job 60] Cached (work_preproc.susan.merge).
211018-10:02:24,515 nipype.workflow INFO:
[Job 61] Cached (work_preproc.susan.multi_inputs).
211018-10:02:26,502 nipype.workflow INFO:
[Job 62] Cached (work_preproc.susan.smooth).
211018-10:02:28,520 nipype.workflow INFO:
[Job 63] Cached (work_preproc.mask_func).
211018-10:02:30,519 nipype.workflow INFO:
[Job 64] Cached (work_preproc.detrend).
211018-10:02:32,520 nipype.workflow INFO:
[Node] Outdated cache found for "work_preproc.datasink".
211018-10:02:32,526 nipype.workflow INFO:
[Node] Setting-up "work_preproc.datasink" in "/output/work_preproc/_subject_id_07/datasink".
211018-10:02:32,529 nipype.workflow INFO:
[Node] Outdated cache found for "work_preproc.datasink".
211018-10:02:32,536 nipype.workflow INFO:
[Node] Running "datasink" ("nipype.interfaces.io.DataSink")
211018-10:02:32,540 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_07/asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par -> /output/datasink_handson/preproc/sub-07.par
211018-10:02:32,545 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_07/art.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt -> /output/datasink_handson/preproc/art.sub-07_outliers.txt
211018-10:02:32,547 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_07/plot.asub-07_ses-test_task-fingerfootlips_bold_roi_mcf.svg -> /output/datasink_handson/preproc/plot.sub-07.svg
211018-10:02:32,549 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_07/detrend.nii.gz -> /output/datasink_handson/preproc/sub-07_detrend.nii.gz
211018-10:02:32,557 nipype.workflow INFO:
[Node] Finished "work_preproc.datasink".
211018-10:02:34,436 nipype.workflow INFO:
[Job 65] Completed (work_preproc.datasink).
211018-10:02:34,441 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.slicetime
* work_preproc.segment
* work_preproc.segment
211018-10:02:34,531 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_anat" in "/output/work_preproc/_subject_id_04/gunzip_anat".
211018-10:02:34,540 nipype.workflow INFO:
[Node] Running "gunzip_anat" ("nipype.algorithms.misc.Gunzip")
211018-10:02:34,947 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_anat".
211018-10:02:36,438 nipype.workflow INFO:
[Job 72] Completed (work_preproc.gunzip_anat).
211018-10:02:36,442 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.slicetime
* work_preproc.segment
* work_preproc.segment
211018-10:02:36,519 nipype.workflow INFO:
[Node] Setting-up "work_preproc.segment" in "/output/work_preproc/_subject_id_04/segment".
211018-10:02:36,532 nipype.workflow INFO:
[Node] Running "segment" ("nipype.interfaces.spm.preprocess.NewSegment")
211018-10:02:38,442 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.segment
* work_preproc.slicetime
* work_preproc.segment
* work_preproc.segment
211018-10:02:39,502 nipype.workflow INFO:
[Node] Finished "work_preproc.slicetime".
211018-10:02:40,443 nipype.workflow INFO:
[Job 69] Completed (work_preproc.slicetime).
211018-10:02:40,449 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
* work_preproc.segment
211018-10:02:40,545 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mcflirt" in "/output/work_preproc/_subject_id_04/mcflirt".
211018-10:02:40,558 nipype.workflow INFO:
[Node] Running "mcflirt" ("nipype.interfaces.fsl.preprocess.MCFLIRT"), a CommandLine Interface with command:
mcflirt -in /output/work_preproc/_subject_id_04/slicetime/asub-04_ses-test_task-fingerfootlips_bold_roi.nii -meanvol -out /output/work_preproc/_subject_id_04/mcflirt/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -plots
211018-10:02:42,450 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.segment
* work_preproc.segment
* work_preproc.segment
211018-10:04:04,968 nipype.workflow INFO:
[Node] Finished "work_preproc.segment".
211018-10:04:06,551 nipype.workflow INFO:
[Job 7] Completed (work_preproc.segment).
211018-10:04:06,566 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 4 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.segment
* work_preproc.segment
211018-10:04:06,753 nipype.workflow INFO:
[Node] Setting-up "work_preproc.resample" in "/output/work_preproc/_subject_id_09/resample".
211018-10:04:06,770 nipype.workflow INFO:
[Node] Running "resample" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_09/segment/c1sub-09_ses-test_T1w.nii -ref /output/work_preproc/_subject_id_09/segment/c1sub-09_ses-test_T1w.nii -out c1sub-09_ses-test_T1w_flirt.nii -omat c1sub-09_ses-test_T1w_flirt.mat -applyisoxfm 4.000000
211018-10:04:08,554 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 3 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.resample
* work_preproc.mcflirt
* work_preproc.segment
* work_preproc.segment
211018-10:04:12,62 nipype.workflow INFO:
[Node] Finished "work_preproc.resample".
211018-10:04:12,557 nipype.workflow INFO:
[Job 8] Completed (work_preproc.resample).
211018-10:04:12,564 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 4 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.segment
* work_preproc.segment
211018-10:04:12,673 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_GM" in "/output/work_preproc/_subject_id_09/mask_GM".
211018-10:04:12,692 nipype.workflow INFO:
[Node] Running "mask_GM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_09/resample/c1sub-09_ses-test_T1w_flirt.nii -thr 0.5000000000 -bin -dilF /output/work_preproc/_subject_id_09/mask_GM/c1sub-09_ses-test_T1w_flirt_thresh.nii
211018-10:04:13,298 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_GM".
211018-10:04:13,512 nipype.workflow INFO:
[Node] Finished "work_preproc.mcflirt".
211018-10:04:14,558 nipype.workflow INFO:
[Job 70] Completed (work_preproc.mcflirt).
211018-10:04:14,560 nipype.workflow INFO:
[Job 9] Completed (work_preproc.mask_GM).
211018-10:04:14,565 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 4 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:04:14,645 nipype.workflow INFO:
[Node] Setting-up "work_preproc.art" in "/output/work_preproc/_subject_id_04/art".
211018-10:04:14,645 nipype.workflow INFO:
[Node] Setting-up "work_preproc.threshold_WM" in "/output/work_preproc/_subject_id_09/threshold_WM".
211018-10:04:14,659 nipype.workflow INFO:
[Node] Running "art" ("nipype.algorithms.rapidart.ArtifactDetect")
211018-10:04:14,660 nipype.workflow INFO:
[Node] Running "threshold_WM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_09/segment/c2sub-09_ses-test_T1w.nii -thr 0.5000000000 -bin /output/work_preproc/_subject_id_09/threshold_WM/c2sub-09_ses-test_T1w_thresh.nii
211018-10:04:15,353 nipype.workflow INFO:
[Node] Finished "work_preproc.threshold_WM".
211018-10:04:15,685 nipype.workflow INFO:
[Node] Finished "work_preproc.art".
211018-10:04:16,16 nipype.workflow INFO:
[Node] Finished "work_preproc.segment".
211018-10:04:16,560 nipype.workflow INFO:
[Job 29] Completed (work_preproc.segment).
211018-10:04:16,562 nipype.workflow INFO:
[Job 10] Completed (work_preproc.threshold_WM).
211018-10:04:16,564 nipype.workflow INFO:
[Job 71] Completed (work_preproc.art).
211018-10:04:16,569 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 5 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.segment
211018-10:04:16,640 nipype.workflow INFO:
[Node] Setting-up "work_preproc.coreg" in "/output/work_preproc/_subject_id_09/coreg".
211018-10:04:16,641 nipype.workflow INFO:
[Node] Setting-up "work_preproc.resample" in "/output/work_preproc/_subject_id_08/resample".
211018-10:04:16,642 nipype.workflow INFO:
[Node] Setting-up "work_preproc.threshold_WM" in "/output/work_preproc/_subject_id_08/threshold_WM".
211018-10:04:16,656 nipype.workflow INFO:
[Node] Running "resample" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_08/segment/c1sub-08_ses-test_T1w.nii -ref /output/work_preproc/_subject_id_08/segment/c1sub-08_ses-test_T1w.nii -out c1sub-08_ses-test_T1w_flirt.nii -omat c1sub-08_ses-test_T1w_flirt.mat -applyisoxfm 4.000000
211018-10:04:16,655 nipype.workflow INFO:
[Node] Running "threshold_WM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_08/segment/c2sub-08_ses-test_T1w.nii -thr 0.5000000000 -bin /output/work_preproc/_subject_id_08/threshold_WM/c2sub-08_ses-test_T1w_thresh.nii
211018-10:04:16,659 nipype.workflow INFO:
[Node] Running "coreg" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_09/mcflirt/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg.nii.gz -ref /output/work_preproc/_subject_id_09/gunzip_anat/sub-09_ses-test_T1w.nii -out asub-09_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.nii -omat asub-09_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -cost bbr -dof 6 -schedule /usr/share/fsl/5.0/etc/flirtsch/bbr.sch -wmseg /output/work_preproc/_subject_id_09/threshold_WM/c2sub-09_ses-test_T1w_thresh.nii
211018-10:04:17,310 nipype.workflow INFO:
[Node] Finished "work_preproc.threshold_WM".
211018-10:04:18,564 nipype.workflow INFO:
[Job 32] Completed (work_preproc.threshold_WM).
211018-10:04:18,570 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.resample
* work_preproc.coreg
* work_preproc.segment
211018-10:04:18,703 nipype.workflow INFO:
[Node] Setting-up "work_preproc.coreg" in "/output/work_preproc/_subject_id_08/coreg".
211018-10:04:18,739 nipype.workflow INFO:
[Node] Running "coreg" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_08/mcflirt/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg.nii.gz -ref /output/work_preproc/_subject_id_08/gunzip_anat/sub-08_ses-test_T1w.nii -out asub-08_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.nii -omat asub-08_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -cost bbr -dof 6 -schedule /usr/share/fsl/5.0/etc/flirtsch/bbr.sch -wmseg /output/work_preproc/_subject_id_08/threshold_WM/c2sub-08_ses-test_T1w_thresh.nii
211018-10:04:20,566 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.coreg
* work_preproc.resample
* work_preproc.coreg
* work_preproc.segment
211018-10:04:21,1 nipype.workflow INFO:
[Node] Finished "work_preproc.resample".
211018-10:04:22,567 nipype.workflow INFO:
[Job 30] Completed (work_preproc.resample).
211018-10:04:22,572 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.coreg
* work_preproc.segment
211018-10:04:22,643 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_GM" in "/output/work_preproc/_subject_id_08/mask_GM".
211018-10:04:22,654 nipype.workflow INFO:
[Node] Running "mask_GM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_08/resample/c1sub-08_ses-test_T1w_flirt.nii -thr 0.5000000000 -bin -dilF /output/work_preproc/_subject_id_08/mask_GM/c1sub-08_ses-test_T1w_flirt_thresh.nii
211018-10:04:23,212 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_GM".
211018-10:04:24,569 nipype.workflow INFO:
[Job 31] Completed (work_preproc.mask_GM).
211018-10:04:24,573 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.coreg
* work_preproc.segment
211018-10:04:24,642 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_03/selectfiles".
211018-10:04:24,650 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-10:04:24,659 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-10:04:26,571 nipype.workflow INFO:
[Job 88] Completed (work_preproc.selectfiles).
211018-10:04:26,575 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.coreg
* work_preproc.segment
211018-10:04:26,645 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_func" in "/output/work_preproc/_subject_id_03/gunzip_func".
211018-10:04:26,656 nipype.workflow INFO:
[Node] Running "gunzip_func" ("nipype.algorithms.misc.Gunzip")
211018-10:04:27,187 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_func".
211018-10:04:28,573 nipype.workflow INFO:
[Job 89] Completed (work_preproc.gunzip_func).
211018-10:04:28,577 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.coreg
* work_preproc.segment
211018-10:04:28,664 nipype.workflow INFO:
[Node] Setting-up "work_preproc.extract" in "/output/work_preproc/_subject_id_03/extract".
211018-10:04:28,674 nipype.workflow INFO:
[Node] Running "extract" ("nipype.interfaces.fsl.utils.ExtractROI"), a CommandLine Interface with command:
fslroi /output/work_preproc/_subject_id_03/gunzip_func/sub-03_ses-test_task-fingerfootlips_bold.nii /output/work_preproc/_subject_id_03/extract/sub-03_ses-test_task-fingerfootlips_bold_roi.nii 4 -1
211018-10:04:29,118 nipype.workflow INFO:
[Node] Finished "work_preproc.extract".
211018-10:04:30,576 nipype.workflow INFO:
[Job 90] Completed (work_preproc.extract).
211018-10:04:30,581 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.coreg
* work_preproc.segment
211018-10:04:30,669 nipype.workflow INFO:
[Node] Setting-up "work_preproc.slicetime" in "/output/work_preproc/_subject_id_03/slicetime".
211018-10:04:30,686 nipype.workflow INFO:
[Node] Running "slicetime" ("nipype.interfaces.spm.preprocess.SliceTiming")
211018-10:04:32,579 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.slicetime
* work_preproc.coreg
* work_preproc.coreg
* work_preproc.segment
211018-10:04:50,299 nipype.workflow INFO:
[Node] Finished "work_preproc.slicetime".
211018-10:04:50,598 nipype.workflow INFO:
[Job 91] Completed (work_preproc.slicetime).
211018-10:04:50,603 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.coreg
* work_preproc.segment
211018-10:04:50,685 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mcflirt" in "/output/work_preproc/_subject_id_03/mcflirt".
211018-10:04:50,696 nipype.workflow INFO:
[Node] Running "mcflirt" ("nipype.interfaces.fsl.preprocess.MCFLIRT"), a CommandLine Interface with command:
mcflirt -in /output/work_preproc/_subject_id_03/slicetime/asub-03_ses-test_task-fingerfootlips_bold_roi.nii -meanvol -out /output/work_preproc/_subject_id_03/mcflirt/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -plots
211018-10:04:52,601 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.coreg
* work_preproc.coreg
* work_preproc.segment
211018-10:06:23,896 nipype.workflow INFO:
[Node] Finished "work_preproc.coreg".
211018-10:06:24,718 nipype.workflow INFO:
[Job 11] Completed (work_preproc.coreg).
211018-10:06:24,723 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.coreg
* work_preproc.segment
211018-10:06:24,835 nipype.workflow INFO:
[Node] Setting-up "work_preproc.applywarp" in "/output/work_preproc/_subject_id_09/applywarp".
211018-10:06:24,858 nipype.workflow INFO:
[Node] Running "applywarp" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_09/mcflirt/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -ref /output/work_preproc/_subject_id_09/gunzip_anat/sub-09_ses-test_T1w.nii -out asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -omat asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.mat -applyisoxfm 4.000000 -init /output/work_preproc/_subject_id_09/coreg/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -interp spline
211018-10:06:26,721 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.applywarp
* work_preproc.mcflirt
* work_preproc.coreg
* work_preproc.segment
211018-10:06:27,279 nipype.workflow INFO:
[Node] Finished "work_preproc.coreg".
211018-10:06:27,965 nipype.workflow INFO:
[Node] Finished "work_preproc.mcflirt".
211018-10:06:28,722 nipype.workflow INFO:
[Job 33] Completed (work_preproc.coreg).
211018-10:06:28,725 nipype.workflow INFO:
[Job 92] Completed (work_preproc.mcflirt).
211018-10:06:28,732 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 4 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.applywarp
* work_preproc.segment
211018-10:06:28,832 nipype.workflow INFO:
[Node] Setting-up "work_preproc.applywarp" in "/output/work_preproc/_subject_id_08/applywarp".
211018-10:06:28,836 nipype.workflow INFO:
[Node] Setting-up "work_preproc.art" in "/output/work_preproc/_subject_id_03/art".
211018-10:06:28,854 nipype.workflow INFO:
[Node] Running "art" ("nipype.algorithms.rapidart.ArtifactDetect")
211018-10:06:28,859 nipype.workflow INFO:
[Node] Running "applywarp" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_08/mcflirt/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -ref /output/work_preproc/_subject_id_08/gunzip_anat/sub-08_ses-test_T1w.nii -out asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -omat asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.mat -applyisoxfm 4.000000 -init /output/work_preproc/_subject_id_08/coreg/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -interp spline
211018-10:06:29,864 nipype.workflow INFO:
[Node] Finished "work_preproc.art".
211018-10:06:30,724 nipype.workflow INFO:
[Job 93] Completed (work_preproc.art).
211018-10:06:30,729 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.applywarp
* work_preproc.applywarp
* work_preproc.segment
211018-10:06:30,809 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_anat" in "/output/work_preproc/_subject_id_03/gunzip_anat".
211018-10:06:30,822 nipype.workflow INFO:
[Node] Running "gunzip_anat" ("nipype.algorithms.misc.Gunzip")
211018-10:06:31,302 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_anat".
211018-10:06:32,724 nipype.workflow INFO:
[Job 94] Completed (work_preproc.gunzip_anat).
211018-10:06:32,729 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.applywarp
* work_preproc.applywarp
* work_preproc.segment
211018-10:06:32,812 nipype.workflow INFO:
[Node] Setting-up "work_preproc.segment" in "/output/work_preproc/_subject_id_03/segment".
211018-10:06:32,838 nipype.workflow INFO:
[Node] Running "segment" ("nipype.interfaces.spm.preprocess.NewSegment")
211018-10:06:34,727 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 1 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.segment
* work_preproc.applywarp
* work_preproc.applywarp
* work_preproc.segment
211018-10:06:37,993 nipype.workflow INFO:
[Node] Finished "work_preproc.applywarp".
211018-10:06:38,731 nipype.workflow INFO:
[Job 12] Completed (work_preproc.applywarp).
211018-10:06:38,735 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.segment
* work_preproc.applywarp
* work_preproc.segment
211018-10:06:38,810 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.mask" in "/output/work_preproc/susan/_subject_id_09/mask".
211018-10:06:38,821 nipype.workflow INFO:
[Node] Setting-up "_mask0" in "/output/work_preproc/susan/_subject_id_09/mask/mapflow/_mask0".
211018-10:06:38,832 nipype.workflow INFO:
[Node] Running "_mask0" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_09/applywarp/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -mas /output/work_preproc/_subject_id_09/mask_GM/c1sub-09_ses-test_T1w_flirt_thresh.nii /output/work_preproc/susan/_subject_id_09/mask/mapflow/_mask0/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz
211018-10:06:40,737 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.susan.mask
* work_preproc.segment
* work_preproc.applywarp
* work_preproc.segment
211018-10:06:41,684 nipype.workflow INFO:
[Node] Finished "work_preproc.applywarp".
211018-10:06:42,736 nipype.workflow INFO:
[Job 34] Completed (work_preproc.applywarp).
211018-10:06:42,741 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 4 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.mask
* work_preproc.segment
* work_preproc.segment
211018-10:06:42,833 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.median" in "/output/work_preproc/susan/_subject_id_09/median".
211018-10:06:42,842 nipype.workflow INFO:
[Node] Setting-up "_median0" in "/output/work_preproc/susan/_subject_id_09/median/mapflow/_median0".
211018-10:06:42,849 nipype.workflow INFO:
[Node] Running "_median0" ("nipype.interfaces.fsl.utils.ImageStats"), a CommandLine Interface with command:
fslstats /output/work_preproc/_subject_id_09/applywarp/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -k /output/work_preproc/_subject_id_09/mask_GM/c1sub-09_ses-test_T1w_flirt_thresh.nii -p 50
211018-10:06:43,66 nipype.workflow INFO:
[Node] Finished "_mask0".
211018-10:06:43,71 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.mask".
211018-10:06:44,739 nipype.workflow INFO:
[Job 13] Completed (work_preproc.susan.mask).
211018-10:06:44,747 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 4 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.median
* work_preproc.segment
* work_preproc.segment
211018-10:06:44,859 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.meanfunc2" in "/output/work_preproc/susan/_subject_id_09/meanfunc2".
211018-10:06:44,887 nipype.workflow INFO:
[Node] Setting-up "_meanfunc20" in "/output/work_preproc/susan/_subject_id_09/meanfunc2/mapflow/_meanfunc20".
211018-10:06:44,912 nipype.workflow INFO:
[Node] Running "_meanfunc20" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_09/mask/mapflow/_mask0/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz -Tmean /output/work_preproc/susan/_subject_id_09/meanfunc2/mapflow/_meanfunc20/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz
211018-10:06:45,504 nipype.workflow INFO:
[Node] Finished "_median0".
211018-10:06:45,514 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.median".
211018-10:06:46,728 nipype.workflow INFO:
[Node] Finished "_meanfunc20".
211018-10:06:46,732 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.meanfunc2".
211018-10:06:46,741 nipype.workflow INFO:
[Job 15] Completed (work_preproc.susan.median).
211018-10:06:46,744 nipype.workflow INFO:
[Job 14] Completed (work_preproc.susan.meanfunc2).
211018-10:06:46,748 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 4 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:06:46,838 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.mask" in "/output/work_preproc/susan/_subject_id_08/mask".
211018-10:06:46,837 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.merge" in "/output/work_preproc/susan/_subject_id_09/merge".
211018-10:06:46,848 nipype.workflow INFO:
[Node] Setting-up "_mask0" in "/output/work_preproc/susan/_subject_id_08/mask/mapflow/_mask0".
211018-10:06:46,851 nipype.workflow INFO:
[Node] Running "merge" ("nipype.interfaces.utility.base.Merge")
211018-10:06:46,863 nipype.workflow INFO:
[Node] Running "_mask0" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_08/applywarp/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -mas /output/work_preproc/_subject_id_08/mask_GM/c1sub-08_ses-test_T1w_flirt_thresh.nii /output/work_preproc/susan/_subject_id_08/mask/mapflow/_mask0/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz
211018-10:06:46,863 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.merge".
211018-10:06:48,743 nipype.workflow INFO:
[Job 16] Completed (work_preproc.susan.merge).
211018-10:06:48,746 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.mask
* work_preproc.segment
* work_preproc.segment
211018-10:06:48,818 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.multi_inputs" in "/output/work_preproc/susan/_subject_id_09/multi_inputs".
211018-10:06:48,833 nipype.workflow INFO:
[Node] Running "multi_inputs" ("nipype.interfaces.utility.wrappers.Function")
211018-10:06:48,846 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.multi_inputs".
211018-10:06:50,541 nipype.workflow INFO:
[Node] Finished "_mask0".
211018-10:06:50,546 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.mask".
211018-10:06:50,745 nipype.workflow INFO:
[Job 35] Completed (work_preproc.susan.mask).
211018-10:06:50,748 nipype.workflow INFO:
[Job 17] Completed (work_preproc.susan.multi_inputs).
211018-10:06:50,754 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 4 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:06:50,846 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.smooth" in "/output/work_preproc/susan/_subject_id_09/smooth".
211018-10:06:50,849 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.meanfunc2" in "/output/work_preproc/susan/_subject_id_08/meanfunc2".
211018-10:06:50,861 nipype.workflow INFO:
[Node] Setting-up "_meanfunc20" in "/output/work_preproc/susan/_subject_id_08/meanfunc2/mapflow/_meanfunc20".
211018-10:06:50,859 nipype.workflow INFO:
[Node] Setting-up "_smooth0" in "/output/work_preproc/susan/_subject_id_09/smooth/mapflow/_smooth0".
211018-10:06:50,868 nipype.workflow INFO:
[Node] Running "_meanfunc20" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_08/mask/mapflow/_mask0/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz -Tmean /output/work_preproc/susan/_subject_id_08/meanfunc2/mapflow/_meanfunc20/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz
211018-10:06:50,878 nipype.workflow INFO:
[Node] Running "_smooth0" ("nipype.interfaces.fsl.preprocess.SUSAN"), a CommandLine Interface with command:
susan /output/work_preproc/_subject_id_09/applywarp/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii 1116.0000000000 1.6986436006 3 1 1 /output/work_preproc/susan/_subject_id_09/meanfunc2/mapflow/_meanfunc20/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz 1116.0000000000 /output/work_preproc/susan/_subject_id_09/smooth/mapflow/_smooth0/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz
211018-10:06:52,406 nipype.workflow INFO:
[Node] Finished "_meanfunc20".
211018-10:06:52,412 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.meanfunc2".
211018-10:06:52,747 nipype.workflow INFO:
[Job 36] Completed (work_preproc.susan.meanfunc2).
211018-10:06:52,751 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.segment
* work_preproc.segment
211018-10:06:52,820 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.median" in "/output/work_preproc/susan/_subject_id_08/median".
211018-10:06:52,829 nipype.workflow INFO:
[Node] Setting-up "_median0" in "/output/work_preproc/susan/_subject_id_08/median/mapflow/_median0".
211018-10:06:52,837 nipype.workflow INFO:
[Node] Running "_median0" ("nipype.interfaces.fsl.utils.ImageStats"), a CommandLine Interface with command:
fslstats /output/work_preproc/_subject_id_08/applywarp/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -k /output/work_preproc/_subject_id_08/mask_GM/c1sub-08_ses-test_T1w_flirt_thresh.nii -p 50
211018-10:06:54,414 nipype.workflow INFO:
[Node] Finished "_median0".
211018-10:06:54,419 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.median".
211018-10:06:54,750 nipype.workflow INFO:
[Job 37] Completed (work_preproc.susan.median).
211018-10:06:54,755 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.segment
* work_preproc.segment
211018-10:06:54,836 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.merge" in "/output/work_preproc/susan/_subject_id_08/merge".
211018-10:06:54,845 nipype.workflow INFO:
[Node] Running "merge" ("nipype.interfaces.utility.base.Merge")
211018-10:06:54,852 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.merge".
211018-10:06:56,753 nipype.workflow INFO:
[Job 38] Completed (work_preproc.susan.merge).
211018-10:06:56,761 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.segment
* work_preproc.segment
211018-10:06:56,985 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.multi_inputs" in "/output/work_preproc/susan/_subject_id_08/multi_inputs".
211018-10:06:57,44 nipype.workflow INFO:
[Node] Running "multi_inputs" ("nipype.interfaces.utility.wrappers.Function")
211018-10:06:57,58 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.multi_inputs".
211018-10:06:58,756 nipype.workflow INFO:
[Job 39] Completed (work_preproc.susan.multi_inputs).
211018-10:06:58,766 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.segment
* work_preproc.segment
211018-10:06:58,900 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.smooth" in "/output/work_preproc/susan/_subject_id_08/smooth".
211018-10:06:58,917 nipype.workflow INFO:
[Node] Setting-up "_smooth0" in "/output/work_preproc/susan/_subject_id_08/smooth/mapflow/_smooth0".
211018-10:06:58,932 nipype.workflow INFO:
[Node] Running "_smooth0" ("nipype.interfaces.fsl.preprocess.SUSAN"), a CommandLine Interface with command:
susan /output/work_preproc/_subject_id_08/applywarp/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii 1039.5000000000 1.6986436006 3 1 1 /output/work_preproc/susan/_subject_id_08/meanfunc2/mapflow/_meanfunc20/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz 1039.5000000000 /output/work_preproc/susan/_subject_id_08/smooth/mapflow/_smooth0/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz
211018-10:07:00,758 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 1 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
* work_preproc.segment
211018-10:07:20,33 nipype.workflow INFO:
[Node] Finished "work_preproc.segment".
211018-10:07:20,741 nipype.workflow INFO:
[Job 73] Completed (work_preproc.segment).
211018-10:07:20,745 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:07:20,828 nipype.workflow INFO:
[Node] Setting-up "work_preproc.resample" in "/output/work_preproc/_subject_id_04/resample".
211018-10:07:20,841 nipype.workflow INFO:
[Node] Running "resample" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_04/segment/c1sub-04_ses-test_T1w.nii -ref /output/work_preproc/_subject_id_04/segment/c1sub-04_ses-test_T1w.nii -out c1sub-04_ses-test_T1w_flirt.nii -omat c1sub-04_ses-test_T1w_flirt.mat -applyisoxfm 4.000000
211018-10:07:22,745 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.resample
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:07:25,77 nipype.workflow INFO:
[Node] Finished "work_preproc.resample".
211018-10:07:26,767 nipype.workflow INFO:
[Job 74] Completed (work_preproc.resample).
211018-10:07:26,789 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 3 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:07:26,962 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_GM" in "/output/work_preproc/_subject_id_04/mask_GM".
211018-10:07:27,99 nipype.workflow INFO:
[Node] Running "mask_GM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_04/resample/c1sub-04_ses-test_T1w_flirt.nii -thr 0.5000000000 -bin -dilF /output/work_preproc/_subject_id_04/mask_GM/c1sub-04_ses-test_T1w_flirt_thresh.nii
211018-10:07:28,815 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 2 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.mask_GM
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:07:29,118 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_GM".
211018-10:07:30,824 nipype.workflow INFO:
[Job 75] Completed (work_preproc.mask_GM).
211018-10:07:30,836 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:07:31,223 nipype.workflow INFO:
[Node] Setting-up "work_preproc.threshold_WM" in "/output/work_preproc/_subject_id_04/threshold_WM".
211018-10:07:31,273 nipype.workflow INFO:
[Node] Running "threshold_WM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_04/segment/c2sub-04_ses-test_T1w.nii -thr 0.5000000000 -bin /output/work_preproc/_subject_id_04/threshold_WM/c2sub-04_ses-test_T1w_thresh.nii
211018-10:07:32,821 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 1 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.threshold_WM
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:07:34,9 nipype.workflow INFO:
[Node] Finished "work_preproc.threshold_WM".
211018-10:07:34,818 nipype.workflow INFO:
[Job 76] Completed (work_preproc.threshold_WM).
211018-10:07:34,824 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:07:34,963 nipype.workflow INFO:
[Node] Setting-up "work_preproc.coreg" in "/output/work_preproc/_subject_id_04/coreg".
211018-10:07:34,985 nipype.workflow INFO:
[Node] Running "coreg" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_04/mcflirt/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg.nii.gz -ref /output/work_preproc/_subject_id_04/gunzip_anat/sub-04_ses-test_T1w.nii -out asub-04_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.nii -omat asub-04_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -cost bbr -dof 6 -schedule /usr/share/fsl/5.0/etc/flirtsch/bbr.sch -wmseg /output/work_preproc/_subject_id_04/threshold_WM/c2sub-04_ses-test_T1w_thresh.nii
211018-10:07:36,821 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 1 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.coreg
* work_preproc.susan.smooth
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:08:10,636 nipype.workflow INFO:
[Node] Finished "_smooth0".
211018-10:08:10,642 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.smooth".
211018-10:08:10,908 nipype.workflow INFO:
[Job 18] Completed (work_preproc.susan.smooth).
211018-10:08:10,914 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:08:11,53 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_func" in "/output/work_preproc/_subject_id_09/mask_func".
211018-10:08:11,62 nipype.workflow INFO:
[Node] Setting-up "_mask_func0" in "/output/work_preproc/_subject_id_09/mask_func/mapflow/_mask_func0".
211018-10:08:11,76 nipype.workflow INFO:
[Node] Running "_mask_func0" ("nipype.interfaces.fsl.maths.ApplyMask"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_09/smooth/mapflow/_smooth0/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz -mas /output/work_preproc/_subject_id_09/mask_GM/c1sub-09_ses-test_T1w_flirt_thresh.nii /output/work_preproc/_subject_id_09/mask_func/mapflow/_mask_func0/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth_masked.nii
211018-10:08:12,509 nipype.workflow INFO:
[Node] Finished "_mask_func0".
211018-10:08:12,516 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_func".
211018-10:08:12,911 nipype.workflow INFO:
[Job 19] Completed (work_preproc.mask_func).
211018-10:08:12,915 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:08:13,12 nipype.workflow INFO:
[Node] Setting-up "work_preproc.detrend" in "/output/work_preproc/_subject_id_09/detrend".
211018-10:08:13,27 nipype.workflow INFO:
[Node] Running "detrend" ("nipype.algorithms.confounds.TSNR")
211018-10:08:14,914 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 1 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.detrend
* work_preproc.coreg
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:08:19,32 nipype.workflow INFO:
[Node] Finished "_smooth0".
211018-10:08:19,37 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.smooth".
211018-10:08:20,920 nipype.workflow INFO:
[Job 40] Completed (work_preproc.susan.smooth).
211018-10:08:20,929 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.detrend
* work_preproc.coreg
* work_preproc.segment
211018-10:08:21,109 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_func" in "/output/work_preproc/_subject_id_08/mask_func".
211018-10:08:21,130 nipype.workflow INFO:
[Node] Setting-up "_mask_func0" in "/output/work_preproc/_subject_id_08/mask_func/mapflow/_mask_func0".
211018-10:08:21,143 nipype.workflow INFO:
[Node] Running "_mask_func0" ("nipype.interfaces.fsl.maths.ApplyMask"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_08/smooth/mapflow/_smooth0/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz -mas /output/work_preproc/_subject_id_08/mask_GM/c1sub-08_ses-test_T1w_flirt_thresh.nii /output/work_preproc/_subject_id_08/mask_func/mapflow/_mask_func0/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth_masked.nii
211018-10:08:22,650 nipype.workflow INFO:
[Node] Finished "_mask_func0".
211018-10:08:22,656 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_func".
211018-10:08:22,926 nipype.workflow INFO:
[Job 41] Completed (work_preproc.mask_func).
211018-10:08:22,936 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.detrend
* work_preproc.coreg
* work_preproc.segment
211018-10:08:23,197 nipype.workflow INFO:
[Node] Setting-up "work_preproc.detrend" in "/output/work_preproc/_subject_id_08/detrend".
211018-10:08:23,235 nipype.workflow INFO:
[Node] Running "detrend" ("nipype.algorithms.confounds.TSNR")
211018-10:08:24,928 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 1 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.detrend
* work_preproc.detrend
* work_preproc.coreg
* work_preproc.segment
211018-10:09:08,353 nipype.workflow INFO:
[Node] Finished "work_preproc.detrend".
211018-10:09:09,621 nipype.workflow INFO:
[Job 20] Completed (work_preproc.detrend).
211018-10:09:09,640 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 2 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.detrend
* work_preproc.coreg
* work_preproc.segment
211018-10:09:10,326 nipype.workflow INFO:
[Node] Setting-up "work_preproc.datasink" in "/output/work_preproc/_subject_id_09/datasink".
211018-10:09:10,374 nipype.workflow INFO:
[Node] Running "datasink" ("nipype.interfaces.io.DataSink")
211018-10:09:10,385 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_09/asub-09_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par -> /output/datasink_handson/preproc/sub-09.par
211018-10:09:10,389 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_09/art.asub-09_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt -> /output/datasink_handson/preproc/art.sub-09_outliers.txt
211018-10:09:10,392 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_09/plot.asub-09_ses-test_task-fingerfootlips_bold_roi_mcf.svg -> /output/datasink_handson/preproc/plot.sub-09.svg
211018-10:09:10,399 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_09/detrend.nii.gz -> /output/datasink_handson/preproc/sub-09_detrend.nii.gz
211018-10:09:10,408 nipype.workflow INFO:
[Node] Finished "work_preproc.datasink".
211018-10:09:11,621 nipype.workflow INFO:
[Job 21] Completed (work_preproc.datasink).
211018-10:09:11,627 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 1 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.detrend
* work_preproc.coreg
* work_preproc.segment
211018-10:09:11,745 nipype.workflow INFO:
[Node] Setting-up "work_preproc.selectfiles" in "/output/work_preproc/_subject_id_02/selectfiles".
211018-10:09:11,757 nipype.workflow INFO:
[Node] Running "selectfiles" ("nipype.interfaces.io.SelectFiles")
211018-10:09:11,774 nipype.workflow INFO:
[Node] Finished "work_preproc.selectfiles".
211018-10:09:11,885 nipype.workflow INFO:
[Node] Finished "work_preproc.detrend".
211018-10:09:13,618 nipype.workflow INFO:
[Job 42] Completed (work_preproc.detrend).
211018-10:09:13,621 nipype.workflow INFO:
[Job 110] Completed (work_preproc.selectfiles).
211018-10:09:13,627 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 3 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.segment
211018-10:09:13,721 nipype.workflow INFO:
[Node] Setting-up "work_preproc.datasink" in "/output/work_preproc/_subject_id_08/datasink".
211018-10:09:13,729 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_func" in "/output/work_preproc/_subject_id_02/gunzip_func".
211018-10:09:13,756 nipype.workflow INFO:
[Node] Running "gunzip_func" ("nipype.algorithms.misc.Gunzip")
211018-10:09:13,770 nipype.workflow INFO:
[Node] Running "datasink" ("nipype.interfaces.io.DataSink")
211018-10:09:13,776 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_08/asub-08_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par -> /output/datasink_handson/preproc/sub-08.par
211018-10:09:13,783 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_08/art.asub-08_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt -> /output/datasink_handson/preproc/art.sub-08_outliers.txt
211018-10:09:13,786 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_08/plot.asub-08_ses-test_task-fingerfootlips_bold_roi_mcf.svg -> /output/datasink_handson/preproc/plot.sub-08.svg
211018-10:09:13,791 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_08/detrend.nii.gz -> /output/datasink_handson/preproc/sub-08_detrend.nii.gz
211018-10:09:13,805 nipype.workflow INFO:
[Node] Finished "work_preproc.datasink".
211018-10:09:14,434 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_func".
211018-10:09:15,619 nipype.workflow INFO:
[Job 43] Completed (work_preproc.datasink).
211018-10:09:15,624 nipype.workflow INFO:
[Job 111] Completed (work_preproc.gunzip_func).
211018-10:09:15,629 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 2 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.segment
211018-10:09:15,709 nipype.workflow INFO:
[Node] Setting-up "work_preproc.gunzip_anat" in "/output/work_preproc/_subject_id_02/gunzip_anat".
211018-10:09:15,716 nipype.workflow INFO:
[Node] Setting-up "work_preproc.extract" in "/output/work_preproc/_subject_id_02/extract".
211018-10:09:15,723 nipype.workflow INFO:
[Node] Running "gunzip_anat" ("nipype.algorithms.misc.Gunzip")
211018-10:09:15,745 nipype.workflow INFO:
[Node] Running "extract" ("nipype.interfaces.fsl.utils.ExtractROI"), a CommandLine Interface with command:
fslroi /output/work_preproc/_subject_id_02/gunzip_func/sub-02_ses-test_task-fingerfootlips_bold.nii /output/work_preproc/_subject_id_02/extract/sub-02_ses-test_task-fingerfootlips_bold_roi.nii 4 -1
211018-10:09:16,73 nipype.workflow INFO:
[Node] Finished "work_preproc.gunzip_anat".
211018-10:09:17,9 nipype.workflow INFO:
[Node] Finished "work_preproc.extract".
211018-10:09:17,621 nipype.workflow INFO:
[Job 112] Completed (work_preproc.extract).
211018-10:09:17,624 nipype.workflow INFO:
[Job 116] Completed (work_preproc.gunzip_anat).
211018-10:09:17,630 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 2 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.segment
211018-10:09:17,729 nipype.workflow INFO:
[Node] Setting-up "work_preproc.segment" in "/output/work_preproc/_subject_id_02/segment".
211018-10:09:17,732 nipype.workflow INFO:
[Node] Setting-up "work_preproc.slicetime" in "/output/work_preproc/_subject_id_02/slicetime".
211018-10:09:17,745 nipype.workflow INFO:
[Node] Running "segment" ("nipype.interfaces.spm.preprocess.NewSegment")
211018-10:09:17,752 nipype.workflow INFO:
[Node] Running "slicetime" ("nipype.interfaces.spm.preprocess.SliceTiming")
211018-10:09:19,626 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 0 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.segment
* work_preproc.slicetime
* work_preproc.coreg
* work_preproc.segment
211018-10:09:38,932 nipype.workflow INFO:
[Node] Finished "work_preproc.slicetime".
211018-10:09:39,643 nipype.workflow INFO:
[Job 113] Completed (work_preproc.slicetime).
211018-10:09:39,647 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 1 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.segment
* work_preproc.coreg
* work_preproc.segment
211018-10:09:39,725 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mcflirt" in "/output/work_preproc/_subject_id_02/mcflirt".
211018-10:09:39,738 nipype.workflow INFO:
[Node] Running "mcflirt" ("nipype.interfaces.fsl.preprocess.MCFLIRT"), a CommandLine Interface with command:
mcflirt -in /output/work_preproc/_subject_id_02/slicetime/asub-02_ses-test_task-fingerfootlips_bold_roi.nii -meanvol -out /output/work_preproc/_subject_id_02/mcflirt/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -plots
211018-10:09:41,648 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 0 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.segment
* work_preproc.coreg
* work_preproc.segment
211018-10:10:41,354 nipype.workflow INFO:
[Node] Finished "work_preproc.coreg".
211018-10:10:41,724 nipype.workflow INFO:
[Job 77] Completed (work_preproc.coreg).
211018-10:10:41,729 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 1 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.mcflirt
* work_preproc.segment
* work_preproc.segment
211018-10:10:41,828 nipype.workflow INFO:
[Node] Setting-up "work_preproc.applywarp" in "/output/work_preproc/_subject_id_04/applywarp".
211018-10:10:41,853 nipype.workflow INFO:
[Node] Running "applywarp" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_04/mcflirt/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -ref /output/work_preproc/_subject_id_04/gunzip_anat/sub-04_ses-test_T1w.nii -out asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -omat asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.mat -applyisoxfm 4.000000 -init /output/work_preproc/_subject_id_04/coreg/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -interp spline
211018-10:10:43,728 nipype.workflow INFO:
[MultiProc] Running 4 tasks, and 0 jobs ready. Free memory (GB): 4.43/5.23, Free processors: 0/4.
Currently running:
* work_preproc.applywarp
* work_preproc.mcflirt
* work_preproc.segment
* work_preproc.segment
211018-10:10:53,670 nipype.workflow INFO:
[Node] Finished "work_preproc.mcflirt".
211018-10:10:53,736 nipype.workflow INFO:
[Job 114] Completed (work_preproc.mcflirt).
211018-10:10:53,740 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 1 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.applywarp
* work_preproc.segment
* work_preproc.segment
211018-10:10:53,877 nipype.workflow INFO:
[Node] Setting-up "work_preproc.art" in "/output/work_preproc/_subject_id_02/art".
211018-10:10:53,900 nipype.workflow INFO:
[Node] Running "art" ("nipype.algorithms.rapidart.ArtifactDetect")
211018-10:10:53,919 nipype.workflow INFO:
[Node] Finished "work_preproc.applywarp".
211018-10:10:54,986 nipype.workflow INFO:
[Node] Finished "work_preproc.art".
211018-10:10:55,737 nipype.workflow INFO:
[Job 78] Completed (work_preproc.applywarp).
211018-10:10:55,739 nipype.workflow INFO:
[Job 115] Completed (work_preproc.art).
211018-10:10:55,745 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 2 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:10:55,830 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.mask" in "/output/work_preproc/susan/_subject_id_04/mask".
211018-10:10:55,833 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.median" in "/output/work_preproc/susan/_subject_id_04/median".
211018-10:10:55,844 nipype.workflow INFO:
[Node] Setting-up "_mask0" in "/output/work_preproc/susan/_subject_id_04/mask/mapflow/_mask0".
211018-10:10:55,845 nipype.workflow INFO:
[Node] Setting-up "_median0" in "/output/work_preproc/susan/_subject_id_04/median/mapflow/_median0".
211018-10:10:55,854 nipype.workflow INFO:
[Node] Running "_median0" ("nipype.interfaces.fsl.utils.ImageStats"), a CommandLine Interface with command:
fslstats /output/work_preproc/_subject_id_04/applywarp/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -k /output/work_preproc/_subject_id_04/mask_GM/c1sub-04_ses-test_T1w_flirt_thresh.nii -p 50
211018-10:10:55,854 nipype.workflow INFO:
[Node] Running "_mask0" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_04/applywarp/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -mas /output/work_preproc/_subject_id_04/mask_GM/c1sub-04_ses-test_T1w_flirt_thresh.nii /output/work_preproc/susan/_subject_id_04/mask/mapflow/_mask0/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz
211018-10:10:57,248 nipype.workflow INFO:
[Node] Finished "_median0".
211018-10:10:57,253 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.median".
211018-10:10:57,740 nipype.workflow INFO:
[Job 81] Completed (work_preproc.susan.median).
211018-10:10:57,743 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 0 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.mask
* work_preproc.segment
* work_preproc.segment
211018-10:10:59,48 nipype.workflow INFO:
[Node] Finished "_mask0".
211018-10:10:59,54 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.mask".
211018-10:10:59,741 nipype.workflow INFO:
[Job 79] Completed (work_preproc.susan.mask).
211018-10:10:59,745 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:10:59,823 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.meanfunc2" in "/output/work_preproc/susan/_subject_id_04/meanfunc2".
211018-10:10:59,831 nipype.workflow INFO:
[Node] Setting-up "_meanfunc20" in "/output/work_preproc/susan/_subject_id_04/meanfunc2/mapflow/_meanfunc20".
211018-10:10:59,841 nipype.workflow INFO:
[Node] Running "_meanfunc20" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_04/mask/mapflow/_mask0/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz -Tmean /output/work_preproc/susan/_subject_id_04/meanfunc2/mapflow/_meanfunc20/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz
211018-10:11:01,49 nipype.workflow INFO:
[Node] Finished "_meanfunc20".
211018-10:11:01,52 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.meanfunc2".
211018-10:11:01,743 nipype.workflow INFO:
[Job 80] Completed (work_preproc.susan.meanfunc2).
211018-10:11:01,746 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:11:01,815 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.merge" in "/output/work_preproc/susan/_subject_id_04/merge".
211018-10:11:01,826 nipype.workflow INFO:
[Node] Running "merge" ("nipype.interfaces.utility.base.Merge")
211018-10:11:01,830 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.merge".
211018-10:11:03,745 nipype.workflow INFO:
[Job 82] Completed (work_preproc.susan.merge).
211018-10:11:03,748 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:11:03,819 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.multi_inputs" in "/output/work_preproc/susan/_subject_id_04/multi_inputs".
211018-10:11:03,832 nipype.workflow INFO:
[Node] Running "multi_inputs" ("nipype.interfaces.utility.wrappers.Function")
211018-10:11:03,839 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.multi_inputs".
211018-10:11:05,748 nipype.workflow INFO:
[Job 83] Completed (work_preproc.susan.multi_inputs).
211018-10:11:05,752 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.segment
* work_preproc.segment
211018-10:11:05,843 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.smooth" in "/output/work_preproc/susan/_subject_id_04/smooth".
211018-10:11:05,851 nipype.workflow INFO:
[Node] Setting-up "_smooth0" in "/output/work_preproc/susan/_subject_id_04/smooth/mapflow/_smooth0".
211018-10:11:05,858 nipype.workflow INFO:
[Node] Running "_smooth0" ("nipype.interfaces.fsl.preprocess.SUSAN"), a CommandLine Interface with command:
susan /output/work_preproc/_subject_id_04/applywarp/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii 1022.2500000000 1.6986436006 3 1 1 /output/work_preproc/susan/_subject_id_04/meanfunc2/mapflow/_meanfunc20/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz 1022.2500000000 /output/work_preproc/susan/_subject_id_04/smooth/mapflow/_smooth0/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz
211018-10:11:07,751 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 0 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.segment
* work_preproc.segment
211018-10:11:30,935 nipype.workflow INFO:
[Node] Finished "work_preproc.segment".
211018-10:11:31,773 nipype.workflow INFO:
[Job 95] Completed (work_preproc.segment).
211018-10:11:31,776 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 2 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:11:31,856 nipype.workflow INFO:
[Node] Setting-up "work_preproc.threshold_WM" in "/output/work_preproc/_subject_id_03/threshold_WM".
211018-10:11:31,855 nipype.workflow INFO:
[Node] Setting-up "work_preproc.resample" in "/output/work_preproc/_subject_id_03/resample".
211018-10:11:31,868 nipype.workflow INFO:
[Node] Running "resample" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_03/segment/c1sub-03_ses-test_T1w.nii -ref /output/work_preproc/_subject_id_03/segment/c1sub-03_ses-test_T1w.nii -out c1sub-03_ses-test_T1w_flirt.nii -omat c1sub-03_ses-test_T1w_flirt.mat -applyisoxfm 4.000000
211018-10:11:31,870 nipype.workflow INFO:
[Node] Running "threshold_WM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_03/segment/c2sub-03_ses-test_T1w.nii -thr 0.5000000000 -bin /output/work_preproc/_subject_id_03/threshold_WM/c2sub-03_ses-test_T1w_thresh.nii
211018-10:11:32,483 nipype.workflow INFO:
[Node] Finished "work_preproc.threshold_WM".
211018-10:11:33,776 nipype.workflow INFO:
[Job 98] Completed (work_preproc.threshold_WM).
211018-10:11:33,780 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 1 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.resample
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:11:33,875 nipype.workflow INFO:
[Node] Setting-up "work_preproc.coreg" in "/output/work_preproc/_subject_id_03/coreg".
211018-10:11:33,889 nipype.workflow INFO:
[Node] Running "coreg" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_03/mcflirt/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg.nii.gz -ref /output/work_preproc/_subject_id_03/gunzip_anat/sub-03_ses-test_T1w.nii -out asub-03_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.nii -omat asub-03_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -cost bbr -dof 6 -schedule /usr/share/fsl/5.0/etc/flirtsch/bbr.sch -wmseg /output/work_preproc/_subject_id_03/threshold_WM/c2sub-03_ses-test_T1w_thresh.nii
211018-10:11:35,706 nipype.workflow INFO:
[Node] Finished "work_preproc.resample".
211018-10:11:35,781 nipype.workflow INFO:
[Job 96] Completed (work_preproc.resample).
211018-10:11:35,785 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 1 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:11:35,874 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_GM" in "/output/work_preproc/_subject_id_03/mask_GM".
211018-10:11:35,888 nipype.workflow INFO:
[Node] Running "mask_GM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_03/resample/c1sub-03_ses-test_T1w_flirt.nii -thr 0.5000000000 -bin -dilF /output/work_preproc/_subject_id_03/mask_GM/c1sub-03_ses-test_T1w_flirt_thresh.nii
211018-10:11:36,413 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_GM".
211018-10:11:37,782 nipype.workflow INFO:
[Job 97] Completed (work_preproc.mask_GM).
211018-10:11:37,786 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 0 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.coreg
* work_preproc.susan.smooth
* work_preproc.segment
211018-10:11:48,566 nipype.workflow INFO:
[Node] Finished "_smooth0".
211018-10:11:48,569 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.smooth".
211018-10:11:49,797 nipype.workflow INFO:
[Job 84] Completed (work_preproc.susan.smooth).
211018-10:11:49,800 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.segment
211018-10:11:49,874 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_func" in "/output/work_preproc/_subject_id_04/mask_func".
211018-10:11:49,882 nipype.workflow INFO:
[Node] Setting-up "_mask_func0" in "/output/work_preproc/_subject_id_04/mask_func/mapflow/_mask_func0".
211018-10:11:49,889 nipype.workflow INFO:
[Node] Running "_mask_func0" ("nipype.interfaces.fsl.maths.ApplyMask"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_04/smooth/mapflow/_smooth0/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz -mas /output/work_preproc/_subject_id_04/mask_GM/c1sub-04_ses-test_T1w_flirt_thresh.nii /output/work_preproc/_subject_id_04/mask_func/mapflow/_mask_func0/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth_masked.nii
211018-10:11:50,921 nipype.workflow INFO:
[Node] Finished "_mask_func0".
211018-10:11:50,925 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_func".
211018-10:11:51,799 nipype.workflow INFO:
[Job 85] Completed (work_preproc.mask_func).
211018-10:11:51,802 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.segment
211018-10:11:51,862 nipype.workflow INFO:
[Node] Setting-up "work_preproc.detrend" in "/output/work_preproc/_subject_id_04/detrend".
211018-10:11:51,870 nipype.workflow INFO:
[Node] Running "detrend" ("nipype.algorithms.confounds.TSNR")
211018-10:11:53,802 nipype.workflow INFO:
[MultiProc] Running 3 tasks, and 0 jobs ready. Free memory (GB): 4.63/5.23, Free processors: 1/4.
Currently running:
* work_preproc.detrend
* work_preproc.coreg
* work_preproc.segment
211018-10:12:02,466 nipype.workflow INFO:
[Node] Finished "work_preproc.detrend".
211018-10:12:03,811 nipype.workflow INFO:
[Job 86] Completed (work_preproc.detrend).
211018-10:12:03,815 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.segment
211018-10:12:03,901 nipype.workflow INFO:
[Node] Setting-up "work_preproc.datasink" in "/output/work_preproc/_subject_id_04/datasink".
211018-10:12:03,925 nipype.workflow INFO:
[Node] Running "datasink" ("nipype.interfaces.io.DataSink")
211018-10:12:03,928 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_04/asub-04_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par -> /output/datasink_handson/preproc/sub-04.par
211018-10:12:03,932 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_04/art.asub-04_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt -> /output/datasink_handson/preproc/art.sub-04_outliers.txt
211018-10:12:03,934 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_04/plot.asub-04_ses-test_task-fingerfootlips_bold_roi_mcf.svg -> /output/datasink_handson/preproc/plot.sub-04.svg
211018-10:12:03,937 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_04/detrend.nii.gz -> /output/datasink_handson/preproc/sub-04_detrend.nii.gz
211018-10:12:03,946 nipype.workflow INFO:
[Node] Finished "work_preproc.datasink".
211018-10:12:05,813 nipype.workflow INFO:
[Job 87] Completed (work_preproc.datasink).
211018-10:12:05,816 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.segment
211018-10:12:36,361 nipype.workflow INFO:
[Node] Finished "work_preproc.segment".
211018-10:12:37,847 nipype.workflow INFO:
[Job 117] Completed (work_preproc.segment).
211018-10:12:37,850 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 2 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:12:37,919 nipype.workflow INFO:
[Node] Setting-up "work_preproc.resample" in "/output/work_preproc/_subject_id_02/resample".
211018-10:12:37,921 nipype.workflow INFO:
[Node] Setting-up "work_preproc.threshold_WM" in "/output/work_preproc/_subject_id_02/threshold_WM".
211018-10:12:37,933 nipype.workflow INFO:
[Node] Running "threshold_WM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_02/segment/c2sub-02_ses-test_T1w.nii -thr 0.5000000000 -bin /output/work_preproc/_subject_id_02/threshold_WM/c2sub-02_ses-test_T1w_thresh.nii
211018-10:12:37,933 nipype.workflow INFO:
[Node] Running "resample" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_02/segment/c1sub-02_ses-test_T1w.nii -ref /output/work_preproc/_subject_id_02/segment/c1sub-02_ses-test_T1w.nii -out c1sub-02_ses-test_T1w_flirt.nii -omat c1sub-02_ses-test_T1w_flirt.mat -applyisoxfm 4.000000
211018-10:12:38,478 nipype.workflow INFO:
[Node] Finished "work_preproc.threshold_WM".
211018-10:12:39,848 nipype.workflow INFO:
[Job 120] Completed (work_preproc.threshold_WM).
211018-10:12:39,851 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.resample
* work_preproc.coreg
211018-10:12:39,923 nipype.workflow INFO:
[Node] Setting-up "work_preproc.coreg" in "/output/work_preproc/_subject_id_02/coreg".
211018-10:12:39,960 nipype.workflow INFO:
[Node] Running "coreg" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_02/mcflirt/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg.nii.gz -ref /output/work_preproc/_subject_id_02/gunzip_anat/sub-02_ses-test_T1w.nii -out asub-02_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.nii -omat asub-02_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -cost bbr -dof 6 -schedule /usr/share/fsl/5.0/etc/flirtsch/bbr.sch -wmseg /output/work_preproc/_subject_id_02/threshold_WM/c2sub-02_ses-test_T1w_thresh.nii
211018-10:12:41,287 nipype.workflow INFO:
[Node] Finished "work_preproc.resample".
211018-10:12:41,851 nipype.workflow INFO:
[Job 118] Completed (work_preproc.resample).
211018-10:12:41,854 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 1 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.coreg
211018-10:12:41,927 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_GM" in "/output/work_preproc/_subject_id_02/mask_GM".
211018-10:12:41,937 nipype.workflow INFO:
[Node] Running "mask_GM" ("nipype.interfaces.fsl.maths.Threshold"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_02/resample/c1sub-02_ses-test_T1w_flirt.nii -thr 0.5000000000 -bin -dilF /output/work_preproc/_subject_id_02/mask_GM/c1sub-02_ses-test_T1w_flirt_thresh.nii
211018-10:12:42,399 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_GM".
211018-10:12:43,854 nipype.workflow INFO:
[Job 119] Completed (work_preproc.mask_GM).
211018-10:12:43,858 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.coreg
* work_preproc.coreg
211018-10:13:11,934 nipype.workflow INFO:
[Node] Finished "work_preproc.coreg".
211018-10:13:13,887 nipype.workflow INFO:
[Job 99] Completed (work_preproc.coreg).
211018-10:13:13,890 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:13:13,957 nipype.workflow INFO:
[Node] Setting-up "work_preproc.applywarp" in "/output/work_preproc/_subject_id_03/applywarp".
211018-10:13:13,966 nipype.workflow INFO:
[Node] Running "applywarp" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_03/mcflirt/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -ref /output/work_preproc/_subject_id_03/gunzip_anat/sub-03_ses-test_T1w.nii -out asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -omat asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.mat -applyisoxfm 4.000000 -init /output/work_preproc/_subject_id_03/coreg/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -interp spline
211018-10:13:15,890 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.applywarp
* work_preproc.coreg
211018-10:13:22,786 nipype.workflow INFO:
[Node] Finished "work_preproc.applywarp".
211018-10:13:23,898 nipype.workflow INFO:
[Job 100] Completed (work_preproc.applywarp).
211018-10:13:23,901 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 2 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:13:23,975 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.mask" in "/output/work_preproc/susan/_subject_id_03/mask".
211018-10:13:23,980 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.median" in "/output/work_preproc/susan/_subject_id_03/median".
211018-10:13:23,982 nipype.workflow INFO:
[Node] Setting-up "_mask0" in "/output/work_preproc/susan/_subject_id_03/mask/mapflow/_mask0".
211018-10:13:23,987 nipype.workflow INFO:
[Node] Running "_mask0" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_03/applywarp/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -mas /output/work_preproc/_subject_id_03/mask_GM/c1sub-03_ses-test_T1w_flirt_thresh.nii /output/work_preproc/susan/_subject_id_03/mask/mapflow/_mask0/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz
211018-10:13:23,992 nipype.workflow INFO:
[Node] Setting-up "_median0" in "/output/work_preproc/susan/_subject_id_03/median/mapflow/_median0".
211018-10:13:23,997 nipype.workflow INFO:
[Node] Running "_median0" ("nipype.interfaces.fsl.utils.ImageStats"), a CommandLine Interface with command:
fslstats /output/work_preproc/_subject_id_03/applywarp/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -k /output/work_preproc/_subject_id_03/mask_GM/c1sub-03_ses-test_T1w_flirt_thresh.nii -p 50
211018-10:13:25,261 nipype.workflow INFO:
[Node] Finished "_median0".
211018-10:13:25,266 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.median".
211018-10:13:25,900 nipype.workflow INFO:
[Job 103] Completed (work_preproc.susan.median).
211018-10:13:25,905 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.susan.mask
* work_preproc.coreg
211018-10:13:26,820 nipype.workflow INFO:
[Node] Finished "_mask0".
211018-10:13:26,823 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.mask".
211018-10:13:27,904 nipype.workflow INFO:
[Job 101] Completed (work_preproc.susan.mask).
211018-10:13:27,909 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:13:28,12 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.meanfunc2" in "/output/work_preproc/susan/_subject_id_03/meanfunc2".
211018-10:13:28,21 nipype.workflow INFO:
[Node] Setting-up "_meanfunc20" in "/output/work_preproc/susan/_subject_id_03/meanfunc2/mapflow/_meanfunc20".
211018-10:13:28,28 nipype.workflow INFO:
[Node] Running "_meanfunc20" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_03/mask/mapflow/_mask0/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz -Tmean /output/work_preproc/susan/_subject_id_03/meanfunc2/mapflow/_meanfunc20/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz
211018-10:13:29,790 nipype.workflow INFO:
[Node] Finished "_meanfunc20".
211018-10:13:29,795 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.meanfunc2".
211018-10:13:29,906 nipype.workflow INFO:
[Job 102] Completed (work_preproc.susan.meanfunc2).
211018-10:13:29,911 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:13:30,23 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.merge" in "/output/work_preproc/susan/_subject_id_03/merge".
211018-10:13:30,31 nipype.workflow INFO:
[Node] Running "merge" ("nipype.interfaces.utility.base.Merge")
211018-10:13:30,38 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.merge".
211018-10:13:31,907 nipype.workflow INFO:
[Job 104] Completed (work_preproc.susan.merge).
211018-10:13:31,910 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:13:31,977 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.multi_inputs" in "/output/work_preproc/susan/_subject_id_03/multi_inputs".
211018-10:13:31,988 nipype.workflow INFO:
[Node] Running "multi_inputs" ("nipype.interfaces.utility.wrappers.Function")
211018-10:13:31,992 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.multi_inputs".
211018-10:13:33,910 nipype.workflow INFO:
[Job 105] Completed (work_preproc.susan.multi_inputs).
211018-10:13:33,912 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:13:33,983 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.smooth" in "/output/work_preproc/susan/_subject_id_03/smooth".
211018-10:13:33,990 nipype.workflow INFO:
[Node] Setting-up "_smooth0" in "/output/work_preproc/susan/_subject_id_03/smooth/mapflow/_smooth0".
211018-10:13:33,995 nipype.workflow INFO:
[Node] Running "_smooth0" ("nipype.interfaces.fsl.preprocess.SUSAN"), a CommandLine Interface with command:
susan /output/work_preproc/_subject_id_03/applywarp/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii 981.7500000000 1.6986436006 3 1 1 /output/work_preproc/susan/_subject_id_03/meanfunc2/mapflow/_meanfunc20/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz 981.7500000000 /output/work_preproc/susan/_subject_id_03/smooth/mapflow/_smooth0/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz
211018-10:13:35,912 nipype.workflow INFO:
[MultiProc] Running 2 tasks, and 0 jobs ready. Free memory (GB): 4.83/5.23, Free processors: 2/4.
Currently running:
* work_preproc.susan.smooth
* work_preproc.coreg
211018-10:14:10,961 nipype.workflow INFO:
[Node] Finished "_smooth0".
211018-10:14:10,964 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.smooth".
211018-10:14:11,953 nipype.workflow INFO:
[Job 106] Completed (work_preproc.susan.smooth).
211018-10:14:11,956 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:14:12,29 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_func" in "/output/work_preproc/_subject_id_03/mask_func".
211018-10:14:12,35 nipype.workflow INFO:
[Node] Setting-up "_mask_func0" in "/output/work_preproc/_subject_id_03/mask_func/mapflow/_mask_func0".
211018-10:14:12,40 nipype.workflow INFO:
[Node] Running "_mask_func0" ("nipype.interfaces.fsl.maths.ApplyMask"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_03/smooth/mapflow/_smooth0/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz -mas /output/work_preproc/_subject_id_03/mask_GM/c1sub-03_ses-test_T1w_flirt_thresh.nii /output/work_preproc/_subject_id_03/mask_func/mapflow/_mask_func0/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth_masked.nii
211018-10:14:13,28 nipype.workflow INFO:
[Node] Finished "_mask_func0".
211018-10:14:13,31 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_func".
211018-10:14:13,956 nipype.workflow INFO:
[Job 107] Completed (work_preproc.mask_func).
211018-10:14:13,959 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.coreg
211018-10:14:14,19 nipype.workflow INFO:
[Node] Setting-up "work_preproc.detrend" in "/output/work_preproc/_subject_id_03/detrend".
211018-10:14:14,24 nipype.workflow INFO:
[Node] Running "detrend" ("nipype.algorithms.confounds.TSNR")
211018-10:14:14,901 nipype.workflow INFO:
[Node] Finished "work_preproc.coreg".
211018-10:14:15,958 nipype.workflow INFO:
[Job 121] Completed (work_preproc.coreg).
211018-10:14:15,962 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.detrend
211018-10:14:16,48 nipype.workflow INFO:
[Node] Setting-up "work_preproc.applywarp" in "/output/work_preproc/_subject_id_02/applywarp".
211018-10:14:16,62 nipype.workflow INFO:
[Node] Running "applywarp" ("nipype.interfaces.fsl.preprocess.FLIRT"), a CommandLine Interface with command:
flirt -in /output/work_preproc/_subject_id_02/mcflirt/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz -ref /output/work_preproc/_subject_id_02/gunzip_anat/sub-02_ses-test_T1w.nii -out asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -omat asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.mat -applyisoxfm 4.000000 -init /output/work_preproc/_subject_id_02/coreg/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz_mean_reg_flirt.mat -interp spline
211018-10:14:17,214 nipype.workflow INFO:
[Node] Finished "work_preproc.detrend".
211018-10:14:17,960 nipype.workflow INFO:
[Job 108] Completed (work_preproc.detrend).
211018-10:14:17,963 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 1 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.applywarp
211018-10:14:18,28 nipype.workflow INFO:
[Node] Setting-up "work_preproc.datasink" in "/output/work_preproc/_subject_id_03/datasink".
211018-10:14:18,45 nipype.workflow INFO:
[Node] Running "datasink" ("nipype.interfaces.io.DataSink")
211018-10:14:18,47 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_03/asub-03_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par -> /output/datasink_handson/preproc/sub-03.par
211018-10:14:18,49 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_03/art.asub-03_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt -> /output/datasink_handson/preproc/art.sub-03_outliers.txt
211018-10:14:18,51 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_03/plot.asub-03_ses-test_task-fingerfootlips_bold_roi_mcf.svg -> /output/datasink_handson/preproc/plot.sub-03.svg
211018-10:14:18,54 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_03/detrend.nii.gz -> /output/datasink_handson/preproc/sub-03_detrend.nii.gz
211018-10:14:18,59 nipype.workflow INFO:
[Node] Finished "work_preproc.datasink".
211018-10:14:19,962 nipype.workflow INFO:
[Job 109] Completed (work_preproc.datasink).
211018-10:14:19,966 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.applywarp
211018-10:14:24,869 nipype.workflow INFO:
[Node] Finished "work_preproc.applywarp".
211018-10:14:25,978 nipype.workflow INFO:
[Job 122] Completed (work_preproc.applywarp).
211018-10:14:25,984 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 2 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-10:14:26,76 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.mask" in "/output/work_preproc/susan/_subject_id_02/mask".
211018-10:14:26,78 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.median" in "/output/work_preproc/susan/_subject_id_02/median".
211018-10:14:26,87 nipype.workflow INFO:
[Node] Setting-up "_mask0" in "/output/work_preproc/susan/_subject_id_02/mask/mapflow/_mask0".
211018-10:14:26,87 nipype.workflow INFO:
[Node] Setting-up "_median0" in "/output/work_preproc/susan/_subject_id_02/median/mapflow/_median0".
211018-10:14:26,94 nipype.workflow INFO:
[Node] Running "_median0" ("nipype.interfaces.fsl.utils.ImageStats"), a CommandLine Interface with command:
fslstats /output/work_preproc/_subject_id_02/applywarp/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -k /output/work_preproc/_subject_id_02/mask_GM/c1sub-02_ses-test_T1w_flirt_thresh.nii -p 50
211018-10:14:26,95 nipype.workflow INFO:
[Node] Running "_mask0" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/_subject_id_02/applywarp/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii -mas /output/work_preproc/_subject_id_02/mask_GM/c1sub-02_ses-test_T1w_flirt_thresh.nii /output/work_preproc/susan/_subject_id_02/mask/mapflow/_mask0/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz
211018-10:14:27,397 nipype.workflow INFO:
[Node] Finished "_median0".
211018-10:14:27,400 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.median".
211018-10:14:27,978 nipype.workflow INFO:
[Job 125] Completed (work_preproc.susan.median).
211018-10:14:27,982 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.susan.mask
211018-10:14:28,773 nipype.workflow INFO:
[Node] Finished "_mask0".
211018-10:14:28,777 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.mask".
211018-10:14:29,983 nipype.workflow INFO:
[Job 123] Completed (work_preproc.susan.mask).
211018-10:14:29,986 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-10:14:30,68 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.meanfunc2" in "/output/work_preproc/susan/_subject_id_02/meanfunc2".
211018-10:14:30,76 nipype.workflow INFO:
[Node] Setting-up "_meanfunc20" in "/output/work_preproc/susan/_subject_id_02/meanfunc2/mapflow/_meanfunc20".
211018-10:14:30,83 nipype.workflow INFO:
[Node] Running "_meanfunc20" ("nipype.interfaces.fsl.utils.ImageMaths"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_02/mask/mapflow/_mask0/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask.nii.gz -Tmean /output/work_preproc/susan/_subject_id_02/meanfunc2/mapflow/_meanfunc20/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz
211018-10:14:31,204 nipype.workflow INFO:
[Node] Finished "_meanfunc20".
211018-10:14:31,208 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.meanfunc2".
211018-10:14:31,991 nipype.workflow INFO:
[Job 124] Completed (work_preproc.susan.meanfunc2).
211018-10:14:31,997 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-10:14:32,76 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.merge" in "/output/work_preproc/susan/_subject_id_02/merge".
211018-10:14:32,85 nipype.workflow INFO:
[Node] Running "merge" ("nipype.interfaces.utility.base.Merge")
211018-10:14:32,91 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.merge".
211018-10:14:34,0 nipype.workflow INFO:
[Job 126] Completed (work_preproc.susan.merge).
211018-10:14:34,5 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-10:14:34,84 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.multi_inputs" in "/output/work_preproc/susan/_subject_id_02/multi_inputs".
211018-10:14:34,95 nipype.workflow INFO:
[Node] Running "multi_inputs" ("nipype.interfaces.utility.wrappers.Function")
211018-10:14:34,103 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.multi_inputs".
211018-10:14:36,5 nipype.workflow INFO:
[Job 127] Completed (work_preproc.susan.multi_inputs).
211018-10:14:36,10 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-10:14:36,94 nipype.workflow INFO:
[Node] Setting-up "work_preproc.susan.smooth" in "/output/work_preproc/susan/_subject_id_02/smooth".
211018-10:14:36,104 nipype.workflow INFO:
[Node] Setting-up "_smooth0" in "/output/work_preproc/susan/_subject_id_02/smooth/mapflow/_smooth0".
211018-10:14:36,111 nipype.workflow INFO:
[Node] Running "_smooth0" ("nipype.interfaces.fsl.preprocess.SUSAN"), a CommandLine Interface with command:
susan /output/work_preproc/_subject_id_02/applywarp/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt.nii 1018.5000000000 1.6986436006 3 1 1 /output/work_preproc/susan/_subject_id_02/meanfunc2/mapflow/_meanfunc20/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_mask_mean.nii.gz 1018.5000000000 /output/work_preproc/susan/_subject_id_02/smooth/mapflow/_smooth0/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz
211018-10:14:38,5 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.susan.smooth
211018-10:15:12,487 nipype.workflow INFO:
[Node] Finished "_smooth0".
211018-10:15:12,492 nipype.workflow INFO:
[Node] Finished "work_preproc.susan.smooth".
211018-10:15:14,46 nipype.workflow INFO:
[Job 128] Completed (work_preproc.susan.smooth).
211018-10:15:14,52 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-10:15:14,140 nipype.workflow INFO:
[Node] Setting-up "work_preproc.mask_func" in "/output/work_preproc/_subject_id_02/mask_func".
211018-10:15:14,147 nipype.workflow INFO:
[Node] Setting-up "_mask_func0" in "/output/work_preproc/_subject_id_02/mask_func/mapflow/_mask_func0".
211018-10:15:14,154 nipype.workflow INFO:
[Node] Running "_mask_func0" ("nipype.interfaces.fsl.maths.ApplyMask"), a CommandLine Interface with command:
fslmaths /output/work_preproc/susan/_subject_id_02/smooth/mapflow/_smooth0/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth.nii.gz -mas /output/work_preproc/_subject_id_02/mask_GM/c1sub-02_ses-test_T1w_flirt_thresh.nii /output/work_preproc/_subject_id_02/mask_func/mapflow/_mask_func0/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_flirt_smooth_masked.nii
211018-10:15:15,159 nipype.workflow INFO:
[Node] Finished "_mask_func0".
211018-10:15:15,163 nipype.workflow INFO:
[Node] Finished "work_preproc.mask_func".
211018-10:15:16,54 nipype.workflow INFO:
[Job 129] Completed (work_preproc.mask_func).
211018-10:15:16,59 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-10:15:16,136 nipype.workflow INFO:
[Node] Setting-up "work_preproc.detrend" in "/output/work_preproc/_subject_id_02/detrend".
211018-10:15:16,144 nipype.workflow INFO:
[Node] Running "detrend" ("nipype.algorithms.confounds.TSNR")
211018-10:15:18,55 nipype.workflow INFO:
[MultiProc] Running 1 tasks, and 0 jobs ready. Free memory (GB): 5.03/5.23, Free processors: 3/4.
Currently running:
* work_preproc.detrend
211018-10:15:20,243 nipype.workflow INFO:
[Node] Finished "work_preproc.detrend".
211018-10:15:22,63 nipype.workflow INFO:
[Job 130] Completed (work_preproc.detrend).
211018-10:15:22,68 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 1 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
211018-10:15:22,148 nipype.workflow INFO:
[Node] Setting-up "work_preproc.datasink" in "/output/work_preproc/_subject_id_02/datasink".
211018-10:15:22,167 nipype.workflow INFO:
[Node] Running "datasink" ("nipype.interfaces.io.DataSink")
211018-10:15:22,170 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_02/asub-02_ses-test_task-fingerfootlips_bold_roi_mcf.nii.gz.par -> /output/datasink_handson/preproc/sub-02.par
211018-10:15:22,173 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_02/art.asub-02_ses-test_task-fingerfootlips_bold_roi_mcf_outliers.txt -> /output/datasink_handson/preproc/art.sub-02_outliers.txt
211018-10:15:22,175 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_02/plot.asub-02_ses-test_task-fingerfootlips_bold_roi_mcf.svg -> /output/datasink_handson/preproc/plot.sub-02.svg
211018-10:15:22,177 nipype.interface INFO:
sub: /output/datasink_handson/preproc/_subject_id_02/detrend.nii.gz -> /output/datasink_handson/preproc/sub-02_detrend.nii.gz
211018-10:15:22,185 nipype.workflow INFO:
[Node] Finished "work_preproc.datasink".
211018-10:15:24,71 nipype.workflow INFO:
[Job 131] Completed (work_preproc.datasink).
211018-10:15:24,76 nipype.workflow INFO:
[MultiProc] Running 0 tasks, and 0 jobs ready. Free memory (GB): 5.23/5.23, Free processors: 4/4.
<networkx.classes.digraph.DiGraph at 0x7f47591f5710>
Now we’re ready for the next section Hands-on 2: How to create a fMRI analysis workflow!